- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries

Python | Extract numbers from string
Many times, while working with strings we come across this issue in which we need to get all the numeric occurrences. This type of problem generally occurs in competitive programming and also in web development. Let’s discuss certain ways in which this problem can be solved in Python .
Extract Numbers from a String in Python
Below are the methods that we will cover in this article:
- Using List comprehension and isdigit() method
- Using re.findall() method
- Using isnumeric() method
- Using Filter() function
- Using a loop and isdigit() method
- Using str.translate() with str.maketrans()
- Using numpy module
Extract numbers from string using list comprehension and isdigit() method
This problem can be solved by using the split function to convert string to list and then the list comprehension which can help us iterate through the list and isdigit function helps to get the digit out of a string.
Time Complexity: O(n), where n is the number of elements in the input string. Auxiliary Space: O(n), where n is the number of numbers in the input string.
Extract Digit from string using re.findall() method
This particular problem can also be solved using Python regex, we can use the findall function to check for the numeric occurrences using a matching regex string.
Extract Interger from string using isnumeric() method
In Python, we have isnumeric function which can tell the user whether a particular element is a number or not so by this method we can also extract the number from a string.
Time Complexity : O(N) Auxiliary Space : O(N)
Extract Digit from string using Filter() function
First, we define the input string then print the original string and split the input string into a list of words using the split() method. Use the filter() function to filter out non-numeric elements from the list by applying the lambda function x .isdigit() to each elementConvert the remaining elements in the filtered list to integers using a list comprehension
Print the resulting list of integers
Time complexity: O(n), where n is the length of the input string. The split() method takes O(n) time to split the input string into a list of words, and the filter() function takes O(n) time to iterate over each element in the list and apply the lambda function. The list comprehension takes O(k) time, where k is the number of elements in the filtered list that are digits, and this is typically much smaller than n. Therefore, the overall time complexity is O(n).
Auxiliary space complexity: O(n), as the split() method creates a list of words that has the same length as the input string, and the filter() function creates a filtered list that can be up to the same length as the input list. The list comprehension creates a new list of integers that is typically much smaller than the input list, but the space complexity is still O(n) in the worst case. Therefore, the overall auxiliary space complexity is O(n)
Extract Interger from string using a loop and isdigit() method
Use a loop to iterate over each character in the string and check if it is a digit using the isdigit() method. If it is a digit, append it to a list.
Time complexity: O(n), where n is the length of the string. Auxiliary space: O(k), where k is the number of digits in the string.
Extract Numbers from string using str.translate() with str.maketrans()
Define the input string then Initialize a translation table to remove non-numeric characters using str. maketrans() . Use str. translate() with the translation table to remove non-numeric characters from the string and store the result in a new string called numeric_string . Use str. split() to split the numeric_string into a list of words and store the result in a new list called words. Initialize an empty list called numbers to store the resulting integers and then iterate over each word in the list of words. Check if the word is a numeric string using str. isdigit() .If the word is a numeric string, convert it to an integer using int() and append it to the list of numbers.
Print the resulting list of integers.
Below is the implementation of the above approach:
Time complexity: O(n), where n is the length of the input string. The str.translate() method and str.split() method take O(n) time, and iterating over each word in the list of words takes O(k) time, where k is the number of words in the list that are numeric strings. Auxiliary Space: O(n), as we create a new string and a new list of words that each have the same length as the input string, and we create a new list of integers that has a maximum length of k, where k is the number of words in the list that are numeric strings.
Extract Numbers from string using numpy module
Initialize the string test_string then split the string into a list of words using the split method and create a numpy array x from the resulting list. Use np.char .isnumeric to create a boolean mask indicating which elements of x are numeric. Use this boolean mask to index x and extract only the numeric elements. Convert the resulting array of strings to an array of integers using astype.
Print the resulting array of integers.
Time complexity: O(n), where n is the length of the original string test_string. This is because the split method takes O(n) time to split the string into a list of words, and the np.char.isnumeric method takes O(n) time to create the boolean mask. The remaining operations take constant time.
Auxiliary Space: O(n), where n is the length of the original string test_string. This is because we create a numpy array x to store the words of the string, which takes O(n) space. The space used by the resulting numpy array of integers is also O(n), since it contains all the numeric elements of the string.
Please Login to comment...
Similar reads.
- Python Programs
- Python string-programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
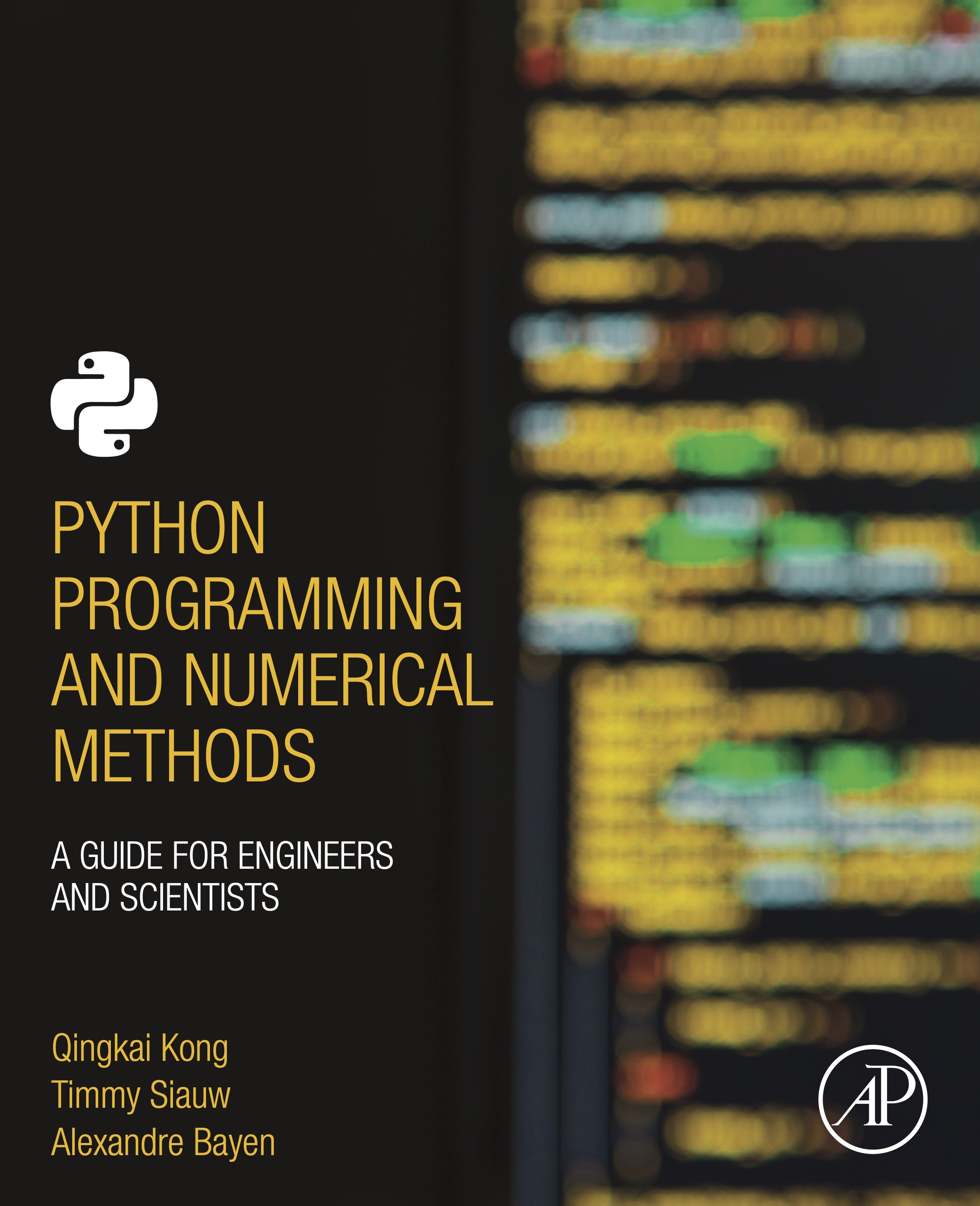
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
Python Flow Control
- Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers and Mathematics
- Python List
- Python Tuple
- Python String
- Python Dictionary
- Python Functions
- Python Function Arguments
Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python Data Types
Python String strip()
- Python String isalnum()
- Python String lower()
Python Strings
In Python, a string is a sequence of characters. For example, "hello" is a string containing a sequence of characters 'h' , 'e' , 'l' , 'l' , and 'o' .
We use single quotes or double quotes to represent a string in Python. For example,
Here, we have created a string variable named string1 . The variable is initialized with the string "Python Programming" .
- Example: Python String
In the above example, we have created string-type variables: name and message with values "Python" and "I love Python" respectively.
Here, we have used double quotes to represent strings, but we can use single quotes too.
- Access String Characters in Python
We can access the characters in a string in three ways.
- Indexing : One way is to treat strings as a list and use index values. For example,
- Negative Indexing : Similar to a list, Python allows negative indexing for its strings. For example,
- Slicing : Access a range of characters in a string by using the slicing operator colon : . For example,
Note : If we try to access an index out of the range or use numbers other than an integer, we will get errors.
- Python Strings are Immutable
In Python, strings are immutable. That means the characters of a string cannot be changed. For example,
However, we can assign the variable name to a new string. For example,
- Python Multiline String
We can also create a multiline string in Python. For this, we use triple double quotes """ or triple single quotes ''' . For example,
In the above example, anything inside the enclosing triple quotes is one multiline string.
- Python String Operations
Many operations can be performed with strings, which makes it one of the most used data types in Python.
1. Compare Two Strings
We use the == operator to compare two strings. If two strings are equal, the operator returns True . Otherwise, it returns False . For example,
In the above example,
- str1 and str2 are not equal. Hence, the result is False .
- str1 and str3 are equal. Hence, the result is True .
2. Join Two or More Strings
In Python, we can join (concatenate) two or more strings using the + operator.
In the above example, we have used the + operator to join two strings: greet and name .
- Iterate Through a Python String
We can iterate through a string using a for loop . For example,
- Python String Length
In Python, we use the len() method to find the length of a string. For example,
- String Membership Test
We can test if a substring exists within a string or not, using the keyword in .
- Methods of Python String
Besides those mentioned above, there are various string methods present in Python. Here are some of those methods:
Methods | Description |
---|---|
Converts the string to uppercase | |
Converts the string to lowercase | |
Returns a tuple | |
Replaces substring inside | |
Returns the index of the first occurrence of substring | |
Removes trailing characters | |
Splits string from left | |
Checks if string starts with the specified string | |
Checks numeric characters | |
Returns index of substring |
- Escape Sequences in Python
The escape sequence is used to escape some of the characters present inside a string.
Suppose we need to include both a double quote and a single quote inside a string,
Since strings are represented by single or double quotes, the compiler will treat "He said, " as a string. Hence, the above code will cause an error.
To solve this issue, we use the escape character \ in Python.
Here is a list of all the escape sequences supported by Python.
Escape Sequence | Description |
---|---|
Backslash | |
Single quote | |
Double quote | |
ASCII Bell | |
ASCII Backspace | |
ASCII Formfeed | |
ASCII Linefeed | |
ASCII Carriage Return | |
ASCII Horizontal Tab | |
ASCII Vertical Tab | |
Character with octal value ooo | |
Character with hexadecimal value HH |
- Python String Formatting (f-Strings)
Python f-Strings makes it easy to print values and variables. For example,
Here, f'{name} is from {country}' is an f-string .
This new formatting syntax is powerful and easy to use. From now on, we will use f-Strings to print strings and variables.
- Python str()
- Python String Interpolation
Table of Contents
Write a function to double every letter in a string.
- For input 'hello' , the return value should be 'hheelllloo' .
Video: Python Strings
Sorry about that.
Related Tutorials
Python Tutorial
Python Library
Join us and get access to thousands of tutorials and a community of expert Pythonistas.
This lesson is for members only. Join us and get access to thousands of tutorials and a community of expert Pythonistas.
Working With Strings and Numbers
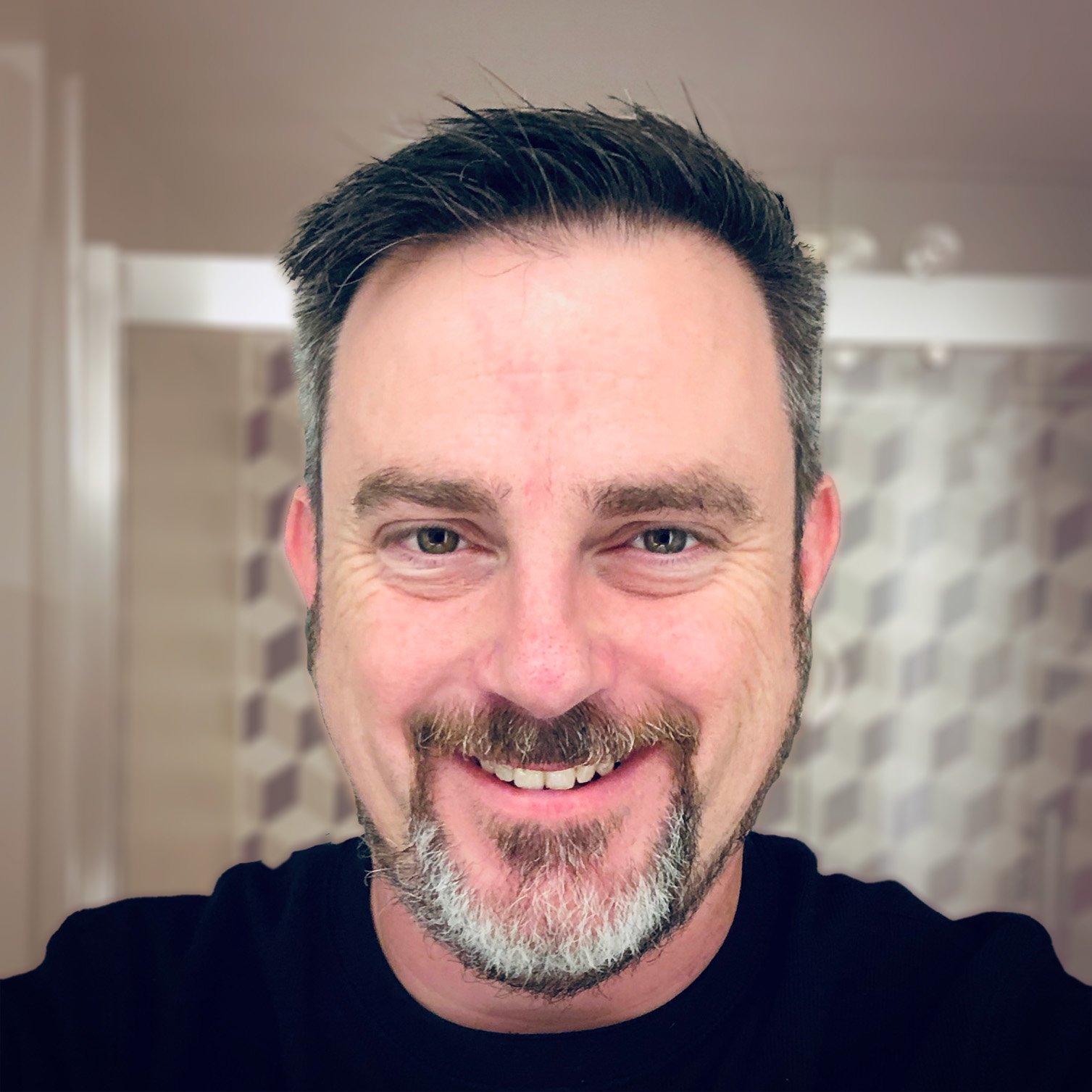
- Discussion (1)
00:00 Now that you’ve worked with user input a bit, let’s talk about working with strings and numbers.
00:07 When you get user input using the built-in input() function, the result is always a string. There are many other situations in which input is given to a program as a string. Sometimes those strings contain numbers, and they need to be fed into calculations. In this lesson, you’ll learn how to deal with strings of numbers.
00:27 And you’ll also see how arithmetic operations work on strings and how they often lead to surprising results. Then you’ll learn how to convert between strings and number types.
00:39 Let’s start this out in the interactive window.
00:44 Try this out. I’ll have you create a variable called num and then assign it the string with the number "2" in it. So this is num , a string literal with "2" in it.
00:55 What if you use the + (plus operator) and say num + num ? What does that do? You might have thought about this already, that it’s going to concatenate them together. It doesn’t equal 4 , but "2" and "2" , which is 22 . Well, the string 22 , not the number.
01:13 If you took num and you used the * (asterisk), which is the multiplication operator, and you said, okay, multiply that by 5 . Well, that should equal 10 , right? Well, in this case, it’s going to create a string with the number "2" being repeated five times, so you get a string of five 2 s, concatenating them all together.
01:40 When using strings with arithmetic operators, now you can tell that it behaves a little differently than you might have thought. The + concatenates two strings together, as you practiced before, and the * , or the star multiplication operator, creates multiple copies of a string.
02:02 And it doesn’t matter if the number is on the right side of the expression or the left. Say you had 7 * num . It still sees num as a string.
02:14 And 7 is just going to be the number of times that the number that’s inside of num , the string, is concatenated together. You can actually do it with other things, right? You could say 5 * "Hello" .
02:28 One thing that you can’t do is let’s say you said the string of "12" multiplied by the string of "3" . That will give you a TypeError .
02:39 You can’t multiply these two together because it says that you can’t multiply a sequence by a non-int of type str . So the sequence it’s talking about is the string "12" , which is a sequence of two characters, and then trying to multiply it by "3" , which is a non-integer string.
03:02 So this doesn’t work. It’s raising a TypeError . And similarly, what if you took the string of "3" and you tried to add it to the number 3 by itself? That gives another type of TypeError .
03:17 It says you can only concatenate str , not an int , to a str . If an object on either side of the + is a string, then Python tries to perform string concatenation.
03:30 It will only perform addition if both objects are numbers. You would have to first convert the string "3" into a number before you could add it to 3 . Let’s look at how to do that.
03:46 So what do you do with all these strings that you would prefer to be numbers? You’re going to use some built-in functions for that. The function converts objects into whole numbers, or also known as integers.
03:58 Sometimes you’re going to want objects to be turned into numbers that actually have decimal points. In that case, you’ll want to use the built in float() function.
04:08 Let’s try these out. To test this out, head back to IDLE, and you’re going to create a new file. You can use the pull-down menu of File and New , or you can use the key command of Command + N or Control + N on Windows to open up an edit window over here. In the edit window, we’ll create a num object, and it will accept the input from a user. And this is the prompt.
04:39 You’re saying "Enter a number to be doubled: " . Close that out. So the built-in input() function is going to prompt this, and then whatever the value that’s typed in will be assigned to the variable num . Then to create doubled_num , you will say num is multiplied by 2 and then print the output. Great. Go ahead and save that.
05:02 I’ll call it doubled or doubled.py . And again, just save it on my desktop. In this case, if you pressed F5 to run it over here, it’s asking for a number to be doubled, and I will say, oh, 8 , please.
05:17 Oh, you see my error? I created a variable with the wrong name here. I didn’t use the correct name again, so I need to either change it here or change it there.
05:26 So I’m going to change here and save. So, a little NameError issue. All right, now that I’ve saved it, and we can run it again. Okay. This time now I can type 8 .
05:35 Instead of getting 8 times 2 of 16 , I get 88 , so 8 and 8 , because it’s doing that for two strings. So we’ve got to figure out how to change this.
05:48 Open up a new shell window here to kind of clean things up a little bit. You can keep going in yours. I just want to not have so much typing on the bottom.
05:56 So how can you work with this? Well, we were dealing with the number 8 earlier. What you can do, again instead of just working with the number "8" by itself as a string is you can convert a string like "8" into an integer, and you can see int() will convert that into an actual number of the value of 8 here.
06:21 It doesn’t have the quotation marks around it like a string literal. int stands for integer and converts objects into whole numbers, whereas float() is going to convert it into a number with a decimal point.
06:33 So if I were to use float() for "8" , you’ll see 8.0 . Okay, so what do we need to do? Well, what we can do is convert it here and say int() for the number. This is one solution. Let’s try it out.
06:52 So I just saved it again. If I press F5 to run it … oh, this time let’s say "12" . Great. There, 12 multiplied by 2 is 24 .
07:02 But what if I wanted to type in, I don’t know, 2.5 ? Well, then we would need it to be a float, and it wouldn’t be bad if this was 12.0 .
07:15 So maybe it might be safer depending on what we want to type in, a little more flexible, to use float() instead. So I’m going to do that. And if I run it after saving. Now, if I were to, like I said, type 2.5 , it’ll say 5.0 . Or even if I were to run it again and type in 12.0 or even 12 by itself, it will come back as a floating point.
07:39 Why did we use float() instead of int() ? If you give the built- in int() function something that is not in base 10, like if you were to say "2.5" , it gets a little angry.
07:52 It says that’s an invalid literal for int() with this requirement of a base 10. So even if you said "12.0" , you get the same kind of error.
08:04 So float() gives you a lot more flexibility depending on the string that you’re putting into it.
08:11 Sometimes you need to convert a number into a string. To do that reverse, there is a function for it too. Let me give you an example. Let’s say we’re going to do some concatenation again.
08:22 Let’s say we have a variable called the num_pancakes , and currently it’s 10 . You’re super hungry. And you say, "I am going to eat " concatenate the num_pancakes to another string with " pancakes" in it. Automatically print that out and insert the number.
08:45 Well, you can only concatenate a string, not an integer to a string. Since the variable num_pancakes is a number, Python can’t can concatenate it with the other string. You need to convert the num_pancakes integer into a string.
09:02 And that’s where we use the built-in str() function.
09:09 Just like int() and float() , there’s a built-in function, str() , that returns a string version of an object.
09:20 Let me copy this to save a little effort. And here you could add the built-in str() function, which takes an object and it outputs a string. Great.
09:32 Let’s see if that works. Yep. That works great. What’s kind of cool is the built-in str() function can actually handle arithmetic expressions right inside of it.
09:43 So let’s say you had a total_pancakes that was 10 , and these are how many that you’ve eaten so far, which is 5 . So we’ve assigned two variables, total_pancakes to the integer 10 and pancakes_eaten to the integer 5 , and you want to create a string that says "Only " concatenate, and this time you’re converting to a string, and you’re going to say the total_pancakes minus the pancakes that have been eaten, and concatenate that to show the number of pancakes that are left.
10:23 So it can do that subtraction right inside there. Let’s look at some additional exercises for you to practice with these.
10:33 Create a string containing an integer, then convert that string into an actual integer using the built-in int() function. Test that your new object is a number by multiplying it by another number and displaying the result.
10:49 Try out the previous exercise again, but use a floating-point number and the built-in float() function. Try creating a string object and an integer object, then display them side by side with a single print using the str() function. Okay. In this one, you’re going to use input() twice to get two different numbers from a user and then multiply the numbers together and display the result.
11:16 The printout should look something like this.
11:21 Up next, you’re going to learn how to streamline your print() function.
s150042028 on Sept. 16, 2023
Become a Member to join the conversation.
- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
How to extract numbers from a string in Python?
There are several ways to extract numbers from a string in Python. One way to extract numbers from a string is to use regular expressions. Regular expressions are patterns that you can use to match and manipulate strings. Here's an example code snippet that uses regular expressions to extract all the numbers from a string:
Using the re.findall() function
In this example, we're using the re.findall() function to search for all occurrences of numbers in the text string. The regular expression \d+\.\d+|\d+ matches both floating-point numbers and integers.
Using the isdigit() method
Another way to extract numbers from a string is to use the isdigit() method, which returns True if all the characters in a string are digits.
In this example, we're splitting the text string into a list of words using the split() method. We then iterate over each word and check if it's a digit using the isdigit() method. If it is, we append it to the numbers list after converting it to an integer.
Using a regular expression
You can use the re module in Python to extract numbers from a string using regular expressions. Here is an example:
This code will output a list of numbers that appear in the string: [3, 1, 2, 3]. The regular expression '\d+' matches one or more digits in the string.
Using a loop and isdigit() method
You can loop through each character in the string and check if it's a digit using the isdigit() method.
Using split() and isdigit() method
If the numbers are separated by non-digit characters, you can split the string using those characters and then check each resulting substring to see if it's a number.
Using the isnumeric() method and a for loop
This code creates an empty list called numbers, then splits the input string into a list of words. It then loops through each word in the list and checks if it is a number using the isnumeric() method. If it is a number, it is appended to the numbers list. Finally, the numbers list is printed.
This code imports the re module and uses the re.findall() function to find all instances of digits in the input string. The resulting list of digit strings is then converted to a list of integers using a list comprehension. Finally, the numbers list is printed.
Using a generator expression and the map() function:
This code uses a generator expression and the map() function to create a list of integers. The generator expression loops through each word in the input string and only returns the ones that are digits using the isdigit() method. The map() function applies the int() function to each digit string, converting it to an integer. Finally, the resulting list of integers is printed.

- Related Articles
- How to extract numbers from a string using Python?
- Extract decimal numbers from a string in Python \n\n
- How to extract date from a string in Python?
- How to extract numbers from a string using regular expressions?
- How To Extract Decimal Numbers From Text String In Excel?
- How to extract a substring from inside a string in Python?
- Python – Extract Percentages from String
- How to extract data from a string with Python Regular Expressions?
- How to extract numbers from text using Python regular expression?
- How to extract characters from a string in R?
- Extract numbers from list of strings in Python
- Python Regex to extract maximum numeric value from a string
- Python – Extract Rear K digits from Numbers
- How to extract words from a string vector in R?
- How to extract multiple integers from a String in Java?
Kickstart Your Career
Get certified by completing the course
Python Enhancement Proposals
- Python »
- PEP Index »
PEP 572 – Assignment Expressions
The importance of real code, exceptional cases, scope of the target, relative precedence of :=, change to evaluation order, differences between assignment expressions and assignment statements, specification changes during implementation, _pydecimal.py, datetime.py, sysconfig.py, simplifying list comprehensions, capturing condition values, changing the scope rules for comprehensions, alternative spellings, special-casing conditional statements, special-casing comprehensions, lowering operator precedence, allowing commas to the right, always requiring parentheses, why not just turn existing assignment into an expression, with assignment expressions, why bother with assignment statements, why not use a sublocal scope and prevent namespace pollution, style guide recommendations, acknowledgements, a numeric example, appendix b: rough code translations for comprehensions, appendix c: no changes to scope semantics.
This is a proposal for creating a way to assign to variables within an expression using the notation NAME := expr .
As part of this change, there is also an update to dictionary comprehension evaluation order to ensure key expressions are executed before value expressions (allowing the key to be bound to a name and then re-used as part of calculating the corresponding value).
During discussion of this PEP, the operator became informally known as “the walrus operator”. The construct’s formal name is “Assignment Expressions” (as per the PEP title), but they may also be referred to as “Named Expressions” (e.g. the CPython reference implementation uses that name internally).
Naming the result of an expression is an important part of programming, allowing a descriptive name to be used in place of a longer expression, and permitting reuse. Currently, this feature is available only in statement form, making it unavailable in list comprehensions and other expression contexts.
Additionally, naming sub-parts of a large expression can assist an interactive debugger, providing useful display hooks and partial results. Without a way to capture sub-expressions inline, this would require refactoring of the original code; with assignment expressions, this merely requires the insertion of a few name := markers. Removing the need to refactor reduces the likelihood that the code be inadvertently changed as part of debugging (a common cause of Heisenbugs), and is easier to dictate to another programmer.
During the development of this PEP many people (supporters and critics both) have had a tendency to focus on toy examples on the one hand, and on overly complex examples on the other.
The danger of toy examples is twofold: they are often too abstract to make anyone go “ooh, that’s compelling”, and they are easily refuted with “I would never write it that way anyway”.
The danger of overly complex examples is that they provide a convenient strawman for critics of the proposal to shoot down (“that’s obfuscated”).
Yet there is some use for both extremely simple and extremely complex examples: they are helpful to clarify the intended semantics. Therefore, there will be some of each below.
However, in order to be compelling , examples should be rooted in real code, i.e. code that was written without any thought of this PEP, as part of a useful application, however large or small. Tim Peters has been extremely helpful by going over his own personal code repository and picking examples of code he had written that (in his view) would have been clearer if rewritten with (sparing) use of assignment expressions. His conclusion: the current proposal would have allowed a modest but clear improvement in quite a few bits of code.
Another use of real code is to observe indirectly how much value programmers place on compactness. Guido van Rossum searched through a Dropbox code base and discovered some evidence that programmers value writing fewer lines over shorter lines.
Case in point: Guido found several examples where a programmer repeated a subexpression, slowing down the program, in order to save one line of code, e.g. instead of writing:
they would write:
Another example illustrates that programmers sometimes do more work to save an extra level of indentation:
This code tries to match pattern2 even if pattern1 has a match (in which case the match on pattern2 is never used). The more efficient rewrite would have been:
Syntax and semantics
In most contexts where arbitrary Python expressions can be used, a named expression can appear. This is of the form NAME := expr where expr is any valid Python expression other than an unparenthesized tuple, and NAME is an identifier.
The value of such a named expression is the same as the incorporated expression, with the additional side-effect that the target is assigned that value:
There are a few places where assignment expressions are not allowed, in order to avoid ambiguities or user confusion:
This rule is included to simplify the choice for the user between an assignment statement and an assignment expression – there is no syntactic position where both are valid.
Again, this rule is included to avoid two visually similar ways of saying the same thing.
This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
This rule is included to discourage side effects in a position whose exact semantics are already confusing to many users (cf. the common style recommendation against mutable default values), and also to echo the similar prohibition in calls (the previous bullet).
The reasoning here is similar to the two previous cases; this ungrouped assortment of symbols and operators composed of : and = is hard to read correctly.
This allows lambda to always bind less tightly than := ; having a name binding at the top level inside a lambda function is unlikely to be of value, as there is no way to make use of it. In cases where the name will be used more than once, the expression is likely to need parenthesizing anyway, so this prohibition will rarely affect code.
This shows that what looks like an assignment operator in an f-string is not always an assignment operator. The f-string parser uses : to indicate formatting options. To preserve backwards compatibility, assignment operator usage inside of f-strings must be parenthesized. As noted above, this usage of the assignment operator is not recommended.
An assignment expression does not introduce a new scope. In most cases the scope in which the target will be bound is self-explanatory: it is the current scope. If this scope contains a nonlocal or global declaration for the target, the assignment expression honors that. A lambda (being an explicit, if anonymous, function definition) counts as a scope for this purpose.
There is one special case: an assignment expression occurring in a list, set or dict comprehension or in a generator expression (below collectively referred to as “comprehensions”) binds the target in the containing scope, honoring a nonlocal or global declaration for the target in that scope, if one exists. For the purpose of this rule the containing scope of a nested comprehension is the scope that contains the outermost comprehension. A lambda counts as a containing scope.
The motivation for this special case is twofold. First, it allows us to conveniently capture a “witness” for an any() expression, or a counterexample for all() , for example:
Second, it allows a compact way of updating mutable state from a comprehension, for example:
However, an assignment expression target name cannot be the same as a for -target name appearing in any comprehension containing the assignment expression. The latter names are local to the comprehension in which they appear, so it would be contradictory for a contained use of the same name to refer to the scope containing the outermost comprehension instead.
For example, [i := i+1 for i in range(5)] is invalid: the for i part establishes that i is local to the comprehension, but the i := part insists that i is not local to the comprehension. The same reason makes these examples invalid too:
While it’s technically possible to assign consistent semantics to these cases, it’s difficult to determine whether those semantics actually make sense in the absence of real use cases. Accordingly, the reference implementation [1] will ensure that such cases raise SyntaxError , rather than executing with implementation defined behaviour.
This restriction applies even if the assignment expression is never executed:
For the comprehension body (the part before the first “for” keyword) and the filter expression (the part after “if” and before any nested “for”), this restriction applies solely to target names that are also used as iteration variables in the comprehension. Lambda expressions appearing in these positions introduce a new explicit function scope, and hence may use assignment expressions with no additional restrictions.
Due to design constraints in the reference implementation (the symbol table analyser cannot easily detect when names are re-used between the leftmost comprehension iterable expression and the rest of the comprehension), named expressions are disallowed entirely as part of comprehension iterable expressions (the part after each “in”, and before any subsequent “if” or “for” keyword):
A further exception applies when an assignment expression occurs in a comprehension whose containing scope is a class scope. If the rules above were to result in the target being assigned in that class’s scope, the assignment expression is expressly invalid. This case also raises SyntaxError :
(The reason for the latter exception is the implicit function scope created for comprehensions – there is currently no runtime mechanism for a function to refer to a variable in the containing class scope, and we do not want to add such a mechanism. If this issue ever gets resolved this special case may be removed from the specification of assignment expressions. Note that the problem already exists for using a variable defined in the class scope from a comprehension.)
See Appendix B for some examples of how the rules for targets in comprehensions translate to equivalent code.
The := operator groups more tightly than a comma in all syntactic positions where it is legal, but less tightly than all other operators, including or , and , not , and conditional expressions ( A if C else B ). As follows from section “Exceptional cases” above, it is never allowed at the same level as = . In case a different grouping is desired, parentheses should be used.
The := operator may be used directly in a positional function call argument; however it is invalid directly in a keyword argument.
Some examples to clarify what’s technically valid or invalid:
Most of the “valid” examples above are not recommended, since human readers of Python source code who are quickly glancing at some code may miss the distinction. But simple cases are not objectionable:
This PEP recommends always putting spaces around := , similar to PEP 8 ’s recommendation for = when used for assignment, whereas the latter disallows spaces around = used for keyword arguments.)
In order to have precisely defined semantics, the proposal requires evaluation order to be well-defined. This is technically not a new requirement, as function calls may already have side effects. Python already has a rule that subexpressions are generally evaluated from left to right. However, assignment expressions make these side effects more visible, and we propose a single change to the current evaluation order:
- In a dict comprehension {X: Y for ...} , Y is currently evaluated before X . We propose to change this so that X is evaluated before Y . (In a dict display like {X: Y} this is already the case, and also in dict((X, Y) for ...) which should clearly be equivalent to the dict comprehension.)
Most importantly, since := is an expression, it can be used in contexts where statements are illegal, including lambda functions and comprehensions.
Conversely, assignment expressions don’t support the advanced features found in assignment statements:
- Multiple targets are not directly supported: x = y = z = 0 # Equivalent: (z := (y := (x := 0)))
- Single assignment targets other than a single NAME are not supported: # No equivalent a [ i ] = x self . rest = []
- Priority around commas is different: x = 1 , 2 # Sets x to (1, 2) ( x := 1 , 2 ) # Sets x to 1
- Iterable packing and unpacking (both regular or extended forms) are not supported: # Equivalent needs extra parentheses loc = x , y # Use (loc := (x, y)) info = name , phone , * rest # Use (info := (name, phone, *rest)) # No equivalent px , py , pz = position name , phone , email , * other_info = contact
- Inline type annotations are not supported: # Closest equivalent is "p: Optional[int]" as a separate declaration p : Optional [ int ] = None
- Augmented assignment is not supported: total += tax # Equivalent: (total := total + tax)
The following changes have been made based on implementation experience and additional review after the PEP was first accepted and before Python 3.8 was released:
- for consistency with other similar exceptions, and to avoid locking in an exception name that is not necessarily going to improve clarity for end users, the originally proposed TargetScopeError subclass of SyntaxError was dropped in favour of just raising SyntaxError directly. [3]
- due to a limitation in CPython’s symbol table analysis process, the reference implementation raises SyntaxError for all uses of named expressions inside comprehension iterable expressions, rather than only raising them when the named expression target conflicts with one of the iteration variables in the comprehension. This could be revisited given sufficiently compelling examples, but the extra complexity needed to implement the more selective restriction doesn’t seem worthwhile for purely hypothetical use cases.
Examples from the Python standard library
env_base is only used on these lines, putting its assignment on the if moves it as the “header” of the block.
- Current: env_base = os . environ . get ( "PYTHONUSERBASE" , None ) if env_base : return env_base
- Improved: if env_base := os . environ . get ( "PYTHONUSERBASE" , None ): return env_base
Avoid nested if and remove one indentation level.
- Current: if self . _is_special : ans = self . _check_nans ( context = context ) if ans : return ans
- Improved: if self . _is_special and ( ans := self . _check_nans ( context = context )): return ans
Code looks more regular and avoid multiple nested if. (See Appendix A for the origin of this example.)
- Current: reductor = dispatch_table . get ( cls ) if reductor : rv = reductor ( x ) else : reductor = getattr ( x , "__reduce_ex__" , None ) if reductor : rv = reductor ( 4 ) else : reductor = getattr ( x , "__reduce__" , None ) if reductor : rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
- Improved: if reductor := dispatch_table . get ( cls ): rv = reductor ( x ) elif reductor := getattr ( x , "__reduce_ex__" , None ): rv = reductor ( 4 ) elif reductor := getattr ( x , "__reduce__" , None ): rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
tz is only used for s += tz , moving its assignment inside the if helps to show its scope.
- Current: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) tz = self . _tzstr () if tz : s += tz return s
- Improved: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) if tz := self . _tzstr (): s += tz return s
Calling fp.readline() in the while condition and calling .match() on the if lines make the code more compact without making it harder to understand.
- Current: while True : line = fp . readline () if not line : break m = define_rx . match ( line ) if m : n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v else : m = undef_rx . match ( line ) if m : vars [ m . group ( 1 )] = 0
- Improved: while line := fp . readline (): if m := define_rx . match ( line ): n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v elif m := undef_rx . match ( line ): vars [ m . group ( 1 )] = 0
A list comprehension can map and filter efficiently by capturing the condition:
Similarly, a subexpression can be reused within the main expression, by giving it a name on first use:
Note that in both cases the variable y is bound in the containing scope (i.e. at the same level as results or stuff ).
Assignment expressions can be used to good effect in the header of an if or while statement:
Particularly with the while loop, this can remove the need to have an infinite loop, an assignment, and a condition. It also creates a smooth parallel between a loop which simply uses a function call as its condition, and one which uses that as its condition but also uses the actual value.
An example from the low-level UNIX world:
Rejected alternative proposals
Proposals broadly similar to this one have come up frequently on python-ideas. Below are a number of alternative syntaxes, some of them specific to comprehensions, which have been rejected in favour of the one given above.
A previous version of this PEP proposed subtle changes to the scope rules for comprehensions, to make them more usable in class scope and to unify the scope of the “outermost iterable” and the rest of the comprehension. However, this part of the proposal would have caused backwards incompatibilities, and has been withdrawn so the PEP can focus on assignment expressions.
Broadly the same semantics as the current proposal, but spelled differently.
Since EXPR as NAME already has meaning in import , except and with statements (with different semantics), this would create unnecessary confusion or require special-casing (e.g. to forbid assignment within the headers of these statements).
(Note that with EXPR as VAR does not simply assign the value of EXPR to VAR – it calls EXPR.__enter__() and assigns the result of that to VAR .)
Additional reasons to prefer := over this spelling include:
- In if f(x) as y the assignment target doesn’t jump out at you – it just reads like if f x blah blah and it is too similar visually to if f(x) and y .
- import foo as bar
- except Exc as var
- with ctxmgr() as var
To the contrary, the assignment expression does not belong to the if or while that starts the line, and we intentionally allow assignment expressions in other contexts as well.
- NAME = EXPR
- if NAME := EXPR
reinforces the visual recognition of assignment expressions.
This syntax is inspired by languages such as R and Haskell, and some programmable calculators. (Note that a left-facing arrow y <- f(x) is not possible in Python, as it would be interpreted as less-than and unary minus.) This syntax has a slight advantage over ‘as’ in that it does not conflict with with , except and import , but otherwise is equivalent. But it is entirely unrelated to Python’s other use of -> (function return type annotations), and compared to := (which dates back to Algol-58) it has a much weaker tradition.
This has the advantage that leaked usage can be readily detected, removing some forms of syntactic ambiguity. However, this would be the only place in Python where a variable’s scope is encoded into its name, making refactoring harder.
Execution order is inverted (the indented body is performed first, followed by the “header”). This requires a new keyword, unless an existing keyword is repurposed (most likely with: ). See PEP 3150 for prior discussion on this subject (with the proposed keyword being given: ).
This syntax has fewer conflicts than as does (conflicting only with the raise Exc from Exc notation), but is otherwise comparable to it. Instead of paralleling with expr as target: (which can be useful but can also be confusing), this has no parallels, but is evocative.
One of the most popular use-cases is if and while statements. Instead of a more general solution, this proposal enhances the syntax of these two statements to add a means of capturing the compared value:
This works beautifully if and ONLY if the desired condition is based on the truthiness of the captured value. It is thus effective for specific use-cases (regex matches, socket reads that return '' when done), and completely useless in more complicated cases (e.g. where the condition is f(x) < 0 and you want to capture the value of f(x) ). It also has no benefit to list comprehensions.
Advantages: No syntactic ambiguities. Disadvantages: Answers only a fraction of possible use-cases, even in if / while statements.
Another common use-case is comprehensions (list/set/dict, and genexps). As above, proposals have been made for comprehension-specific solutions.
This brings the subexpression to a location in between the ‘for’ loop and the expression. It introduces an additional language keyword, which creates conflicts. Of the three, where reads the most cleanly, but also has the greatest potential for conflict (e.g. SQLAlchemy and numpy have where methods, as does tkinter.dnd.Icon in the standard library).
As above, but reusing the with keyword. Doesn’t read too badly, and needs no additional language keyword. Is restricted to comprehensions, though, and cannot as easily be transformed into “longhand” for-loop syntax. Has the C problem that an equals sign in an expression can now create a name binding, rather than performing a comparison. Would raise the question of why “with NAME = EXPR:” cannot be used as a statement on its own.
As per option 2, but using as rather than an equals sign. Aligns syntactically with other uses of as for name binding, but a simple transformation to for-loop longhand would create drastically different semantics; the meaning of with inside a comprehension would be completely different from the meaning as a stand-alone statement, while retaining identical syntax.
Regardless of the spelling chosen, this introduces a stark difference between comprehensions and the equivalent unrolled long-hand form of the loop. It is no longer possible to unwrap the loop into statement form without reworking any name bindings. The only keyword that can be repurposed to this task is with , thus giving it sneakily different semantics in a comprehension than in a statement; alternatively, a new keyword is needed, with all the costs therein.
There are two logical precedences for the := operator. Either it should bind as loosely as possible, as does statement-assignment; or it should bind more tightly than comparison operators. Placing its precedence between the comparison and arithmetic operators (to be precise: just lower than bitwise OR) allows most uses inside while and if conditions to be spelled without parentheses, as it is most likely that you wish to capture the value of something, then perform a comparison on it:
Once find() returns -1, the loop terminates. If := binds as loosely as = does, this would capture the result of the comparison (generally either True or False ), which is less useful.
While this behaviour would be convenient in many situations, it is also harder to explain than “the := operator behaves just like the assignment statement”, and as such, the precedence for := has been made as close as possible to that of = (with the exception that it binds tighter than comma).
Some critics have claimed that the assignment expressions should allow unparenthesized tuples on the right, so that these two would be equivalent:
(With the current version of the proposal, the latter would be equivalent to ((point := x), y) .)
However, adopting this stance would logically lead to the conclusion that when used in a function call, assignment expressions also bind less tight than comma, so we’d have the following confusing equivalence:
The less confusing option is to make := bind more tightly than comma.
It’s been proposed to just always require parentheses around an assignment expression. This would resolve many ambiguities, and indeed parentheses will frequently be needed to extract the desired subexpression. But in the following cases the extra parentheses feel redundant:
Frequently Raised Objections
C and its derivatives define the = operator as an expression, rather than a statement as is Python’s way. This allows assignments in more contexts, including contexts where comparisons are more common. The syntactic similarity between if (x == y) and if (x = y) belies their drastically different semantics. Thus this proposal uses := to clarify the distinction.
The two forms have different flexibilities. The := operator can be used inside a larger expression; the = statement can be augmented to += and its friends, can be chained, and can assign to attributes and subscripts.
Previous revisions of this proposal involved sublocal scope (restricted to a single statement), preventing name leakage and namespace pollution. While a definite advantage in a number of situations, this increases complexity in many others, and the costs are not justified by the benefits. In the interests of language simplicity, the name bindings created here are exactly equivalent to any other name bindings, including that usage at class or module scope will create externally-visible names. This is no different from for loops or other constructs, and can be solved the same way: del the name once it is no longer needed, or prefix it with an underscore.
(The author wishes to thank Guido van Rossum and Christoph Groth for their suggestions to move the proposal in this direction. [2] )
As expression assignments can sometimes be used equivalently to statement assignments, the question of which should be preferred will arise. For the benefit of style guides such as PEP 8 , two recommendations are suggested.
- If either assignment statements or assignment expressions can be used, prefer statements; they are a clear declaration of intent.
- If using assignment expressions would lead to ambiguity about execution order, restructure it to use statements instead.
The authors wish to thank Alyssa Coghlan and Steven D’Aprano for their considerable contributions to this proposal, and members of the core-mentorship mailing list for assistance with implementation.
Appendix A: Tim Peters’s findings
Here’s a brief essay Tim Peters wrote on the topic.
I dislike “busy” lines of code, and also dislike putting conceptually unrelated logic on a single line. So, for example, instead of:
instead. So I suspected I’d find few places I’d want to use assignment expressions. I didn’t even consider them for lines already stretching halfway across the screen. In other cases, “unrelated” ruled:
is a vast improvement over the briefer:
The original two statements are doing entirely different conceptual things, and slamming them together is conceptually insane.
In other cases, combining related logic made it harder to understand, such as rewriting:
as the briefer:
The while test there is too subtle, crucially relying on strict left-to-right evaluation in a non-short-circuiting or method-chaining context. My brain isn’t wired that way.
But cases like that were rare. Name binding is very frequent, and “sparse is better than dense” does not mean “almost empty is better than sparse”. For example, I have many functions that return None or 0 to communicate “I have nothing useful to return in this case, but since that’s expected often I’m not going to annoy you with an exception”. This is essentially the same as regular expression search functions returning None when there is no match. So there was lots of code of the form:
I find that clearer, and certainly a bit less typing and pattern-matching reading, as:
It’s also nice to trade away a small amount of horizontal whitespace to get another _line_ of surrounding code on screen. I didn’t give much weight to this at first, but it was so very frequent it added up, and I soon enough became annoyed that I couldn’t actually run the briefer code. That surprised me!
There are other cases where assignment expressions really shine. Rather than pick another from my code, Kirill Balunov gave a lovely example from the standard library’s copy() function in copy.py :
The ever-increasing indentation is semantically misleading: the logic is conceptually flat, “the first test that succeeds wins”:
Using easy assignment expressions allows the visual structure of the code to emphasize the conceptual flatness of the logic; ever-increasing indentation obscured it.
A smaller example from my code delighted me, both allowing to put inherently related logic in a single line, and allowing to remove an annoying “artificial” indentation level:
That if is about as long as I want my lines to get, but remains easy to follow.
So, in all, in most lines binding a name, I wouldn’t use assignment expressions, but because that construct is so very frequent, that leaves many places I would. In most of the latter, I found a small win that adds up due to how often it occurs, and in the rest I found a moderate to major win. I’d certainly use it more often than ternary if , but significantly less often than augmented assignment.
I have another example that quite impressed me at the time.
Where all variables are positive integers, and a is at least as large as the n’th root of x, this algorithm returns the floor of the n’th root of x (and roughly doubling the number of accurate bits per iteration):
It’s not obvious why that works, but is no more obvious in the “loop and a half” form. It’s hard to prove correctness without building on the right insight (the “arithmetic mean - geometric mean inequality”), and knowing some non-trivial things about how nested floor functions behave. That is, the challenges are in the math, not really in the coding.
If you do know all that, then the assignment-expression form is easily read as “while the current guess is too large, get a smaller guess”, where the “too large?” test and the new guess share an expensive sub-expression.
To my eyes, the original form is harder to understand:
This appendix attempts to clarify (though not specify) the rules when a target occurs in a comprehension or in a generator expression. For a number of illustrative examples we show the original code, containing a comprehension, and the translation, where the comprehension has been replaced by an equivalent generator function plus some scaffolding.
Since [x for ...] is equivalent to list(x for ...) these examples all use list comprehensions without loss of generality. And since these examples are meant to clarify edge cases of the rules, they aren’t trying to look like real code.
Note: comprehensions are already implemented via synthesizing nested generator functions like those in this appendix. The new part is adding appropriate declarations to establish the intended scope of assignment expression targets (the same scope they resolve to as if the assignment were performed in the block containing the outermost comprehension). For type inference purposes, these illustrative expansions do not imply that assignment expression targets are always Optional (but they do indicate the target binding scope).
Let’s start with a reminder of what code is generated for a generator expression without assignment expression.
- Original code (EXPR usually references VAR): def f (): a = [ EXPR for VAR in ITERABLE ]
- Translation (let’s not worry about name conflicts): def f (): def genexpr ( iterator ): for VAR in iterator : yield EXPR a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a simple assignment expression.
- Original code: def f (): a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): if False : TARGET = None # Dead code to ensure TARGET is a local variable def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a global TARGET declaration in f() .
- Original code: def f (): global TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): global TARGET def genexpr ( iterator ): global TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Or instead let’s add a nonlocal TARGET declaration in f() .
- Original code: def g (): TARGET = ... def f (): nonlocal TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def g (): TARGET = ... def f (): nonlocal TARGET def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Finally, let’s nest two comprehensions.
- Original code: def f (): a = [[ TARGET := i for i in range ( 3 )] for j in range ( 2 )] # I.e., a = [[0, 1, 2], [0, 1, 2]] print ( TARGET ) # prints 2
- Translation: def f (): if False : TARGET = None def outer_genexpr ( outer_iterator ): nonlocal TARGET def inner_generator ( inner_iterator ): nonlocal TARGET for i in inner_iterator : TARGET = i yield i for j in outer_iterator : yield list ( inner_generator ( range ( 3 ))) a = list ( outer_genexpr ( range ( 2 ))) print ( TARGET )
Because it has been a point of confusion, note that nothing about Python’s scoping semantics is changed. Function-local scopes continue to be resolved at compile time, and to have indefinite temporal extent at run time (“full closures”). Example:
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-0572.rst
Last modified: 2023-10-11 12:05:51 GMT
Python Programming
Practice Python Exercises and Challenges with Solutions
Free Coding Exercises for Python Developers. Exercises cover Python Basics , Data structure , to Data analytics . As of now, this page contains 18 Exercises.
What included in these Python Exercises?
Each exercise contains specific Python topic questions you need to practice and solve. These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges.
- All exercises are tested on Python 3.
- Each exercise has 10-20 Questions.
- The solution is provided for every question.
- Practice each Exercise in Online Code Editor
These Python programming exercises are suitable for all Python developers. If you are a beginner, you will have a better understanding of Python after solving these exercises. Below is the list of exercises.
Select the exercise you want to solve .
Basic Exercise for Beginners
Practice and Quickly learn Python’s necessary skills by solving simple questions and problems.
Topics : Variables, Operators, Loops, String, Numbers, List
Python Input and Output Exercise
Solve input and output operations in Python. Also, we practice file handling.
Topics : print() and input() , File I/O
Python Loop Exercise
This Python loop exercise aims to help developers to practice branching and Looping techniques in Python.
Topics : If-else statements, loop, and while loop.
Python Functions Exercise
Practice how to create a function, nested functions, and use the function arguments effectively in Python by solving different questions.
Topics : Functions arguments, built-in functions.
Python String Exercise
Solve Python String exercise to learn and practice String operations and manipulations.
Python Data Structure Exercise
Practice widely used Python types such as List, Set, Dictionary, and Tuple operations in Python
Python List Exercise
This Python list exercise aims to help Python developers to learn and practice list operations.

Python Dictionary Exercise
This Python dictionary exercise aims to help Python developers to learn and practice dictionary operations.
Python Set Exercise
This exercise aims to help Python developers to learn and practice set operations.
Python Tuple Exercise
This exercise aims to help Python developers to learn and practice tuple operations.
Python Date and Time Exercise
This exercise aims to help Python developers to learn and practice DateTime and timestamp questions and problems.
Topics : Date, time, DateTime, Calendar.
Python OOP Exercise
This Python Object-oriented programming (OOP) exercise aims to help Python developers to learn and practice OOP concepts.
Topics : Object, Classes, Inheritance
Python JSON Exercise
Practice and Learn JSON creation, manipulation, Encoding, Decoding, and parsing using Python
Python NumPy Exercise
Practice NumPy questions such as Array manipulations, numeric ranges, Slicing, indexing, Searching, Sorting, and splitting, and more.
Python Pandas Exercise
Practice Data Analysis using Python Pandas. Practice Data-frame, Data selection, group-by, Series, sorting, searching, and statistics.
Python Matplotlib Exercise
Practice Data visualization using Python Matplotlib. Line plot, Style properties, multi-line plot, scatter plot, bar chart, histogram, Pie chart, Subplot, stack plot.
Random Data Generation Exercise
Practice and Learn the various techniques to generate random data in Python.
Topics : random module, secrets module, UUID module
Python Database Exercise
Practice Python database programming skills by solving the questions step by step.
Use any of the MySQL, PostgreSQL, SQLite to solve the exercise
Exercises for Intermediate developers
The following practice questions are for intermediate Python developers.
If you have not solved the above exercises, please complete them to understand and practice each topic in detail. After that, you can solve the below questions quickly.
Exercise 1: Reverse each word of a string
Expected Output
- Use the split() method to split a string into a list of words.
- Reverse each word from a list
- finally, use the join() function to convert a list into a string
Steps to solve this question :
- Split the given string into a list of words using the split() method
- Use a list comprehension to create a new list by reversing each word from a list.
- Use the join() function to convert the new list into a string
- Display the resultant string
Exercise 2: Read text file into a variable and replace all newlines with space
Given : Assume you have a following text file (sample.txt).
Expected Output :
- First, read a text file.
- Next, use string replace() function to replace all newlines ( \n ) with space ( ' ' ).
Steps to solve this question : -
- First, open the file in a read mode
- Next, read all content from a file using the read() function and assign it to a variable.
- Display final string
Exercise 3: Remove items from a list while iterating
Description :
In this question, You need to remove items from a list while iterating but without creating a different copy of a list.
Remove numbers greater than 50
Expected Output : -
- Get the list's size
- Iterate list using while loop
- Check if the number is greater than 50
- If yes, delete the item using a del keyword
- Reduce the list size
Solution 1: Using while loop
Solution 2: Using for loop and range()
Exercise 4: Reverse Dictionary mapping
Exercise 5: display all duplicate items from a list.
- Use the counter() method of the collection module.
- Create a dictionary that will maintain the count of each item of a list. Next, Fetch all keys whose value is greater than 2
Solution 1 : - Using collections.Counter()
Solution 2 : -
Exercise 6: Filter dictionary to contain keys present in the given list
Exercise 7: print the following number pattern.
Refer to Print patterns in Python to solve this question.
- Use two for loops
- The outer loop is reverse for loop from 5 to 0
- Increment value of x by 1 in each iteration of an outer loop
- The inner loop will iterate from 0 to the value of i of the outer loop
- Print value of x in each iteration of an inner loop
- Print newline at the end of each outer loop
Exercise 8: Create an inner function
Question description : -
- Create an outer function that will accept two strings, x and y . ( x= 'Emma' and y = 'Kelly' .
- Create an inner function inside an outer function that will concatenate x and y.
- At last, an outer function will join the word 'developer' to it.
Exercise 9: Modify the element of a nested list inside the following list
Change the element 35 to 3500
Exercise 10: Access the nested key increment from the following dictionary
Under Exercises: -
Python Object-Oriented Programming (OOP) Exercise: Classes and Objects Exercises
Updated on: December 8, 2021 | 52 Comments
Python Date and Time Exercise with Solutions
Updated on: December 8, 2021 | 10 Comments
Python Dictionary Exercise with Solutions
Updated on: May 6, 2023 | 56 Comments
Python Tuple Exercise with Solutions
Updated on: December 8, 2021 | 96 Comments
Python Set Exercise with Solutions
Updated on: October 20, 2022 | 27 Comments
Python if else, for loop, and range() Exercises with Solutions
Updated on: July 6, 2024 | 296 Comments
Updated on: August 2, 2022 | 155 Comments
Updated on: September 6, 2021 | 109 Comments
Python List Exercise with Solutions
Updated on: December 8, 2021 | 200 Comments
Updated on: December 8, 2021 | 7 Comments
Python Data Structure Exercise for Beginners
Updated on: December 8, 2021 | 116 Comments
Python String Exercise with Solutions
Updated on: October 6, 2021 | 221 Comments
Updated on: March 9, 2021 | 23 Comments
Updated on: March 9, 2021 | 51 Comments
Updated on: July 20, 2021 | 29 Comments
Python Basic Exercise for Beginners
Updated on: August 31, 2023 | 495 Comments
Useful Python Tips and Tricks Every Programmer Should Know
Updated on: May 17, 2021 | 23 Comments
Python random Data generation Exercise
Updated on: December 8, 2021 | 13 Comments
Python Database Programming Exercise
Updated on: March 9, 2021 | 17 Comments
- Online Python Code Editor
Updated on: June 1, 2022 |
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
- Docs »
- Lesson notes »
- Lesson 1 - Numbers, Strings
- Edit on GitHub
Lesson 1 - Numbers, Strings ¶
Welcome to the very first lesson on Python. I am sure you are going to have a very nice time learning to program.
Python is a very popular programming language that is used in a wide variety of applications, games, and in building web sites. In this course, we will only focus on the very basic features of the language as the main emphasis here is not to master the language but rather to understand general programming concepts. Keep in mind that many things you learn here apply to other programming languages as well.
Data Types ¶
When you start learning any programming language, the very first thing you should do is to understand what types of data that it can work with and what kind of operations that it supports on them. This is generally referred to as types or data types . The most basic data types that all languages support are numbers and strings so we will start with those.
Python supports all kinds of numbers that you know from your Maths class.
Python supports all the usual arithmetic operations on numbers so you can use it as a calculator:
BTW, did you notice how you can write comments in the code using # symbol? Any text following this character is ignored by Python so you can use it to write comments in your code (to explain what your code is doing if it is not already clear).
Let us now move to “strings”. You will need to manipulate strings in many programs so it is very useful to have good knowledge about them. A string is a sequence of characters. A single character is also a string which has a length of 1. In Python, strings are enclosed in either double quotes or single quotes.
Python supports several operations on Strings. Here are some of them.:
You can combine two strings using + operator.:
Note that the first string contains a “space” which is no different from any other character.
print() function ¶
You can print various values using the function print() . You can either print single values or a combination of them.:
Notice how Python is adding a “space” between items that are passed to “print”.
As you have already seen, you use a function by passing it some values in parenthesis. However, passing values is not mandatory (but you still need parenthesis). Here is an example:
Python has many other functions which we will learn in later lessons.
Variables ¶
Many times in your program, you need to store values before using them later. For this, you use “variables”. Here is an example:
Here, we could have used “42” directly but instead, we created a variable called “age” which now contains the value “42”. You can now use “age” to mean 42 at any place in the code.
= is known as assignment operator which assigns values from right side to variables on the left side.
You can choose any name you want for variables (subject to some rules) but it is very important that you name them appropriately. In particular, variables should be named such that they describe the values they may contain. . This helps you and others in understanding the code, especially when you are reading it at a later time.
Apart from naming variables descriptively, you should not use Python function names to name your variables.
Formatting strings ¶
You can combine strings and integers and even other data types in any format you want to form a new string. This is especially useful if you want to print some information to the console.
Here is an example:
Here, {} are just place holders. They will be replaced by the values you pass to format() function.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Rearranging numbers in a string
Rearrange Numbers in String
Given a string, write a program to re-arrange all the numbers appearing in the string in decreasing order. Note: There will not be any negative numbers or numbers with decimal part.
The input will be a single line containing a string.
The output should be a single line containing the modified string with all the numbers in string re-ordered in decreasing order.
Explanation:
For example, if the given string is "I am 5 years and 11 months old", the numbers are 5, 11. Your code should print the sentence after re-ordering the numbers as "I am 11 years and 5 months old".
My approach:
But there should be space between 28months
- substitution

- 3 Nice first post, thanks for taking your time to fix the errors. – Patrick Artner Commented Jul 9, 2021 at 11:44
6 Answers 6
You do a lot of string manipulation on a character by character basis in your code. Finding numbers digit-wise is a complicated way to do what you must do. Your lost space is caused by your approach:
Hint: check the length's of your numbers in your text - you may not always replace a 1-digit number by another 1-digit number - sometimes you would need to replace a 1-digit number by a 3 digit number:
and in that case your "char-by-char" replacing will get wonky and you loose the space.
Essentially you replace "2 " by a 2-digit number (or "12 " by a 3-digit one etc. killing the space).
It would be better to
- detect all numbers
- sort detected numbers by their integer value descending
- replace all detected numbers in your text with format-placeholder '{}'
- use string.formt() to substitute numbers in correct order
- Your clarification is Upto Mark. Thank you for elaborating @Patrick Artner – OLD Soul Commented Jul 11, 2021 at 11:45
You could use a regular expression to find all numbers:

You can try this:
Sample run.
You can use string.split() and list comprehension. To populate the new string you can transform the sorted list of numbers in an iterator:
You can use re.sub and re.findall :

this code is lengthy and only for beginner those who just started coding and stuck at some point and unable to solve the problem but need to complete it.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python string numbers substitution or ask your own question .
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- What is the translation of point man in French?
- Did the United States have consent from Texas to cede a piece of land that was part of Texas?
- What is the origin and meaning of the phrase “wear the brown helmet”?
- If I purchase a house through an installment sale, can I use it as collateral for a loan?
- Is It Possible to Assign Meaningful Amplitudes to Properties in Quantum Mechanics?
- How to fix a bottom bracket shell that has been cut from outside?
- Uneven Spacing with Consecutive Math Environments
- Where will the ants position themselves so that they are precisely twice as far from vinegar as they are from peanut butter?
- MOSFETs keep shorting way below rated current
- Solve an integral analytically
- Guitar amplifier placement for live band
- Can I travel with regional trains from operators other than DB if I can "use any train" due to a schedule change?
- Why did R Yochanan say 'We' when he Refuted Reish Lakish on his own?
- How can I prove both series are equal?
- Has technology regressed in the Alien universe?
- Where exactly was this picture taken?
- How predictable are the voting records of members of the US legislative branch?
- Why in QM the solution to Laguerre equations are ONLY Laguerre polynomials?
- What's wrong with my app authentication scheme?
- Finding a Linear Algebra reading topic
- How to allow just one user to use SSH?
- Does H3PO exist?
- Ancestry & FTM- unknown names
- how to label a tikz path, where the node label extends outward perpendicular to the path at a point
CopyAssignment
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs.
String modification in Python
Problem statement:.
We are given a string containing words and numbers(integers). We need to arrange the sort numbers in decreasing order. For example, converting CopyAssignment is 2 years, 5 months, and 2 days old now to CopyAssignment is 5 years, 2 months, and 2 days old now .
Code for String modification in Python:

- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python
Author: Harry

Search….
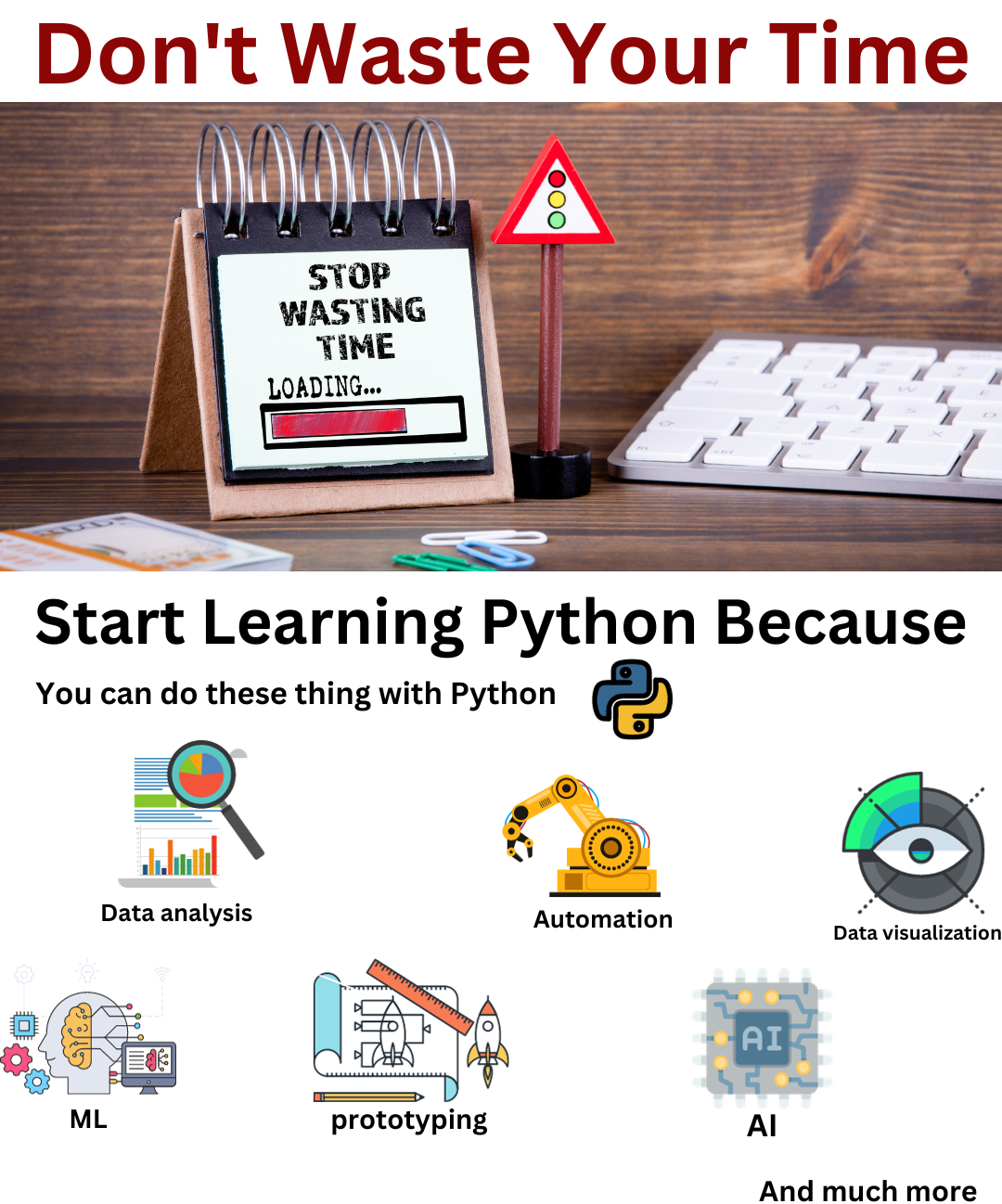
Machine Learning
Data Structures and Algorithms(Python)
Python Turtle
Games with Python
All Blogs On-Site
Python Compiler(Interpreter)
Online Java Editor
Online C++ Editor
Online C Editor
All Editors
Services(Freelancing)
Recent Posts
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
© Copyright 2019-2024 www.copyassignment.com. All rights reserved. Developed by copyassignment
- How it works
- Homework answers
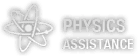
Answer to Question #171149 in Python for Radhika
Numbers in String - 2
Given a string, write a program to return the sum and average of the numbers that appear in the string, ignoring all other characters.Input
The input will be a single line containing a string.Output
The output should contain the sum and average of the numbers that appear in the string.
Note: Round the average value to two decimal places.Explanation
For example, if the given string is "I am 25 years and 10 months old", the numbers are 25, 10. Your code should print the sum of the numbers(35) and the average of the numbers(17.5) in the new line.
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
i want sum and average for this code. you give only sum.
Leave a comment
Ask your question, related questions.
- 1. You are given a square matrix A of dimensions NxN. You need to apply the below given 3 operations on
- 2. Explore the dependence of the period of a pendulum's motion as a function of its initial amplit
- 3. Using array-like vector of unknowns for the ode equation at time t, classes, and the integrate.odein
- 4. After your program has prompted the user for how many values should be in the array, generated those
- 5. Task Description You are to present your findings in the form of a problem based report. This repor
- 6. Time ConverterIn this assignment, let's build a Time Converter by applying the concepts we lear
- 7. Given product code, name, and price for n=7 products as shown in the code snippetbelow.prod = [11,
- Programming
- Engineering
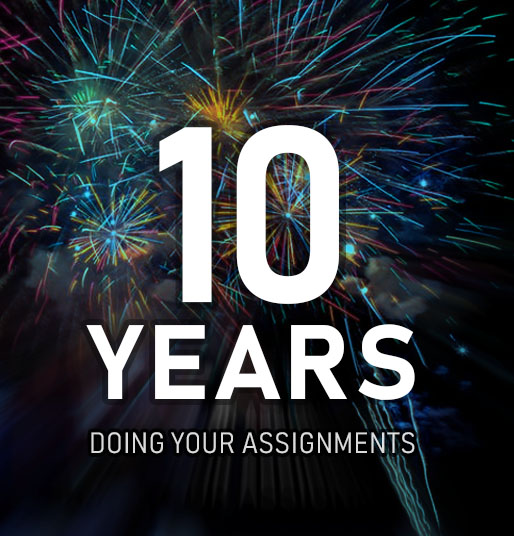

IMAGES
COMMENTS
Numbers in string-1. Given a string, write a program to return the sum and average of the digits of all numbers that appear in the string, ignoring all other characters. Sample Input 1. Anjali25 is python4 Expert. Sample output 1. 11. 3.67. Sample input 2. Tech Foundation 35567. Sample output 2. 26. 5.2
Question #174135. Numbers in String - 1. Given a string, write a program to return the sum and average of the digits of all numbers that appear in the string, ignoring all other characters.Input. The input will be a single line containing a string.Output. The output should contain the sum and average of the digits of all numbers that appear in ...
Get homework answers from experts in Python. Submit your question, choose a relevant category and get a detailed answer for free.
339 Most of the questions I've found are biased on the fact they're looking for letters in their numbers, whereas I'm looking for numbers in what I'd like to be a numberless string. I need to enter a string and check to see if it contains any numbers and if it does reject it.
This Python String exercise project is to help you to learn and practice String operations. All 18 string programs are tested on Python 3.
Extract numbers from string using list comprehension and isdigit () method This problem can be solved by using the split function to convert string to list and then the list comprehension which can help us iterate through the list and isdigit function helps to get the digit out of a string.
Variables and Assignment When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable ...
Python Strings. In Python, a string is a sequence of characters. For example, "hello" is a string containing a sequence of characters 'h', 'e', 'l', 'l', and 'o'. We use single quotes or double quotes to represent a string in Python. For example, # create a string using double quotes. string1 = "Python programming" # create a string using ...
In this tutorial, you'll learn how to use Python's assignment operators to write assignment statements that allow you to create, initialize, and update variables in your code.
Answer to Question #199844 in Python for vinay. Given a string, write a program to return the sum and average of the digits of all numbers that appear in the string, ignoring all other characters.Input. The input will be a single line containing a string.Output. The output should contain the sum and average of the digits of all numbers that ...
Working With Strings and Numbers. 00:00 Now that you've worked with user input a bit, let's talk about working with strings and numbers. 00:07 When you get user input using the built-in input() function, the result is always a string. There are many other situations in which input is given to a program as a string.
There are several ways to extract numbers from a string in Python. One way to extract numbers from a string is to use regular expressions. Regular expressions are patterns that you can use to match and manipulate strings. Here's an example code snippet that uses regular expressions to extract all the numbers from a string:
For example, if the given string is "I am 25 years and 10 months old", the digits of all numbers that appear in the string are 2, 5, 1, 0. Your code should print the sum of all digits( 8) and the average of all digits( 2.0) in the new line .
Assigning integers to strings Asked 11 years, 6 months ago Modified 11 years, 6 months ago Viewed 14k times
This shows that what looks like an assignment operator in an f-string is not always an assignment operator. The f-string parser uses : to indicate formatting options.
Practice Python Exercises and Challenges with Solutions Free Coding Exercises for Python Developers. Exercises cover Python Basics, Data structure, to Data analytics. As of now, this page contains 18 Exercises.
The most basic data types that all languages support are numbers and strings so we will start with those. Python supports all kinds of numbers that you know from your Maths class. >>> 42 42 >>> 10.0 10.0. Python supports all the usual arithmetic operations on numbers so you can use it as a calculator: >>> 42 + 10 52 >>> 42 * 10 420 >>> 42/2 21. ...
Output The output should be a single line containing the modified string with all the numbers in string re-ordered in decreasing order. Explanation: For example, if the given string is "I am 5 years and 11 months old", the numbers are 5, 11. Your code should print the sentence after re-ordering the numbers as "I am 11 years and 5 months old".
Question #224341. Numbers in String - 2. Given a string, write a program to return the sum and average of the numbers that appear in the string, ignoring all other characters.Input. The input will be a single line containing a string.Output. The output should contain the sum and average of the numbers that appear in the string.
Problem Statement: We are given a string containing words and numbers (integers). We need to arrange the sort numbers in decreasing order. For example, converting CopyAssignment is 2 years, 5 months, and 2 days old now to CopyAssignment is 5 years, 2 months, and 2 days old now.
Answer to Question #171149 in Python for Radhika. Numbers in String - 2. Given a string, write a program to return the sum and average of the numbers that appear in the string, ignoring all other characters.Input. The input will be a single line containing a string.Output. The output should contain the sum and average of the numbers that appear ...