- Verify Certificate
- Classroom Training
- Upcoming Batches
- Online Courses
- Website Design
- Understanding Embedded Assignments, Chained Assignments, and Compound Assignments in C++

Embedded Assignments
Chained assignment, compound assignment.
- C++ Programming Language: Features, History, and Applications
- First Program in C++: Displaying a Welcome Message
- Tokens in C++: Identifiers, Keywords, Constants, Strings, and Operators
- Data Types in C++: Built-in, User-Defined, and Derived Data Types with Size and Range
- Identifiers in C++: Naming Variables, Constants, Functions, and More
- User-Defined Data Types in C++: Structures, Unions, Classes, and Enumerations
- Understanding Storage Classes in C++: Automatic, External, Static, and Register
- Exploring Derived Data Types in C++: Functions, Arrays, and Pointers
- Symbolic Constants in C++: const Keyword and enum Keyword
- Variable Declaration, Dynamic Initialization, and Reference Variables in C++
- Scope Resolution Operator in C++: Accessing Local and Global Variables
- Guide to Arithmetic, Relational, and Logical Operators in C++
- Guide to Conditional Statements and Switch Statements in C++
- Complete Guide to Loops in C++: For, While, Do-While, and Nested Loops
- Functions in C++: Definition, Parameters, Call by Reference, Inline Functions, and Default Arguments
- Classes and Inheritance in C++: Syntax, Objects, Member Functions, Constructors, Destructors, Friend Functions, and Inheritance Types

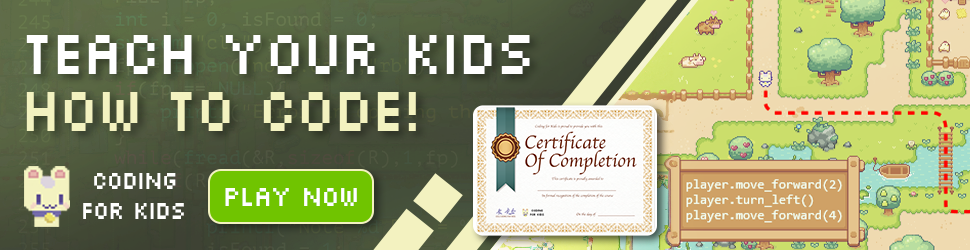
Linked lists
Introduction.
Linked lists are the best and simplest example of a dynamic data structure that uses pointers for its implementation. However, understanding pointers is crucial to understanding how linked lists work, so if you've skipped the pointers tutorial, you should go back and redo it. You must also be familiar with dynamic memory allocation and structures.
Essentially, linked lists function as an array that can grow and shrink as needed, from any point in the array.
Linked lists have a few advantages over arrays:
- Items can be added or removed from the middle of the list
- There is no need to define an initial size
However, linked lists also have a few disadvantages:
- There is no "random" access - it is impossible to reach the nth item in the array without first iterating over all items up until that item. This means we have to start from the beginning of the list and count how many times we advance in the list until we get to the desired item.
- Dynamic memory allocation and pointers are required, which complicates the code and increases the risk of memory leaks and segment faults.
- Linked lists have a much larger overhead over arrays, since linked list items are dynamically allocated (which is less efficient in memory usage) and each item in the list also must store an additional pointer.
What is a linked list?
A linked list is a set of dynamically allocated nodes, arranged in such a way that each node contains one value and one pointer. The pointer always points to the next member of the list. If the pointer is NULL, then it is the last node in the list.
A linked list is held using a local pointer variable which points to the first item of the list. If that pointer is also NULL, then the list is considered to be empty.
Let's define a linked list node:
Notice that we are defining the struct in a recursive manner, which is possible in C. Let's name our node type node_t .
Now we can use the nodes. Let's create a local variable which points to the first item of the list (called head ).
We've just created the first variable in the list. We must set the value, and the next item to be empty, if we want to finish populating the list. Notice that we should always check if malloc returned a NULL value or not.
To add a variable to the end of the list, we can just continue advancing to the next pointer:
This can go on and on, but what we should actually do is advance to the last item of the list, until the next variable will be NULL .
Iterating over a list
Let's build a function that prints out all the items of a list. To do this, we need to use a current pointer that will keep track of the node we are currently printing. After printing the value of the node, we set the current pointer to the next node, and print again, until we've reached the end of the list (the next node is NULL).
Adding an item to the end of the list
To iterate over all the members of the linked list, we use a pointer called current . We set it to start from the head and then in each step, we advance the pointer to the next item in the list, until we reach the last item.
The best use cases for linked lists are stacks and queues, which we will now implement:
Adding an item to the beginning of the list (pushing to the list)
To add to the beginning of the list, we will need to do the following:
- Create a new item and set its value
- Link the new item to point to the head of the list
- Set the head of the list to be our new item
This will effectively create a new head to the list with a new value, and keep the rest of the list linked to it.
Since we use a function to do this operation, we want to be able to modify the head variable. To do this, we must pass a pointer to the pointer variable (a double pointer) so we will be able to modify the pointer itself.
Removing the first item (popping from the list)
To pop a variable, we will need to reverse this action:
- Take the next item that the head points to and save it
- Free the head item
- Set the head to be the next item that we've stored on the side
Here is the code:
Removing the last item of the list
Removing the last item from a list is very similar to adding it to the end of the list, but with one big exception - since we have to change one item before the last item, we actually have to look two items ahead and see if the next item is the last one in the list:
Removing a specific item
To remove a specific item from the list, either by its index from the beginning of the list or by its value, we will need to go over all the items, continuously looking ahead to find out if we've reached the node before the item we wish to remove. This is because we need to change the location to where the previous node points to as well.
Here is the algorithm:
- Iterate to the node before the node we wish to delete
- Save the node we wish to delete in a temporary pointer
- Set the previous node's next pointer to point to the node after the node we wish to delete
- Delete the node using the temporary pointer
There are a few edge cases we need to take care of, so make sure you understand the code.
You must implement the function remove_by_value which receives a double pointer to the head and removes the first item in the list which has the value val .
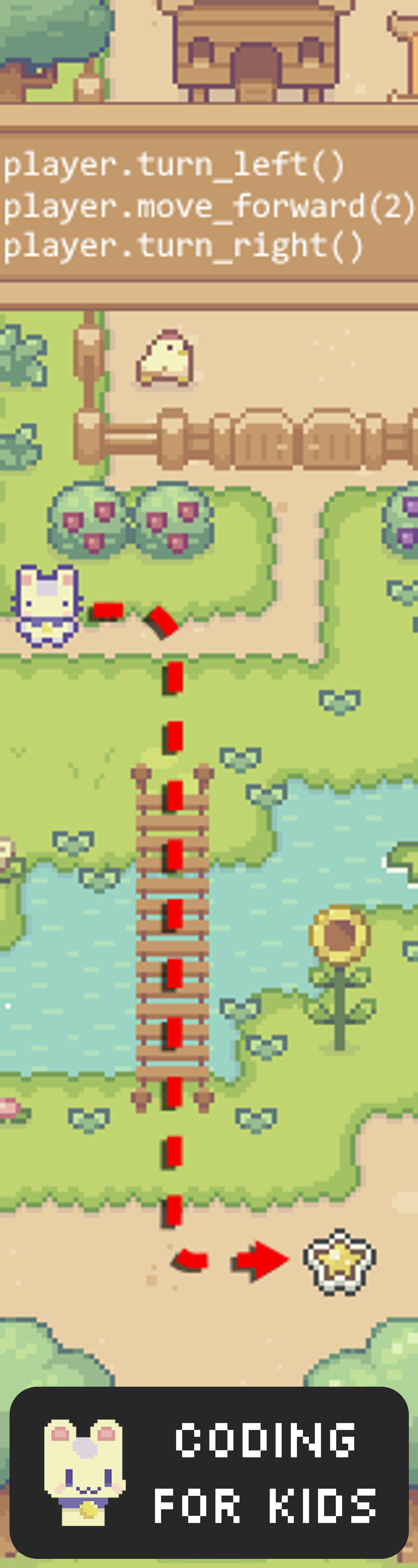
Coding for Kids is an online interactive tutorial that teaches your kids how to code while playing!
Receive a 50% discount code by using the promo code:
Start now and play the first chapter for free, without signing up.
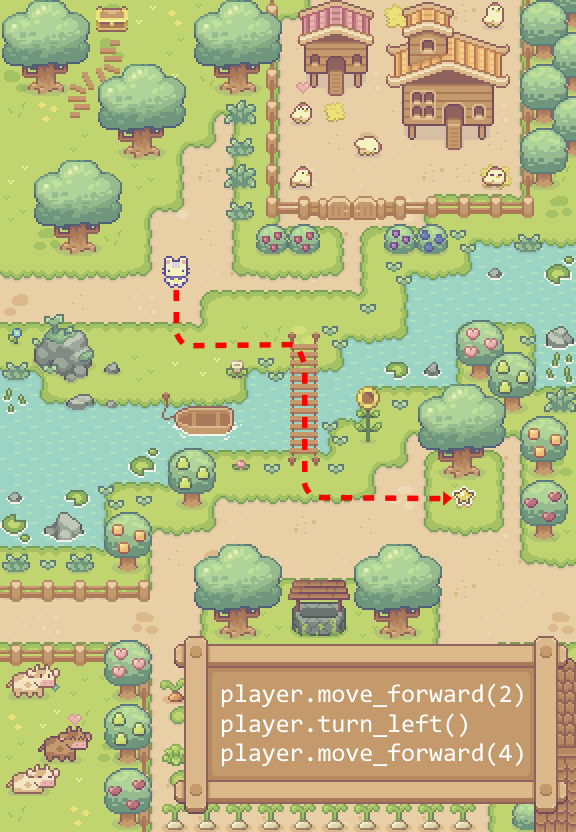
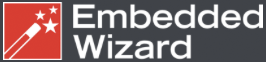
Welcome to Embedded Wizard
Basic concepts
Quick Tour - a Tutorial
Working with Embedded Wizard
Build Environments
Embedded Wizard Studio
Programming language Chora
Project members
Member attributes
Expressions
+=, -=, *=, /=, %=, <<=, >>=, &=, |= ^=
Index operator []
Type conversion
Instant properties
Instant methods
Instant constructors
Built-in variables
Built-in functions
Built-in macros
Compiler directives
Framework Mosaic
Platform Integration Aspects
Release notes
Operators: =
Assignment operator.
destination‑operand = operand
The assignment operator = assigns the value of the right operand to the left destination operand. If the destination operand is a variable , an array , a local variable or a local array , then the value is written directly into the memory of the affected variable or array. If the left operand is a property , then the property associated onset method is called with the value passed in its value parameter. For example:
var string localVariable ; array rect localArray [ 4 ]; // Assign a value to a local variable localVariable = "Hello World" ; // Assign a value to a local array element localArray [ 0 ] = <100,200,110,220> ; // Assign a value to an object variable someObject . ColorVariable = SomeTextView . Color ; // Assign a value to an object array someObject . StringArray [ 5 ] = SomeTextView . String + "!" ; // Assign a value to a property SomeTextView . Bounds = Bounds . orect ;
Implicit type conversion
The Chora assignment operator subjects the value of the right operand to an implicit (automatic) type conversion if the data type of the right operand does not match the data type of the destination operand. For example:
var int32 a = 1369 ; var float b ; // Convert automatically the int32 value to the float data type b = a ; // b = 1369.0
Chained assignment
An assignment operation can be embedded as operand within another more complex operation. In this way a single expression can perform multiple assignments to multiple variables or the assignments can be chained. Following example demonstrates the function. Here you can consider the single expression as being composed of two partial expressions d = a + b and e = d + c :
var int32 a = 10 ; var int32 b = 20 ; var int32 c = 30 ; var int32 d ; var int32 e ; e = ( d = a + b ) + c ; // d = 30, e = 60
When chaining assignment operators you should note the data type resulting from the nested assignment. As explained in the section above the assignment operator performs an implicit type conversion to ensure that the assigned value does correctly conform the data type of the destination operand. If this assignment is part of another more complex expression, the value it represents as operand within this expression is the value after the implicit type conversion took place.
The following example performs a chained assignment of the value 300 first to an int8 integer variable b and then the result of this operation to an int32 variable c . During the assignment to the variable b the assigned value is converted to fit in the 8-bit integer. Since it is too large, the value 300 is truncated and results in the value 44 . This value 44 is consequently assigned to the variable c :
var int32 a = 300 ; var int8 b ; var int32 c ; c = b = a ; // b = 44, c = 44
Compound assignment
Besides the above explained simple assignment operator = Chora also supports a set of compound assignment operators . These operators combine the assignment = with another regular operation. When executed, the operator first performs the regular operation with the both operands found on its left and right side and then, in second step, assigns the result of the operation to the operand on its left side.
Embedded Wizard is a product of TARA Systems GmbH
Concept and implementation by Paul Banach and Manfred Schweyer
Copyright © 2024 TARA Systems GmbH • About us • Contact • Imprint • Privacy Policy
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Anti-idiom? Chained equality operators
I got a headscratcher: someone submitted code to test whether all of some checkboxes were unchecked, and it indicated True when an even number were checked. The code looked something like:
and I read it naively as: if each one is false... But this was incorrect. I figured out why (C# evaluates from left to right, and the result of a boolean compare is a boolean: false == false evaluates to true), but I wondered if this shows up often, and has a name? I guess I would name it Chained Falsehood or maybe False Decay as it would be fine if all operands were True.
The same idea works fine with assignment, so I can see why the coder tried it this way.
Ok, based on comments, this should not be called an anti-pattern because it is a bug . I think it should be called an idiom from another language. But vanity of vanities, thy name is Python , and its name should be called Haddock's Ayes .
- coding-style
- boolean-logic
- 4 This is my first time seeing something like this. I don't think it's a pattern. Maybe just call it an "anti"? :) – Samuel Commented Sep 27, 2017 at 18:57
- 3 seems like a bug more than a pattern – yitzih Commented Sep 27, 2017 at 19:55
- 2 I don't think the criteria for a pattern (or anti-pattern) is as simple as "more than one person does it". A pattern is more of an established practice for solving a particular type of problem. Just because a few people use it here or there doesn't mean it rises to the level of pattern. We would have millions of patterns if that were the case. – Eric King Commented Sep 27, 2017 at 20:01
- 4 @Newtopian It's definitely not a bug in C# except perhaps not warning against/disallowing it. In my opinion, Python's support for this is a misfeature. If nothing else, Python is clearly the outlier here. (In math, there's usually a distinction between propositions and the objects of the language so x = y = z is unambiguous, as ( x = y ) = z is not even syntactically correct. If we were talking about = as a Boolean operation, i.e. <->, then x <-> y <-> z would behave like the C# if <-> had the appropriate associativity, but it is clearest for it to be non-associative.) – Derek Elkins left SE Commented Sep 27, 2017 at 21:13
- 4 How about The Boolean Centipede in reference to imdb.com/title/tt1467304 – Newtopian Commented Sep 27, 2017 at 21:42
5 Answers 5
This is just a coding error due to someone's misunderstanding. I don't know why you think it should have a name. It's definitely not an anti-pattern. An anti-pattern describes a common architectural pattern that negatively impacts maintainability, not a coding error.
I don't know how common this is, but I expect it to be rather rare in any professional context even from junior programmers. I can see it being more common in some introductory programming classes, but it should quickly be discovered to be incorrect. First, the students won't be taught this. Second, even the most cursory of testing will reveal that it doesn't work. Third, the most obvious way of doing this is simpler, namely !box1.checked && !box2.checked && ... && !boxN.checked .
If you are working in a professional context (and arguably even in a non-professional context), the real problem is a process anti-pattern where people are submitting untested code.
- 2 @nocomprende: Still doesn't have a name. Call it "bad coding." – Robert Harvey Commented Sep 27, 2017 at 20:17
- 2 @nocomprende: This isn't off-by-one; it's just bad coding. You can code badly an almost unlimited number of ways; doesn't mean each way deserves a name. – Robert Harvey Commented Sep 27, 2017 at 20:21
- 4 @nocomprende: Further, I think the current generation of programmers' obsession with naming things is actively harmful to our profession. Using arbitrary vocabulary gives the false impression that the people using said vocabulary understand things that they actually do not understand. – Robert Harvey Commented Sep 27, 2017 at 20:28
- 2 @nocomprende Except for cache invalidation. – Derek Elkins left SE Commented Sep 28, 2017 at 1:16
- 2 @RobertHarvey That's so true. Dependency Injection was something a lot of people , including myself, were using even before it had a name. When the term popped up everywhere, it left a lot of people scratching heads trying to understand what was so special there. And worse - a few newbies that didn't grasp the concept but liked the fancy name put their hands on some weird API and ended up writing some horrendous pieces of Injection Hell. – T. Sar Commented Sep 28, 2017 at 11:14
It's not an anti-pattern. To be an anti-pattern, it would first have to be a pattern. Which means it would have to be a common solution to a problem to be a pattern, and a bad common solution to be an anti-pattern. But this is not a common solution to anything.
I'd say it is a WTF. WTF is obviously an abbreviation. You may assume that it means "Worse Than Failure". Or you may assume it means something else.
I have once in my life had to check that at least two of three conditions are true, so I counted how many were true. If I actually needed to do what the code here does (not what it was likely intended to do), I'd write
- 1 Wouldn't this check if exactly an odd number of boxes were checked? This seems different from what your paragraph is talking about which I would render as falseCount >= 2 . – Derek Elkins left SE Commented Sep 27, 2017 at 23:02
- Maybe it is an anti-dark-pattern? Maybe 95% of all code is not visible? – user251748 Commented Sep 28, 2017 at 0:38
- I like the idea of WTF! – T. Sar Commented Sep 28, 2017 at 14:33
I see this every now and then, not on this exact form but in the more general form of "a line of code doing a lot of things".
It could be a pattern if common and misguided ways for trying to achieve premature optimization or to make you look smart qualified as patterns (I am not really sure if they do) and it had a concise name. In that case it would be an antipattern because:
- Premature optimization and looking smart should not be amongst your concerns.
- More often than not it does not buy you any actual optimization (does not really solve this problem).
- Makes you look less smart (does not solve this problem either).
- Makes easier to make mistakes and introduce bugs (just like in your example).
- Obfuscates your intent.
- Makes your code harder to debug.

- If I wrote x = y = z = 5; that would make perfect sense, and probably work in most languages, wouldn't it? x == y == z == false does not work, because the pairs of false == false become true. The false values make it fail where all trues would succeed. They might have done it to look smart, not sure. I don't think it had to do with optimization. It doesn't obfuscate the intent at all, if anything the intent is more clear than the correct formulations, especially because those use negation. – user251748 Commented Sep 27, 2017 at 20:39
- 1 @nocomprende Intent being clear is meaningless when the code is wrong – mmathis Commented Sep 27, 2017 at 21:04
- @mmathis now if we could just create a language where clear intent produced correct code... – user251748 Commented Sep 27, 2017 at 21:28
- 1 @nocomprende I find it hard to read and somewhat obfuscated even if I am used to python. Anyway at this point I am confused about what "this" refers to in "I wondered if this shows up often". Chained comparison? Getting the semantics wrong? Using python idioms in c#? I stand by my claims but it looks like I missed the point of the question. – Stop harming Monica Commented Sep 27, 2017 at 21:41
- 1 @nocomprende: I see what you mean when you say that the intent is more clear; but you're missing something: how would this notation meaningfully be able to differ between && (all of them need to be false) and || (one of them must be false)? The absence of this distinction should reveal that the intent is incomplete . – Flater Commented Sep 28, 2017 at 15:43
I am going to disagree with the other answers here and say this is an anti-pattern as there is a pattern in use. That pattern is using equality to test booleans, rather than using logical operators. eg using if (b == true) , rather than if (b) . A common excuse offered for using this (anti)pattern is that ! is hard to read, so those folk write if (b == false) rather than if (!b) and then use == true for consistency.
This question neatly highlights the dangers with that pattern though. Using logical operators results in code working as expected, so this is an opportunity to educate folk in the idiomatic use of those logical operators:

- What you just did was isolate each part of the Boolean expression--which is the correct thing to do. In essence you now have 5 separate boxX.checked expressions ORed together instead of one like in the OP. I agree that using box1.checked is easier to read than box1.checked == true , but if each expression were written with the longer form, then your answer would essentially be the same. Just more verbose. – Berin Loritsch Commented Sep 28, 2017 at 12:10
- 1 What is really fun is when someone inverts the condition to make it more understandable, which is fine, then has an empty Then body and puts the code in an Else body. So amusing. – user251748 Commented Sep 28, 2017 at 12:14
- I do also find b == true/false unpleasant and common, but this is focusing on a side issue. If the original code was instead checking if all boxes had the same checked state (i.e. all checked or all unchecked), the original author of the code would presumably have written the same thing except omitting the == false at the end. It's completely reasonable to use == to test the equality of Booleans in that case, but, of course, this still can't be written as x == y == z . – Derek Elkins left SE Commented Sep 28, 2017 at 12:21
- 1 I'm not sure if using equality to test booleans is a pattern os just coding style. Patterns usually don't apply to single lines of code, they are somewhat more complex constructs that try to solve a problem. Arguing that this is a pattern open doors for claiming that "using guard conditions" is a pattern, too. I'm not sure they are, but I look at them just as good practices, not a high-level problem solving structure. – T. Sar Commented Sep 28, 2017 at 14:37
- @T.Sar yeah, I decided to change from 'pattern' to 'idiom'. This is indeed an idiom (or at least good code) in Python. Careful where you give that thumb-circle OK sign, eh? – user251748 Commented Sep 28, 2017 at 16:19
There are reasons why it is considered good practice to separate both assignments and Boolean expressions. The number of surprises you can get from implicit converts (if the language supports that) and such increase with each segment in the chain.
This is the first time I've seen a Boolean chain, and hopefully it is the last. I would consider a special case of "chained assignment", which you can find a lot of conversation about it when you search.
More often than not, chained assignments have unexpected side-effects. Most code style guidelines I've seen recommend avoiding them completely.
Since there needs to be multiple instances of anything to be called a pattern, I'd say this type of thing fails that threshold. However it does win a gold star for creative bug writing.

- 6 There are no assignments, chained or unchained, in the question. – Stop harming Monica Commented Sep 27, 2017 at 19:24
- @Goyo, The same problems with chained assignments are affecting this problem. There is an implicit assignment to the if statement at the end of this. Like I said a special case of a well known problem. – Berin Loritsch Commented Sep 27, 2017 at 20:13
- 1 @BerinLoritsch What are you talking about? I don't even know what you mean by an "assignment to the if statement" implicit or otherwise. It's possible that even at the level of assembly literally nothing gets updated other than the instruction pointer, so I'm not sure what you believe is being implicitly assigned to. – Derek Elkins left SE Commented Sep 27, 2017 at 20:35
- x = y = z = 5 would work. I have not seen cases where that is problematic. But when you start throwing Booleans around, false values do strange things. (True values, of course, do not. It is like odd and even numbers, or primes and composite numbers. The world is just weird.) – user251748 Commented Sep 27, 2017 at 20:41
- 1 @Newtopian so what's a few = signs between friends? – user251748 Commented Sep 27, 2017 at 21:29
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Software Engineering Stack Exchange. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
- The Overflow Blog
- Unpacking the 2024 Developer Survey results
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Introducing an accessibility dashboard and some upcoming changes to display...
Hot Network Questions
- When/why did software only engines overtake custom hardware?
- Error using \verb in a footnote in Beamer
- Schengen visa expires during my flight layover
- Why, fundamentally, does adding sin graphs together always produce another sin graph?
- Significance of negative work done
- Tips/strategies to managing my debt
- Best (safest) order of travel for Russia and the USA (short research trip)
- Solar System Replacement?
- Who‘s to say that beliefs held because of rational reasons are indeed more justified than beliefs held because of emotional ones
- Splitting a Number into Random Parts
- How do we know that the number of points on a line is uncountable?
- Why do commercial airliners go around on hard touchdown?
- High-precision solution for the area of a region
- Why do most published papers hit the maximum page limit exactly?
- Set up GeForce GT 620 for Ubuntu 24.04
- Why would sperm cells derived from women's stem cells always produce female children?
- New job, reporting manager is not replying to me after a few days: how to proceed?
- In Europe, are you allowed to enter an intersection on red light in order to allow emergency vehicles to pass?
- Small adjustment or fix to a toilet float to fix constant draining?
- "Seagulls are gulling away."
- In Norway, when number ranges are listed 3 times on a sign, what do they mean?
- Pure emphasis use of 那個?
- How does "regina" derive from "rex"?
- Should I include MA theses in my phd literature review?
- Biology and Medical
- Earth Sciences
- Computer Science
- Computing and Technology
- DIY Projects
Follow along with the video below to see how to install our site as a web app on your home screen.
Note: This feature may not be available in some browsers.
- Other Sciences
- Programming and Computer Science
How, exactly, are chained assignments processed?
- Thread starter in the rye
- Start date Jan 2, 2018
- Jan 2, 2018
A PF Electron
- Research team improves fuel cell durability with fatigue-resistant membranes
- Self-extinguishing batteries could reduce the risk of deadly and costly battery fires
- Chemists decipher reaction process that could improve lithium-sulfur batteries
in the rye said: Hi all, I have a question. I am writing a copy constructor for a class, and I'm not sure this has ever made sense to me. When we write the copy constructor we return *this to support chained assignments. But my question is, why is this required? Suppose we have integers a,b,c where c = 0. If I assign: a = b = c I understand that b = c will assign 0 to b then return 0 so that a is assigned 0. However, why is the return value in the constructor required? It seems to me that if we left it out, b=c should be assigned, return nothing, but does this means that the b=c just drops out from the expression entirely so that we have nothing on the rhs? It just seems like a should be assigned 0 regardless. Since b=c will be assigned 0 and now a is being assigned to b and b is now 0. The return value doesn't seem to be necessary, but obviously I'm wrong and I want to know why. Thanks.
A PF Organism
Related to how, exactly, are chained assignments processed, 1. how are chained assignments processed.
Chained assignments are processed from right to left. This means that the value on the right side of the assignment is assigned to the variable on the left, and then the value of that variable is assigned to the next variable on the left, and so on.
2. What happens if one of the variables in a chained assignment is already defined?
If one of the variables is already defined, its value will be overwritten by the value on the right side of the assignment. This value will then be passed on to the next variable on the left, and so on.
3. Can I chain more than three variables in an assignment?
Yes, you can chain as many variables as you want in an assignment. There is no limit to the number of variables that can be chained together.
4. How does chaining assignments affect the performance of my code?
Chaining assignments does not have a significant impact on the performance of your code. In fact, it can sometimes even improve performance, as it reduces the number of lines of code and can make the code more concise.
5. Are there any potential pitfalls to be aware of when using chained assignments?
One potential pitfall to be aware of is accidentally reusing a variable name in a chained assignment. This can lead to unexpected results and can be difficult to debug. It is important to use unique variable names to avoid this issue.
Similar threads
- Dec 9, 2020
- Dec 15, 2020
- Feb 6, 2023
- Feb 4, 2023
- Mar 4, 2021
- Apr 17, 2017
- Dec 29, 2020
- Oct 17, 2023
- Mar 30, 2017
- Oct 15, 2023
Hot Threads
- Python Most efficient way to randomly choose a word from a file with a list of words
- Is an API Always Necessary for Server-Client Communication?
- Python Python Socket library to create a server and client scripts
- JavaScript How do I run an exe file on an Apple iPad?
- Git, staging and committing files
Recent Insights
- Insights PBS Video Comment: “What If Physics IS NOT Describing Reality”
- Insights Aspects Behind the Concept of Dimension in Various Fields
- Insights Views On Complex Numbers
- Insights Addition of Velocities (Velocity Composition) in Special Relativity
- Insights Schrödinger’s Cat and the Qbit
- Insights The Slinky Drop Experiment Analysed
Copy-on-Write (CoW) #
Copy-on-Write will become the default in pandas 3.0. We recommend turning it on now to benefit from all improvements.
Copy-on-Write was first introduced in version 1.5.0. Starting from version 2.0 most of the optimizations that become possible through CoW are implemented and supported. All possible optimizations are supported starting from pandas 2.1.
CoW will be enabled by default in version 3.0.
CoW will lead to more predictable behavior since it is not possible to update more than one object with one statement, e.g. indexing operations or methods won’t have side-effects. Additionally, through delaying copies as long as possible, the average performance and memory usage will improve.
Previous behavior #
pandas indexing behavior is tricky to understand. Some operations return views while other return copies. Depending on the result of the operation, mutating one object might accidentally mutate another:
Mutating subset , e.g. updating its values, also updates df . The exact behavior is hard to predict. Copy-on-Write solves accidentally modifying more than one object, it explicitly disallows this. With CoW enabled, df is unchanged:
The following sections will explain what this means and how it impacts existing applications.
Migrating to Copy-on-Write #
Copy-on-Write will be the default and only mode in pandas 3.0. This means that users need to migrate their code to be compliant with CoW rules.
The default mode in pandas will raise warnings for certain cases that will actively change behavior and thus change user intended behavior.
We added another mode, e.g.
that will warn for every operation that will change behavior with CoW. We expect this mode to be very noisy, since many cases that we don’t expect that they will influence users will also emit a warning. We recommend checking this mode and analyzing the warnings, but it is not necessary to address all of these warning. The first two items of the following lists are the only cases that need to be addressed to make existing code work with CoW.
The following few items describe the user visible changes:
Chained assignment will never work
loc should be used as an alternative. Check the chained assignment section for more details.
Accessing the underlying array of a pandas object will return a read-only view
This example returns a NumPy array that is a view of the Series object. This view can be modified and thus also modify the pandas object. This is not compliant with CoW rules. The returned array is set to non-writeable to protect against this behavior. Creating a copy of this array allows modification. You can also make the array writeable again if you don’t care about the pandas object anymore.
See the section about read-only NumPy arrays for more details.
Only one pandas object is updated at once
The following code snippet updates both df and subset without CoW:
This won’t be possible anymore with CoW, since the CoW rules explicitly forbid this. This includes updating a single column as a Series and relying on the change propagating back to the parent DataFrame . This statement can be rewritten into a single statement with loc or iloc if this behavior is necessary. DataFrame.where() is another suitable alternative for this case.
Updating a column selected from a DataFrame with an inplace method will also not work anymore.
This is another form of chained assignment. This can generally be rewritten in 2 different forms:
A different alternative would be to not use inplace :
Constructors now copy NumPy arrays by default
The Series and DataFrame constructors will now copy NumPy array by default when not otherwise specified. This was changed to avoid mutating a pandas object when the NumPy array is changed inplace outside of pandas. You can set copy=False to avoid this copy.
Description #
CoW means that any DataFrame or Series derived from another in any way always behaves as a copy. As a consequence, we can only change the values of an object through modifying the object itself. CoW disallows updating a DataFrame or a Series that shares data with another DataFrame or Series object inplace.
This avoids side-effects when modifying values and hence, most methods can avoid actually copying the data and only trigger a copy when necessary.
The following example will operate inplace with CoW:
The object df does not share any data with any other object and hence no copy is triggered when updating the values. In contrast, the following operation triggers a copy of the data under CoW:
reset_index returns a lazy copy with CoW while it copies the data without CoW. Since both objects, df and df2 share the same data, a copy is triggered when modifying df2 . The object df still has the same values as initially while df2 was modified.
If the object df isn’t needed anymore after performing the reset_index operation, you can emulate an inplace-like operation through assigning the output of reset_index to the same variable:
The initial object gets out of scope as soon as the result of reset_index is reassigned and hence df does not share data with any other object. No copy is necessary when modifying the object. This is generally true for all methods listed in Copy-on-Write optimizations .
Previously, when operating on views, the view and the parent object was modified:
CoW triggers a copy when df is changed to avoid mutating view as well:
Chained Assignment #
Chained assignment references a technique where an object is updated through two subsequent indexing operations, e.g.
The column foo is updated where the column bar is greater than 5. This violates the CoW principles though, because it would have to modify the view df["foo"] and df in one step. Hence, chained assignment will consistently never work and raise a ChainedAssignmentError warning with CoW enabled:
With copy on write this can be done by using loc .

Read-only NumPy arrays #
Accessing the underlying NumPy array of a DataFrame will return a read-only array if the array shares data with the initial DataFrame:
The array is a copy if the initial DataFrame consists of more than one array:
The array shares data with the DataFrame if the DataFrame consists of only one NumPy array:
This array is read-only, which means that it can’t be modified inplace:
The same holds true for a Series, since a Series always consists of a single array.
There are two potential solution to this:
Trigger a copy manually if you want to avoid updating DataFrames that share memory with your array.
Make the array writeable. This is a more performant solution but circumvents Copy-on-Write rules, so it should be used with caution.
Patterns to avoid #
No defensive copy will be performed if two objects share the same data while you are modifying one object inplace.
This creates two objects that share data and thus the setitem operation will trigger a copy. This is not necessary if the initial object df isn’t needed anymore. Simply reassigning to the same variable will invalidate the reference that is held by the object.
No copy is necessary in this example. Creating multiple references keeps unnecessary references alive and thus will hurt performance with Copy-on-Write.
Copy-on-Write optimizations #
A new lazy copy mechanism that defers the copy until the object in question is modified and only if this object shares data with another object. This mechanism was added to methods that don’t require a copy of the underlying data. Popular examples are DataFrame.drop() for axis=1 and DataFrame.rename() .
These methods return views when Copy-on-Write is enabled, which provides a significant performance improvement compared to the regular execution.
How to enable CoW #
Copy-on-Write can be enabled through the configuration option copy_on_write . The option can be turned on __globally__ through either of the following:
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
C Logical Operators
Logical operators in C are used to combine multiple conditions/constraints. Logical Operators returns either 0 or 1, it depends on whether the expression result is true or false. In C programming for decision-making, we use logical operators.
We have 3 logical operators in the C language:
- Logical AND ( && )
- Logical OR ( || )
- Logical NOT ( ! )
Types of Logical Operators
1. logical and operator ( && ).
The logical AND operator (&&) returns true only if both operands are non-zero. Otherwise, it returns false (0). The return type of the result is int. Below is the truth table for the logical AND operator.
X | Y | X && Y |
---|---|---|
1 | 1 | 1 |
1 | 0 | 0 |
0 | 1 | 0 |
0 | 0 | 0 |
2. Logical OR Operator ( || )
The logical OR operator returns true if any one of the operands is non-zero. Otherwise, it returns false i.e., 0 as the value. Below is the truth table for the logical OR operator.
X | Y | X || Y |
---|---|---|
1 | 1 | 1 |
1 | 0 | 1 |
0 | 1 | 1 |
0 | 0 | 0 |
3. Logical NOT Operator ( ! )
If the given operand is true then the logical NOT operator will make it false and vice-versa. Below is the truth table for the logical NOT operator.
X | !X |
---|---|
0 | 1 |
1 | 0 |
Short Circuit Logical Operators
When the result can be determined by evaluating the preceding Logical expression without evaluating the further operands, it is known as short-circuiting.
Short-circuiting can be seen in the equation having more than one Logical operator. They can either AND, OR, or both.
1. Short Circuiting in Logical AND Operator
The logical AND operator returns true if and only if all operands evaluate to true. If the first operand is false, then the further operands will not be evaluated. This is because even if the further operands evaluate to true, the entire condition will still return false.
2. Short Circuiting in Logical OR Operator
OR operator returns true if at least one operand evaluates to true. If the first operand is true, then the further operands will not be evaluated. This is because even if the further operands evaluate to false, the entire condition will still return true.
FAQs on Logical operators
Q1. what is the precedence of logical operators in programming.
The precedence of logical operators is: NOT, AND, OR. However, it is always recommended to use parentheses to make the order of evaluation explicit and avoid confusion.
Q2. Can logical operators be chained together?
Yes, logical operators can be chained together to create complex conditions. For example, we can combine multiple logical AND (&&) or logical OR (||) operators in a single expression to evaluate multiple conditions simultaneously.
Q3. What will be the output of the following code?
Q4. what will be the output of the following code.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?

The Assignment with Audie Cornish
Every thursday on the assignment, host audie cornish explores the animating forces of this extraordinary american political moment. it’s not about the horse race, it’s about the larger cultural ideas driving the conversation: the role of online influencers on the electorate, the intersection of pop culture and politics, and discussions with primary voices and thinkers who are shaping the political conversation..
- Apple Podcasts

Back to episodes list
CNN’s Elle Reeve did her best-known reporting during the 2017 Unite the Right rally in Charlottesville, Virginia, when she followed neo-Nazis over a weekend of violent protests. Seven years later, her new book looks at how that movement — born in online communities of mostly white men — gave rise to extremist thinking that is now threaded through today’s political discourse.
Audie talks with Reeve about reporting on Nazis, the mainstreaming of their ideology, and why she started “ Black Pill: How I Witnessed the Darkest Corners of the Internet Come to Life, Poison Society, and Capture American Politics ” with a story about a dead cat.
© 2024 Cable News Network. A Warner Bros. Discovery Company. All Rights Reserved. CNN Audio's transcripts are made available as soon as possible. They are not fully edited for grammar or spelling and may be revised in the future. The audio record represents the final version of CNN Audio.
Get the Reddit app
A place to get a quick fix of python tips and tricks to make you a better Pythonista.
Python chained assignment (a=b=0)
When assigning variables to the same number for some kind of initialization, rather than doing
or using tuple unpacking
you can actually do
Note that this can be used with any object on the RHS, and that:
is equivalent to
Note that the chain length for assignment can be longer than just two variables, so a = b = c = d = 0 is valid (though it probably gets a bit ugly if you go too far). Specifically, what happens is that the far RHS is evaluated and then from left to right assignments of the variables are made to it. This is different to C .
Obviously, you should be careful if you use this when assigning to mutable objects (such as lists), because of the typical object reference trip-ups in python that can be seen here ; it means that
Still, I think it looks a bit neater in some cases, is very nice for number assignment and is a cool little tip :)
The specific python documentation is here , and you can read more about them on stack overflow here and here
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
Python Assignment Operator
Python assignment sequence unpacking, python chained assignments, python arithmetic operators, python integer arithmetic, python negative number division, python float arithmetic, python complex num operator, python compound assignment, python comparison operators, python logical operators, python in operator, python is operator, python convert kilometer to mile, python operator exercise 1, python operator exercise 2, python operator exercise 3, python variable swap two numbers using a single line of code.
Chained assignments are used as a shortcut when you want to bind several variables to the same value.
which is the same as this:
Note that the preceding statements may not be the same as.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How are chained assignments in java defined? Is there a difference between value and reference types?
How are chained assignments in java defined, considering following points:
- Is there a difference between chained assignment and chained declaration?
- Is there a way for reference types to repeat the statement instead of passing the reference?
In JLS 15.26 Assignment Operators it says
At run time, the result of the assignment expression is the value of the variable after the assignment has occurred. The result of an assignment expression is not itself a variable.
So a == b should be true.
Is there a way to achieve
in one line so that a != b , since a and b are different objects.
Additional Info
The question is already answered, but I felt it was not clear enough, so here some code to clarify it.
- variable-assignment
- assignment-operator

- Generally Integers from -128 to 127 are cached in memory so you will never get a != b in your example, but I get your point. – ddmps Commented Aug 13, 2013 at 14:50
- @Pescis a != b as in reference equality, not value equality. – Sotirios Delimanolis Commented Aug 13, 2013 at 14:51
- I mixed in a mistake Integer a, b = new Integer(4); leaves a null – mike Commented Aug 13, 2013 at 14:51
- 2 @Pescis: new Integer will always return a reference to a new object. This isn't boxing or using Integer.valueOf . – Jon Skeet Commented Aug 13, 2013 at 14:51
Personally I think that's less readable than using two separate declarations though, and there's no way of doing it without either repeating the new Integer(4) or extracting that to some other method which you then call twice.
- Thought maybe there was a way, where I don't have to repeat the constructor call. Thx! – mike Commented Aug 13, 2013 at 14:54
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged java variable-assignment assignment-operator or ask your own question .
- The Overflow Blog
- Unpacking the 2024 Developer Survey results
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Introducing an accessibility dashboard and some upcoming changes to display...
- Tag hover experiment wrap-up and next steps
Hot Network Questions
- English equilvant to this hindi proverb "A washerman's dog belongs neither at home nor at the riverbank."?
- How bright would nights be if the moon were terraformed?
- Approximating a partition
- Significance of negative work done
- Splitting a Number into Random Parts
- How do Driftgloom Coyote and Sugar Coat interact?
- How does Chakotay know about the mirror universe?
- New job, reporting manager is not replying to me after a few days: how to proceed?
- Pass ethernet directly to VM and use WiFi for OS
- Is there such a thing as icing in the propeller?
- Tips/strategies to managing my debt
- Where is the Minor Reflection spell?
- GAMs and random effects: Significant differences between GAMM and GAMM4 outputs
- Fantasy book in which a joker wonders what's at the top of a stone column
- Search values by priority in stream
- When/why did software only engines overtake custom hardware?
- Why did all countries converge on the exact same rules for voting (no poll tax, full suffrage, no maximum age, etc)?
- Solar System Replacement?
- A finance broker made me the primary instead of a co-signer
- How can I select all pair of two points A and B has integer coordinates and length of AB is also integer?
- Iterative mixing problem
- How to use Mathematica to plot following helix solid geometry?
- What does "No camping 10-21" mean?
- Set all numbers greater than "X" bold, for decimal numbers

IMAGES
COMMENTS
As you see there is a difference. In C++ the assignment operator returns an lvalue referring to the left operand while in C it returns the value of the left operand after the assignment,111) but is not an lvalue. It means that in C++ the following code is valid. int a, b = 20; ( a = 10 ) = b;
If you have a bunch of them, then perhaps save the logical groups of them in a set, and then apply an attribute change to the whole set with aid of a helper function. Still, as Robert said, try to minimize state! Frankly, try to simplify the UI first. You can also logically separate controls by groupboxes, or give them a specific Tag, and then ...
Bucket Index: The value returned by the Hash function is the bucket index for a key in a separate chaining method. Each index in the array is called a bucket as it is a bucket of a linked list. Rehashing: Rehashing is a concept that reduces collision when the elements are increased in the current hash table. It will make a new array of doubled size and copy the previous array elements to it ...
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators.This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left.
Assignment (computer science) In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location (s) denoted by a variable name; in other words, it copies a value into the variable. In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.
Learn about embedded assignments, chained assignments, and compound assignments in C++. Explore examples to understand how these assignment techniques work and how they can be used to assign values to variables efficiently. Gain insights into the compound assignment operator and its role in simplifying complex assignments in C++ programming
We execute "B = the reference to C" and return a reference to B. We execute "A = the reference to B" and return a reference to A. If the assignment operator did not return anything at all, we could not have chained assignment statements, which are part of the overall C++ language. What this example does is more or less exactly what the default ...
Essentially, linked lists function as an array that can grow and shrink as needed, from any point in the array. Linked lists have a few advantages over arrays: Items can be added or removed from the middle of the list. There is no need to define an initial size. However, linked lists also have a few disadvantages:
If this assignment is part of another more complex expression, the value it represents as operand within this expression is the value after the implicit type conversion took place. The following example performs a chained assignment of the value 300 first to an int8 integer variable b and then the result of this operation to an int32 variable c.
This is the first time I've seen a Boolean chain, and hopefully it is the last. I would consider a special case of "chained assignment", which you can find a lot of conversation about it when you search. More often than not, chained assignments have unexpected side-effects. Most code style guidelines I've seen recommend avoiding them completely.
Chained assignments are processed from right to left. This means that the value on the right side of the assignment is assigned to the variable on the left, and then the value of that variable is assigned to the next variable on the left, and so on. 2. What happens if one of the variables in a chained assignment is already defined?
Check the chained assignment section for more details. Accessing the underlying array of a pandas object will return a read-only view. In [10]: ser = pd. Series ([1, 2, 3]) In [11]: ser. to_numpy Out[11]: array([1, 2, 3]) This example returns a NumPy array that is a view of the Series object. This view can be modified and thus also modify the ...
C Logical Operators. Last Updated : 29 Jul, 2024. Logical operators in C are used to combine multiple conditions/constraints. Logical Operators returns either 0 or 1, it depends on whether the expression result is true or false. In C programming for decision-making, we use logical operators. We have 3 logical operators in the C language:
assignment policy is mco 5060.20, marine corps drill and ceremonies manual. ref c is mco 5060.20, marine corps drill and ceremonies manual.// poc/j. f. richard jr/sgtmaj/unit: marbks washington,d.c.//
Example 2 Setting pd.options.mode.chained_assignment = "warn" results in the following output (a warning is printed, but no exception). pd.options.mode.chained_assignment : warn A B 0 0 3 1 1 4 2 2 5 NO EXCEPTION C:\Users\my.name\my\directory\test.py:14:SettingWithCopyWarning: A value is trying to be set on a copy of a slice from a DataFrame.
The Assignment is a production of CNN audio. This episode was produced by Isoke Samuel and Graelyn Brashear. Our senior producer is Matt Martinez. Dan Dzula is our technical director, and Steve ...
"Transforming mental health is a long game, it takes time," said Giuseppe Raviola, a director at Partners In Health, which also set up a mental help line in Sierra Leone last year for ...
Chained assignment is essentially when you are assigning a value to something which will be returning something then further indexed upon. For example, the following code: a b. is a chained assignment. First, a reference to the pd.Series object df['a'] is gotten, then is further indexed upon with [0]. This will work in some cases (like this one ...
Python chained assignment (a=b=0) When assigning variables to the same number for some kind of initialization, rather than doing. or using tuple unpacking. you can actually do. Note that this can be used with any object on the RHS, and that: is equivalent to. Note that the chain length for assignment can be longer than just two variables, so a ...
The SettingWithCopyWarning was created to flag potentially confusing "chained" assignments, such as the following, which does not always work as expected, particularly when the first selection returns a copy. [see GH5390 and GH5597 for background discussion.]. df[df['A'] > 2]['B'] = new_val # new_val not set in df The warning offers a suggestion to rewrite as follows:
Chained assignments are used as a shortcut when you want to bind several variables to the same value. x = y = somefunction() which is the same as this: y = somefunction() x = y. Note that the preceding statements may not be the same as. x = somefunction()
I do not really get how the chained assignment concept in Pandas works and how the usage of .ix(), .iloc(), or .loc() affects it. I get the SettingWithCopyWarning warnings for the following lines of code, where data is a Panda dataframe and amount is a column (Series) name in that dataframe:
一度クリアしているのでキャリー枠の者です@sunazame @torippy_channel @mutuu_gaming 気に入ったらチャンネル登録しておくれ → https ...
In JLS 15.26 Assignment Operators it says. At run time, the result of the assignment expression is the value of the variable after the assignment has occurred. The result of an assignment expression is not itself a variable. So a == b should be true. Is there a way to achieve. Integer a = new Integer(4) Integer b = new Integer(4)