35 Python Programming Exercises and Solutions
To understand a programming language deeply, you need to practice what you’ve learned. If you’ve completed learning the syntax of Python programming language, it is the right time to do some practice programs.

1. Python program to check whether the given number is even or not.
2. python program to convert the temperature in degree centigrade to fahrenheit, 3. python program to find the area of a triangle whose sides are given, 4. python program to find out the average of a set of integers, 5. python program to find the product of a set of real numbers, 6. python program to find the circumference and area of a circle with a given radius, 7. python program to check whether the given integer is a multiple of 5, 8. python program to check whether the given integer is a multiple of both 5 and 7, 9. python program to find the average of 10 numbers using while loop, 10. python program to display the given integer in a reverse manner, 11. python program to find the geometric mean of n numbers, 12. python program to find the sum of the digits of an integer using a while loop, 13. python program to display all the multiples of 3 within the range 10 to 50, 14. python program to display all integers within the range 100-200 whose sum of digits is an even number, 15. python program to check whether the given integer is a prime number or not, 16. python program to generate the prime numbers from 1 to n, 17. python program to find the roots of a quadratic equation, 18. python program to print the numbers from a given number n till 0 using recursion, 19. python program to find the factorial of a number using recursion, 20. python program to display the sum of n numbers using a list, 21. python program to implement linear search, 22. python program to implement binary search, 23. python program to find the odd numbers in an array, 24. python program to find the largest number in a list without using built-in functions, 25. python program to insert a number to any position in a list, 26. python program to delete an element from a list by index, 27. python program to check whether a string is palindrome or not, 28. python program to implement matrix addition, 29. python program to implement matrix multiplication, 30. python program to check leap year, 31. python program to find the nth term in a fibonacci series using recursion, 32. python program to print fibonacci series using iteration, 33. python program to print all the items in a dictionary, 34. python program to implement a calculator to do basic operations, 35. python program to draw a circle of squares using turtle.
For practicing more such exercises, I suggest you go to hackerrank.com and sign up. You’ll be able to practice Python there very effectively.
I hope these exercises were helpful to you. If you have any doubts, feel free to let me know in the comments.
12 thoughts on “ 35 Python Programming Exercises and Solutions ”
I don’t mean to nitpick and I don’t want this published but you might want to check code for #16. 4 is not a prime number.
You can only check if integer is a multiple of 35. It always works the same – just multiply all the numbers you need to check for multiplicity.
v_str = str ( input(‘ Enter a valid string or number :- ‘) ) v_rev_str=” for v_d in v_str: v_rev_str = v_d + v_rev_str
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Recent Posts
- How it works
- Homework answers
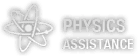
Python Answers
Questions: 5 831
Answers by our Experts: 5 728
Ask Your question
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Search & Filtering
Create a method named check_angles. The sum of a triangle's three angles should return True if the sum is equal to 180, and False otherwise. The method should print whether the angles belong to a triangle or not.
11.1 Write methods to verify if the triangle is an acute triangle or obtuse triangle.
11.2 Create an instance of the triangle class and call all the defined methods.
11.3 Create three child classes of triangle class - isosceles_triangle, right_triangle and equilateral_triangle.
11.4 Define methods which check for their properties.
Create an empty dictionary called Car_0 . Then fill the dictionary with Keys : color , speed , X_position and Y_position.
car_0 = {'x_position': 10, 'y_position': 72, 'speed': 'medium'} .
a) If the speed is slow the coordinates of the X_pos get incremented by 2.
b) If the speed is Medium the coordinates of the X_pos gets incremented by 9
c) Now if the speed is Fast the coordinates of the X_pos gets incremented by 22.
Print the modified dictionary.
Create a simple Card game in which there are 8 cards which are randomly chosen from a deck. The first card is shown face up. The game asks the player to predict whether the next card in the selection will have a higher or lower value than the currently showing card.
For example, say the card that’s shown is a 3. The player chooses “higher,” and the next card is shown. If that card has a higher value, the player is correct. In this example, if the player had chosen “lower,” they would have been incorrect. If the player guesses correctly, they get 20 points. If they choose incorrectly, they lose 15 points. If the next card to be turned over has the same value as the previous card, the player is incorrect.
Consider an ongoing test cricket series. Following are the names of the players and their scores in the test1 and 2.
Test Match 1 :
Dhoni : 56 , Balaji : 94
Test Match 2 :
Balaji : 80 , Dravid : 105
Calculate the highest number of runs scored by an individual cricketer in both of the matches. Create a python function Max_Score (M) that reads a dictionary M that recognizes the player with the highest total score. This function will return ( Top player , Total Score ) . You can consider the Top player as String who is the highest scorer and Top score as Integer .
Input : Max_Score({‘test1’:{‘Dhoni’:56, ‘Balaji : 85}, ‘test2’:{‘Dhoni’ 87, ‘Balaji’’:200}}) Output : (‘Balaji ‘ , 200)
Write a Python program to demonstrate Polymorphism.
1. Class Vehicle with a parameterized function Fare, that takes input value as fare and
returns it to calling Objects.
2. Create five separate variables Bus, Car, Train, Truck and Ship that call the Fare
3. Use a third variable TotalFare to store the sum of fare for each Vehicle Type. 4. Print the TotalFare.
Write a Python program to demonstrate multiple inheritance.
1. Employee class has 3 data members EmployeeID , Gender (String) , Salary and
PerformanceRating ( Out of 5 ) of type int. It has a get() function to get these details from
2. JoiningDetail class has a data member DateOfJoining of type Date and a function
getDoJ to get the Date of joining of employees.
3. Information Class uses the marks from Employee class and the DateOfJoining date
from the JoiningDetail class to calculate the top 3 Employees based on their Ratings and then Display, using readData , all the details on these employees in Ascending order of their Date Of Joining.
You are given an array of numbers as input: [10,20,10,40,50,45,30,70,5,20,45] and a target value: 50. You are required to find pairs of elements (indices of two numbers) from the given array whose sum equals a specific target number. Your solution should not use the same element twice, thus it must be a single solution for each input
1.1 Write a Python class that defines a function to find pairs which takes 2 parameters (input array and target value) and returns a list of pairs whose sum is equal to target given above. You are required to print the list of pairs and state how many pairs if found. Your solution should call the function to find pairs, then return a list of pairs.
1.2 Given the input array nums in 1.1 above. Write a second program to find a set of good pairs from that input array nums. Here a pair (i,j) is said to be a good pair if nums[i] is the same as nums[j] and i < j. You are required to display an array of good pairs indices and the number of good pairs.
How to find largest number inn list in python
Given a list of integers, write a program to print the sum of all prime numbers in the list of integers.
Note: one is neither prime nor composite number
Using the pass statement
how to get a input here ! example ! 2 4 5 6 7 8 2 4 5 2 3 8 how to get it?
- Programming
- Engineering
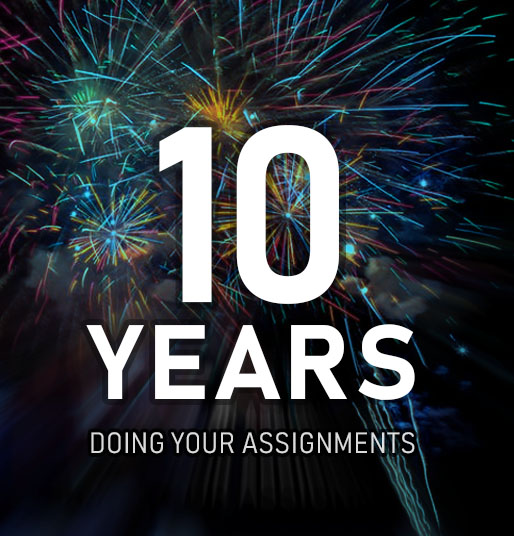
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python exercises.
You can test your Python skills with W3Schools' Exercises.
We have gathered a variety of Python exercises (with answers) for each Python Chapter.
Try to solve an exercise by filling in the missing parts of a code. If you're stuck, hit the "Show Answer" button to see what you've done wrong.
Count Your Score
You will get 1 point for each correct answer. Your score and total score will always be displayed.
Start Python Exercises
Start Python Exercises ❯
If you don't know Python, we suggest that you read our Python Tutorial from scratch.
Kickstart your career
Get certified by completing the course

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
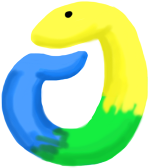
Practice Python
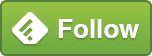
Beginner Python exercises
- Why Practice Python?
- Why Chilis?
- Resources for learners
All Exercises

All Solutions
- 1: Character Input Solutions
- 2: Odd Or Even Solutions
- 3: List Less Than Ten Solutions
- 4: Divisors Solutions
- 5: List Overlap Solutions
- 6: String Lists Solutions
- 7: List Comprehensions Solutions
- 8: Rock Paper Scissors Solutions
- 9: Guessing Game One Solutions
- 10: List Overlap Comprehensions Solutions
- 11: Check Primality Functions Solutions
- 12: List Ends Solutions
- 13: Fibonacci Solutions
- 14: List Remove Duplicates Solutions
- 15: Reverse Word Order Solutions
- 16: Password Generator Solutions
- 17: Decode A Web Page Solutions
- 18: Cows And Bulls Solutions
- 19: Decode A Web Page Two Solutions
- 20: Element Search Solutions
- 21: Write To A File Solutions
- 22: Read From File Solutions
- 23: File Overlap Solutions
- 24: Draw A Game Board Solutions
- 25: Guessing Game Two Solutions
- 26: Check Tic Tac Toe Solutions
- 27: Tic Tac Toe Draw Solutions
- 28: Max Of Three Solutions
- 29: Tic Tac Toe Game Solutions
- 30: Pick Word Solutions
- 31: Guess Letters Solutions
- 32: Hangman Solutions
- 33: Birthday Dictionaries Solutions
- 34: Birthday Json Solutions
- 35: Birthday Months Solutions
- 36: Birthday Plots Solutions
- 37: Functions Refactor Solution
- 38: f Strings Solution
- 39: Character Input Datetime Solution
- 40: Error Checking Solution
- Python Crash Course on GitHub
Solutions for selected exercises from each chapter can be found below. Be careful about looking at the solutions too quickly; make sure you’ve given yourself time to wrestle with the concepts you just learned before looking at a solution. Also, there are several ways to solve many of the exercises, and the solutions only show one possible way to complete each exercise.
I haven’t included solutions for Chapters 18-20, because the exercises for those chapters are really projects in themselves. If you’re having trouble with an exercise from one of those chapters consider posting on Stack Overflow , r/learnpython , or get in touch.
Questions and Feedback
If you’re stuck on something and what you see here isn’t helping, please feel free to get in touch. Also, if you think you’ve found a mistake in the book or in the online resources, I’d love to know about it!
Email: [email protected]
Twitter: @ehmatthes
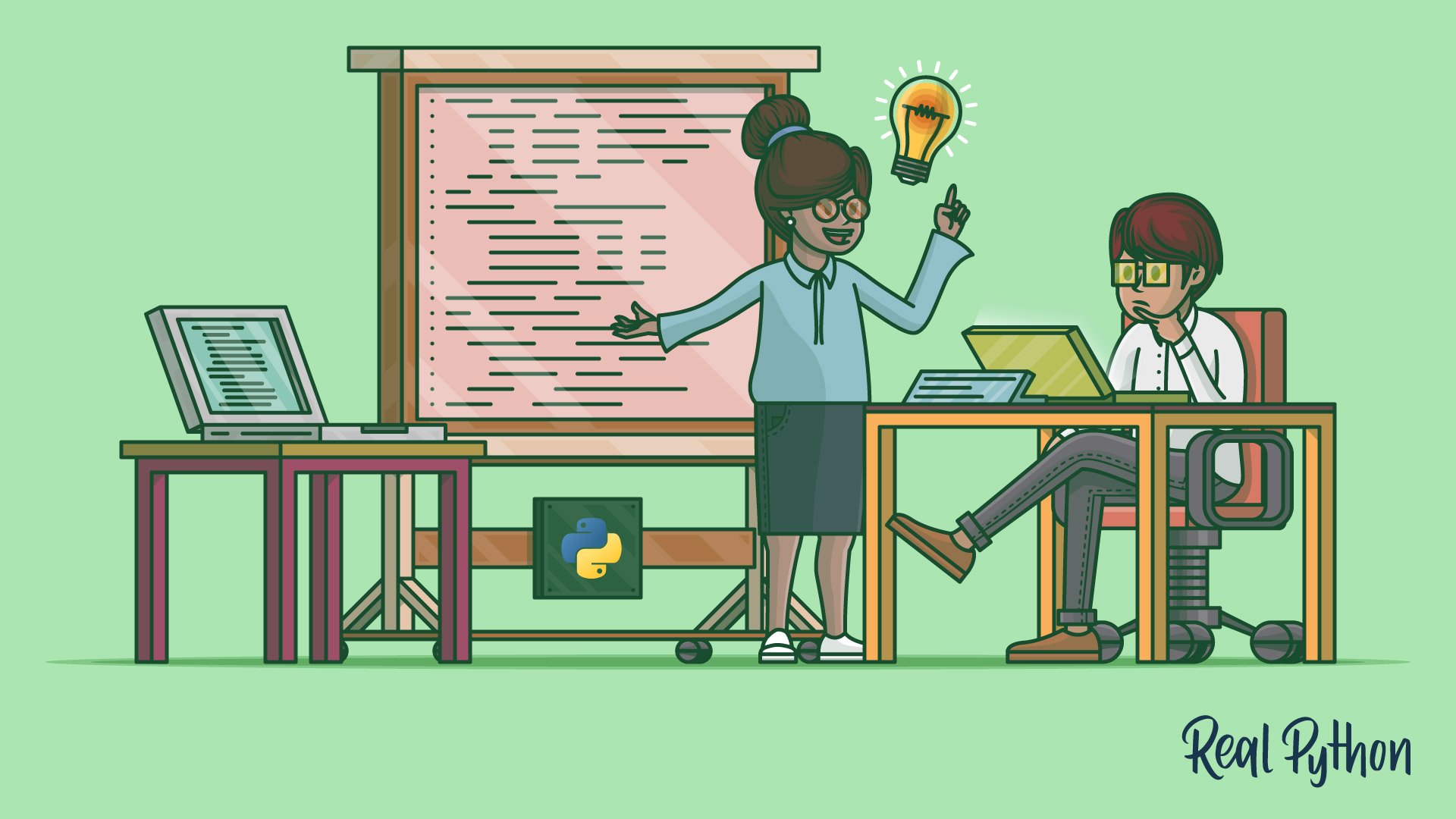
Python Practice Problems: Get Ready for Your Next Interview
Table of Contents
Problem Description
Problem solution.
Are you a Python developer brushing up on your skills before an interview ? If so, then this tutorial will usher you through a series of Python practice problems meant to simulate common coding test scenarios. After you develop your own solutions, you’ll walk through the Real Python team’s answers so you can optimize your code, impress your interviewer, and land your dream job!
In this tutorial, you’ll learn how to:
- Write code for interview-style problems
- Discuss your solutions during the interview
- Work through frequently overlooked details
- Talk about design decisions and trade-offs
This tutorial is aimed at intermediate Python developers. It assumes a basic knowledge of Python and an ability to solve problems in Python. You can get skeleton code with failing unit tests for each of the problems you’ll see in this tutorial by clicking on the link below:
Download the sample code: Click here to get the code you’ll use to work through the Python practice problems in this tutorial.
Each of the problems below shows the file header from this skeleton code describing the problem requirements. So download the code, fire up your favorite editor, and let’s dive into some Python practice problems!
Python Practice Problem 1: Sum of a Range of Integers
Let’s start with a warm-up question. In the first practice problem, you’ll write code to sum a list of integers . Each practice problem includes a problem description. This description is pulled directly from the skeleton files in the repo to make it easier to remember while you’re working on your solution.
You’ll see a solution section for each problem as well. Most of the discussion will be in a collapsed section below that. Clone that repo if you haven’t already, work out a solution to the following problem, then expand the solution box to review your work.
Here’s your first problem:
Sum of Integers Up To n ( integersums.py ) Write a function, add_it_up() , that takes a single integer as input and returns the sum of the integers from zero to the input parameter. The function should return 0 if a non-integer is passed in.
Remember to run the unit tests until you get them passing!
Here’s some discussion of a couple of possible solutions.
Note: Remember, don’t open the collapsed section below until you’re ready to look at the answer for this Python practice problem!
Solution for Sum of a Range of Integers Show/Hide
How did writing the solution go? Ready to look at the answer?
For this problem, you’ll look at a few different solutions. The first of these is not so good:
In this solution, you manually build a while loop to run through the numbers 1 through n . You keep a running sum and then return it when you’ve finished the loop.
This solution works, but it has two problems:
It doesn’t display your knowledge of Python and how the language simplifies tasks like this.
It doesn’t meet the error conditions in the problem description. Passing in a string will result in the function throwing an exception when it should just return 0 .
You’ll deal with the error conditions in the final answer below, but first let’s refine the core solution to be a bit more Pythonic .
The first thing to think about is that while loop . Python has powerful mechanisms for iterating over lists and ranges. Creating your own is usually unnecessary, and that’s certainly the case here. You can replace the while loop with a loop that iterates over a range() :
You can see that the for...range() construct has replaced your while loop and shortened the code. One thing to note is that range() goes up to but does not include the number given, so you need to use n + 1 here.
This was a nice step! It removes some of the boilerplate code of looping over a range and makes your intention clearer. But there’s still more you can do here.
Summing a list of integers is another thing Python is good at:
Wow! By using the built-in sum() , you got this down to one line of code! While code golf generally doesn’t produce the most readable code, in this case you have a win-win: shorter and more readable code.
There’s one problem remaining, however. This code still doesn’t handle the error conditions correctly. To fix that, you can wrap your previous code in a try...except block:
This solves the problem and handles the error conditions correctly. Way to go!
Occasionally, interviewers will ask this question with a fixed limit, something like “Print the sum of the first nine integers.” When the problem is phrased that way, one correct solution would be print(45) .
If you give this answer, however, then you should follow up with code that solves the problem step by step. The trick answer is a good place to start your answer, but it’s not a great place to end.
If you’d like to extend this problem, try adding an optional lower limit to add_it_up() to give it more flexibility!
Python Practice Problem 2: Caesar Cipher
The next question is a two-parter. You’ll code up a function to compute a Caesar cipher on text input. For this problem, you’re free to use any part of the Python standard library to do the transform.
Hint: There’s a function in the str class that will make this task much easier!
The problem statement is at the top of the skeleton source file:
Caesar Cipher ( caesar.py ) A Caesar cipher is a simple substitution cipher in which each letter of the plain text is substituted with a letter found by moving n places down the alphabet. For example, assume the input plain text is the following: abcd xyz If the shift value, n , is 4, then the encrypted text would be the following: efgh bcd You are to write a function that accepts two arguments, a plain-text message and a number of letters to shift in the cipher. The function will return an encrypted string with all letters transformed and all punctuation and whitespace remaining unchanged. Note: You can assume the plain text is all lowercase ASCII except for whitespace and punctuation.
Remember, this part of the question is really about how well you can get around in the standard library. If you find yourself figuring out how to do the transform without the library, then save that thought! You’ll need it later!
Here’s a solution to the Caesar cipher problem described above.
Note: Remember, don’t open the collapsed section below until you’re ready to look at the answers for this Python practice problem!
Solution for Caesar Cipher Show/Hide
This solution makes use of .translate() from the str class in the standard library. If you struggled with this problem, then you might want to pause a moment and consider how you could use .translate() in your solution.
Okay, now that you’re ready, let’s look at this solution:
You can see that the function makes use of three things from the string module:
- .ascii_lowercase
- .maketrans()
- .translate()
In the first two lines, you create a variable with all the lowercase letters of the alphabet (ASCII only for this program) and then create a mask , which is the same set of letters, only shifted. The slicing syntax is not always obvious, so let’s walk through it with a real-world example:
You can see that x[3:] is all the letters after the third letter, 'c' , while x[:3] is just the first three letters.
Line 6 in the solution, letters[shift_num:] + letters[:shift_num] , creates a list of letters shifted by shift_num letters, with the letters at the end wrapped around to the front. Once you have the list of letters and the mask of letters you want to map to, you call .maketrans() to create a translation table.
Next, you pass the translation table to the string method .translate() . It maps all characters in letters to the corresponding letters in mask and leaves all other characters alone.
This question is an exercise in knowing and using the standard library. You may be asked a question like this at some point during an interview. If that happens to you, it’s good to spend some time thinking about possible answers. If you can remember the method— .translate() in this case—then you’re all set.
But there are a couple of other scenarios to consider:
You may completely draw a blank. In this case, you’ll probably solve this problem the way you solve the next one , and that’s an acceptable answer.
You may remember that the standard library has a function to do what you want but not remember the details.
If you were doing normal work and hit either of these situations, then you’d just do some searching and be on your way. But in an interview situation, it will help your cause to talk through the problem out loud.
Asking the interviewer for specific help is far better than just ignoring it. Try something like “I think there’s a function that maps one set of characters to another. Can you help me remember what it’s called?”
In an interview situation, it’s often better to admit that you don’t know something than to try to bluff your way through.
Now that you’ve seen a solution using the Python standard library, let’s try the same problem again, but without that help!
Python Practice Problem 3: Caesar Cipher Redux
For the third practice problem, you’ll solve the Caesar cipher again, but this time you’ll do it without using .translate() .
The description of this problem is the same as the previous problem. Before you dive into the solution, you might be wondering why you’re repeating the same exercise, just without the help of .translate() .
That’s a great question. In normal life, when your goal is to get a working, maintainable program, rewriting parts of the standard library is a poor choice. The Python standard library is packed with working, well-tested, and fast solutions for problems large and small. Taking full advantage of it is a mark of a good programmer.
That said, this is not a work project or a program you’re building to satisfy a need. This is a learning exercise, and it’s the type of question that might be asked during an interview. The goal for both is to see how you can solve the problem and what interesting design trade-offs you make while doing it.
So, in the spirit of learning, let’s try to resolve the Caesar cipher without .translate() .
For this problem, you’ll have two different solutions to look at when you’re ready to expand the section below.
Solutions for Caesar Cipher Redux Show/Hide
For this problem, two different solutions are provided. Check out both and see which one you prefer!
For the first solution, you follow the problem description closely, adding an amount to each character and flipping it back to the beginning of the alphabet when it goes on beyond z :
Starting on line 14, you can see that caesar() does a list comprehension , calling a helper function for each letter in message . It then does a .join() to create the new encoded string. This is short and sweet, and you’ll see a similar structure in the second solution. The interesting part happens in shift_n() .
Here you can see another use for string.ascii_lowercase , this time filtering out any letter that isn’t in that group. Once you’re certain you’ve filtered out any non-letters, you can proceed to encoding. In this version of encoding, you use two functions from the Python standard library:
Again, you’re encouraged not only to learn these functions but also to consider how you might respond in an interview situation if you couldn’t remember their names.
ord() does the work of converting a letter to a number, and chr() converts it back to a letter. This is handy as it allows you to do arithmetic on letters, which is what you want for this problem.
The first step of your encoding on line 7 gets the numeric value of the encoded letter by using ord() to get the numeric value of the original letter. ord() returns the Unicode code point of the character, which turns out to be the ASCII value.
For many letters with small shift values, you can convert the letter back to a character and you’ll be done. But consider the starting letter, z .
A shift of one character should result in the letter a . To achieve this wraparound, you find the difference from the encoded letter to the letter z . If that difference is positive, then you need to wrap back to the beginning.
You do this in lines 8 to 11 by repeatedly adding 26 to or subtracting it from the character until it’s in the range of ASCII characters. Note that this is a fairly inefficient method for fixing this issue. You’ll see a better solution in the next answer.
Finally, on line 12, your conversion shift function takes the numeric value of the new letter and converts it back to a letter to return it.
While this solution takes a literal approach to solving the Caesar cipher problem, you could also use a different approach modeled after the .translate() solution in practice problem 2 .
The second solution to this problem mimics the behavior of Python’s built-in method .translate() . Instead of shifting each letter by a given amount, it creates a translation map and uses it to encode each letter:
Starting with caesar() on line 11, you start by fixing the problem of amount being greater than 26 . In the previous solution, you looped repeatedly until the result was in the proper range. Here, you take a more direct and more efficient approach using the mod operator ( % ).
The mod operator produces the remainder from an integer division. In this case, you divide by 26 , which means the results are guaranteed to be between 0 and 25 , inclusive.
Next, you create the translation table. This is a change from the previous solutions and is worth some attention. You’ll see more about this toward the end of this section.
Once you create the table , the rest of caesar() is identical to the previous solution: a list comprehension to encrypt each letter and a .join() to create a string.
shift_n() finds the index of the given letter in the alphabet and then uses this to pull a letter from the table . The try...except block catches those cases that aren’t found in the list of lowercase letters.
Now let’s discuss the table creation issue. For this toy example, it probably doesn’t matter too much, but it illustrates a situation that occurs frequently in everyday development: balancing clarity of code against known performance bottlenecks.
If you examine the code again, you’ll see that table is used only inside shift_n() . This indicates that, in normal circumstances, it should have been created in, and thus have its scope limited to, shift_n() :
The issue with that approach is that it spends time calculating the same table for every letter of the message. For small messages, this time will be negligible, but it might add up for larger messages.
Another possible way that you could avoid this performance penalty would be to make table a global variable . While this also cuts down on the construction penalty, it makes the scope of table even larger. This doesn’t seem better than the approach shown above.
At the end of the day, the choice between creating table once up front and giving it a larger scope or just creating it for every letter is what’s called a design decision . You need to choose the design based on what you know about the actual problem you’re trying to solve.
If this is a small project and you know it will be used to encode large messages, then creating the table only once could be the right decision. If this is only a portion of a larger project, meaning maintainability is key, then perhaps creating the table each time is the better option.
Since you’ve looked at two solutions, it’s worth taking a moment to discuss their similarities and differences.
Solution Comparison
You’ve seen two solutions in this part of the Caesar cipher, and they’re fairly similar in many ways. They’re about the same number of lines. The two main routines are identical except for limiting amount and creating table . It’s only when you look at the two versions of the helper function, shift_n() , that the differences appear.
The first shift_n() is an almost literal translation of what the problem is asking for: “Shift the letter down the alphabet and wrap it around at z .” This clearly maps back to the problem statement, but it has a few drawbacks.
Although it’s about the same length as the second version, the first version of shift_n() is more complex. This complexity comes from the letter conversion and math needed to do the translation. The details involved—converting to numbers, subtracting, and wrapping—mask the operation you’re performing. The second shift_n() is far less involved in its details.
The first version of the function is also specific to solving this particular problem. The second version of shift_n() , like the standard library’s .translate() that it’s modeled after, is more general-purpose and can be used to solve a larger set of problems. Note that this is not necessarily a good design goal.
One of the mantras that came out of the Extreme Programming movement is “You aren’t gonna need it” (YAGNI). Frequently, software developers will look at a function like shift_n() and decide that it would be better and more general-purpose if they made it even more flexible, perhaps by passing in a parameter instead of using string.ascii_lowercase .
While that would indeed make the function more general-purpose, it would also make it more complex. The YAGNI mantra is there to remind you not to add complexity before you have a specific use case for it.
To wrap up your Caesar cipher section, there are clear trade-offs between the two solutions, but the second shift_n() seems like a slightly better and more Pythonic function.
Now that you’ve written the Caesar cipher three different ways, let’s move on to a new problem.
Python Practice Problem 4: Log Parser
The log parser problem is one that occurs frequently in software development. Many systems produce log files during normal operation, and sometimes you’ll need to parse these files to find anomalies or general information about the running system.
For this problem, you’ll need to parse a log file with a specified format and generate a report:
Log Parser ( logparse.py ) Accepts a filename on the command line. The file is a Linux-like log file from a system you are debugging. Mixed in among the various statements are messages indicating the state of the device. They look like this: Jul 11 16:11:51:490 [139681125603136] dut: Device State: ON The device state message has many possible values, but this program cares about only three: ON , OFF , and ERR . Your program will parse the given log file and print out a report giving how long the device was ON and the timestamp of any ERR conditions.
Note that the provided skeleton code doesn’t include unit tests. This was omitted since the exact format of the report is up to you. Think about and write your own during the process.
A test.log file is included, which provides you with an example. The solution you’ll examine produces the following output:
While that format is generated by the Real Python solution, you’re free to design your own format for the output. The sample input file should generate equivalent information.
In the collapsed section below, you’ll find a possible solution to the log parser problem. When you’re ready, expand the box and compare it with what you came up with!
Solution for Log Parser Problem Show/Hide
Full Solution
Since this solution is longer than what you saw for the integer sums or the Caesar cipher problems, let’s start with the full program:
That’s your full solution. You can see that the program consists of three functions and the main section. You’ll work through them from the top.
Helper Function: get_next_event()
First up is get_next_event() :
Because it contains a yield statement, this function is a generator . That means you can use it to generate one event from the log file at a time.
You could have just used for line in datafile , but instead you add a little bit of filtering. The calling routine will get only those events that have dut: Device State: in them. This keeps all the file-specific parsing contained in a single function.
This might make get_next_event() a bit more complicated, but it’s a relatively small function, so it remains short enough to read and comprehend. It also keeps that complicated code encapsulated in a single location.
You might be wondering when datafile gets closed. As long as you call the generator until all of the lines are read from datafile , the for loop will complete, allowing you to leave the with block and exit from the function.
Helper Function: compute_time_diff_seconds()
The second function is compute_time_diff_seconds() , which, as the name suggests, computes the number of seconds between two timestamps:
There are a few interesting points to this function. The first is that subtracting the two datetime objects results in a datetime.timedelta . For this problem, you will report total seconds, so returning .total_seconds() from the timedelta is appropriate.
The second item of note is that there are many, many packages in Python that simplify handling dates and times. In this case, your use model is simple enough that it doesn’t warrant the complexity of pulling in an external library when the standard library functions will suffice.
That said, datetime.datetime.strptime() is worthy of mention. When passed a string and a specific format, .strptime() parses that string with the given format and produces a datetime object.
This is another place where, in an interview situation, it’s important not to panic if you can’t remember the exact names of the Python standard library functions.
Helper Function: extract_data()
Next up is extract_data() , which does the bulk of the work in this program. Before you dive into the code, let’s step back and talk about state machines.
State machines are software (or hardware) devices that transition from one state to another depending on specific inputs. That’s a really broad definition that might be difficult to grasp, so let’s look at a diagram of the state machine you’ll be using below:
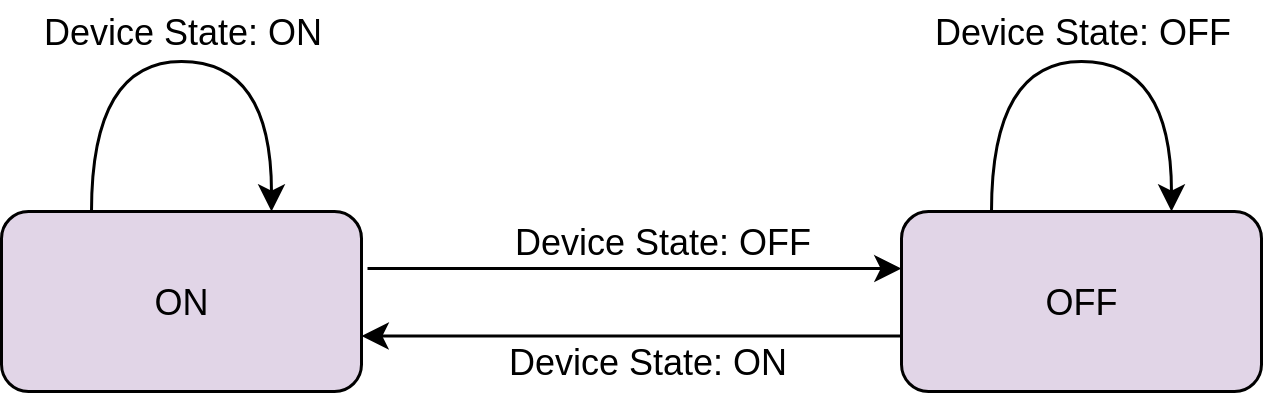
In this diagram, the states are represented by the labeled boxes. There are only two states here, ON and OFF , which correspond to the state of the device. There are also two input signals, Device State: ON and Device State: OFF . The diagram uses arrows to show what happens when an input occurs while the machine is in each state.
For example, if the machine is in the ON state and the Device State: ON input occurs, then the machine stays in the ON state. No change happens. Conversely, if the machine receives the Device State: OFF input when it’s in the ON state, then it will transition to the OFF state.
While the state machine here is only two states with two inputs, state machines are often much more complex. Creating a diagram of expected behavior can help you make the code that implements the state machine more concise.
Let’s move back to extract_data() :
It might be hard to see the state machine here. Usually, state machines require a variable to hold the state. In this case, you use time_on_started to serve two purposes:
- Indicate state: time_on_started holds the state of your state machine. If it’s None , then the machine is in the OFF state. If it’s not None , then the machine is ON .
- Store start time: If the state is ON , then time_on_started also holds the timestamp of when the device turned on. You use this timestamp to call compute_time_diff_seconds() .
The top of extract_data() sets up your state variable, time_on_started , and also the two outputs you want. errs is a list of timestamps at which the ERR message was found, and total_time_on is the sum of all periods when the device was on.
Once you’ve completed the initial setup, you call the get_next_event() generator to retrieve each event and timestamp. The action it receives is used to drive the state machine, but before it checks for state changes, it first uses an if block to filter out any ERR conditions and add those to errs .
After the error check, the first elif block handles transitions to the ON state. You can transition to ON only when you’re in the OFF state, which is signaled by time_on_started being False . If you’re not already in the ON state and the action is "ON" , then you store the timestamp , putting the machine into the ON state.
The second elif handles the transition to the OFF state. On this transition, extract_data() needs to compute the number of seconds the device was on. It does this using the compute_time_diff_seconds() you saw above. It adds this time to the running total_time_on and sets time_on_started back to None , effectively putting the machine back into the OFF state.
Main Function
Finally, you can move on to the __main__ section. This final section passes sys.argv[1] , which is the first command-line argument , to extract_data() and then presents a report of the results:
To call this solution, you run the script and pass the name of the log file. Running your example code results in this output:
Your solution might have different formatting, but the information should be the same for the sample log file.
There are many ways to solve a problem like this. Remember that in an interview situation, talking through the problem and your thought process can be more important than which solution you choose to implement.
That’s it for the log-parsing solution. Let’s move on to the final challenge: sudoku!
Python Practice Problem 5: Sudoku Solver
Your final Python practice problem is to solve a sudoku puzzle!
Finding a fast and memory-efficient solution to this problem can be quite a challenge. The solution you’ll examine has been selected for readability rather than speed, but you’re free to optimize your solution as much as you want.
The description for the sudoku solver is a little more involved than the previous problems:
Sudoku Solver ( sudokusolve.py ) Given a string in SDM format, described below, write a program to find and return the solution for the sudoku puzzle in the string. The solution should be returned in the same SDM format as the input. Some puzzles will not be solvable. In that case, return the string “Unsolvable”. The general SDM format is described here . For our purposes, each SDM string will be a sequence of 81 digits, one for each position on the sudoku puzzle. Known numbers will be given, and unknown positions will have a zero value. For example, assume you’re given this string of digits: 004006079000000602056092300078061030509000406020540890007410920105000000840600100 The string represents this starting sudoku puzzle: 0 0 4 0 0 6 0 7 9 0 0 0 0 0 0 6 0 2 0 5 6 0 9 2 3 0 0 0 7 8 0 6 1 0 3 0 5 0 9 0 0 0 4 0 6 0 2 0 5 4 0 8 9 0 0 0 7 4 1 0 9 2 0 1 0 5 0 0 0 0 0 0 8 4 0 6 0 0 1 0 0 The provided unit tests may take a while to run, so be patient. Note: A description of the sudoku puzzle can be found on Wikipedia .
You can see that you’ll need to deal with reading and writing to a particular format as well as generating a solution.
When you’re ready, you can find a detailed explanation of a solution to the sudoku problem in the box below. A skeleton file with unit tests is provided in the repo.
Solution for Sudoku Solver Show/Hide
This is a larger and more complex problem than you’ve looked at so far in this tutorial. You’ll walk through the problem step by step, ending with a recursive function that solves the puzzle. Here’s a rough outline of the steps you’ll take:
- Read the puzzle into a grid form.
- Place the number in the cell.
- Remove that number from the row, column, and small square.
- Move to the next position.
- If no possible numbers remain, then declare the puzzle unsolvable .
- If all cells are filled, then return the solution .
The tricky part of this algorithm is keeping track of the grid at each step of the process. You’ll use recursion, making a new copy of the grid at each level of the recursion, to maintain this information.
With that outline in mind, let’s start with the first step, creating the grid.
Generating a Grid From a Line
To start, it’s helpful to convert the puzzle data into a more usable format. Even if you eventually want to solve the puzzle in the given SDM format , you’ll likely make faster progress working through the details of your algorithm with the data in a grid form. Once you have a solution that works, then you can convert it to work on a different data structure.
To this end, let’s start with a couple of conversion functions:
Your first function, line_to_grid() , converts the data from a single string of eighty-one digits to a list of lists. For example, it converts the string line to a grid like start :
Each inner list here represents a horizontal row in your sudoku puzzle.
You start with an empty grid and an empty line . You then build each line by converting nine characters from the values string to single-digit integers and then appending them to the current line . Once you have nine values in a line , as indicated by index % 9 == 0 on line 7, you insert that line into the grid and start a new one.
The function ends by appending the final line to the grid . You need this because the for loop will end with the last line still stored in the local variable and not yet appended to grid .
The inverse function, grid_to_line() , is slightly shorter. It uses a generator expression with .join() to create a nine-digit string for each row. It then appends that string to the overall line and returns it. Note that it’s possible to use nested generators to create this result in fewer lines of code, but the readability of the solution starts to fall off dramatically.
Now that you’ve got the data in the data structure you want, let’s start working with it.
Generating a Small Square Iterator
Your next function is a generator that will help you search for the smaller three-by-three square a given position is in. Given the x- and y-coordinates of the cell in question, this generator will produce a list of coordinates that match the square that contains it:
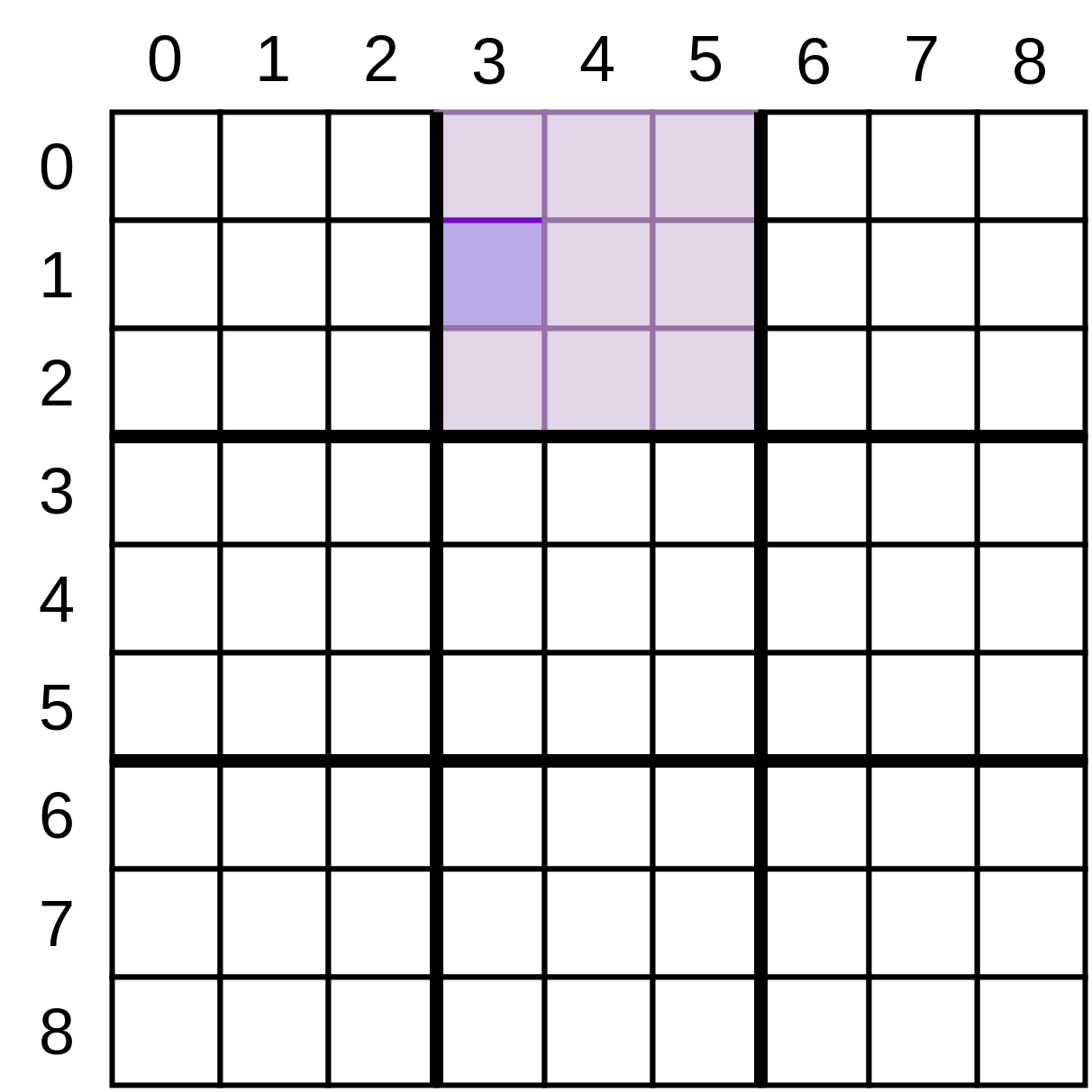
In the image above, you’re examining cell (3, 1) , so your generator will produce coordinate pairs corresponding to all the lightly shaded cells, skipping the coordinates that were passed in:
Putting the logic for determining this small square in a separate utility function keeps the flow of your other functions more readable. Making this a generator allows you to use it in a for loop to iterate through each of the values.
The function to do this involves using the limitations of integer math:
There are a lot of threes in a couple of those lines, which makes lines like ((x + 3) // 3) * 3 look confusing. Here’s what happens when x is 1 .
Using the rounding of integer math allows you to get the next-highest multiple of three above a given value. Once you have this, subtracting three will give you the multiple of three below the given number.
There are a few more low-level utility functions to examine before you start building on top of them.
Moving to the Next Spot
Your solution will need to walk through the grid structure one cell at a time. This means that at some point, you’ll need to figure out what the next position should be. compute_next_position() to the rescue!
compute_next_position() takes the current x- and y-coordinates as input and returns a tuple containing a finished flag along with the x- and y-coordinates of the next position:
The finished flag tells the caller that the algorithm has walked off the end of the puzzle and has completed all the squares. You’ll see how that’s used in a later section.
Removing Impossible Numbers
Your final low-level utility is quite small. It takes an integer value and an iterable. If the value is nonzero and appears in the iterable, then the function removes it from the iterable:
Typically, you wouldn’t make this small bit of functionality into a function. You’ll use this function several times, though, so it’s best to follow the DRY principle and pull it up to a function.
Now you’ve seen the bottom level of the functionality pyramid. It’s time to step up and use those tools to build a more complex function. You’re almost ready to solve the puzzle!
Finding What’s Possible
Your next function makes use of some of the low-level functions you’ve just walked through. Given a grid and a position on that grid, it determines what values that position could still have:
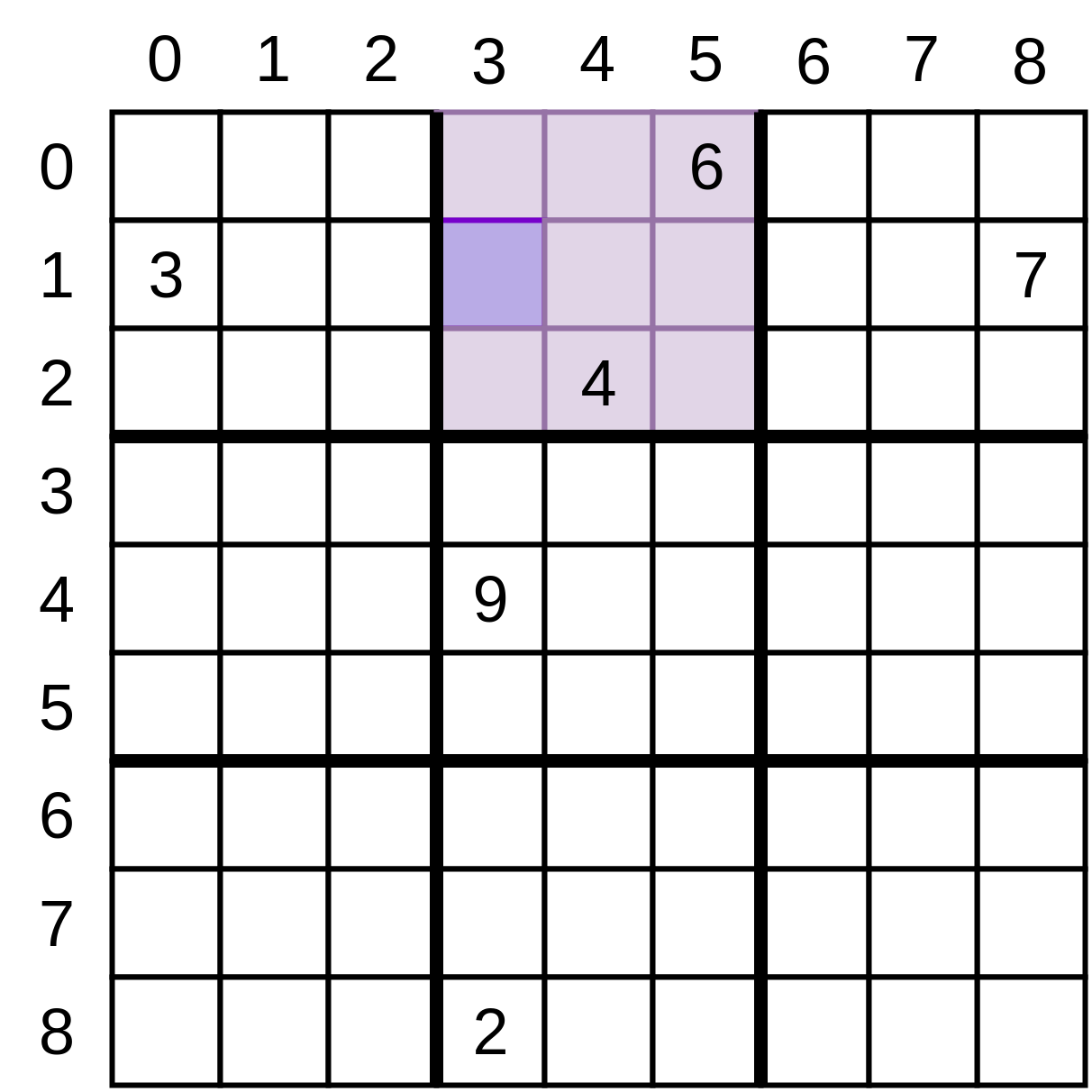
For the grid above, at the position (3, 1) , the possible values are [1, 5, 8] because the other values are all present, either in that row or column or in the small square you looked at earlier.
This is the responsibility of detect_possible() :
The function starts by checking if the given position at x and y already has a nonzero value. If so, then that’s the only possible value and it returns.
If not, then the function creates a set of the numbers one through nine. The function proceeds to check different blocking numbers and removes those from this set.
It starts by checking the column and row of the given position. This can be done with a single loop by just alternating which subscript changes. grid[x][index] checks values in the same column, while grid[index][y] checks those values in the same row. You can see that you’re using test_and_remove() here to simplify the code.
Once those values have been removed from your possible set, the function moves on to the small square. This is where the small_square() generator you created before comes in handy. You can use it to iterate over each position in the small square, again using test_and_remove() to eliminate any known values from your possible list.
Once all the known blocking values have been removed from your set, you have the list of all possible values for that position on that grid.
You might wonder why the code and its description make a point about the position being “on that grid.” In your next function, you’ll see that the program makes many copies of the grid as it tries to solve it.
You’ve reached the heart of this solution: solve() ! This function is recursive, so a little up-front explanation might help.
The general design of solve() is based on testing a single position at a time. For the position of interest, the algorithm gets the list of possible values and then selects those values, one at a time, to be in this position.
For each of these values, it creates a grid with the guessed value in this position. It then calls a function to test for a solution, passing in the new grid and the next position.
It just so happens that the function it calls is itself.
For any recursion, you need a termination condition. This algorithm has four of them:
- There are no possible values for this position. That indicates the solution it’s testing can’t work.
- It’s walked to the end of the grid and found a possible value for each position. The puzzle is solved!
- One of the guesses at this position, when passed back to the solver, returns a solution.
- It’s tried all possible values at this position and none of them will work.
Let’s look at the code for this and see how it all plays out:
The first thing to note in this function is that it makes a .deepcopy() of the grid. It does a deep copy because the algorithm needs to keep track of exactly where it was at any point in the recursion. If the function made only a shallow copy, then every recursive version of this function would use the same grid.
Once the grid is copied, solve() can work with the new copy, temp . A position on the grid was passed in, so that’s the number that this version of the function will solve. The first step is to see what values are possible in this position. As you saw earlier, detect_possible() returns a list of possible values that may be empty.
If there are no possible values, then you’ve hit the first termination condition for the recursion. The function returns False , and the calling routine moves on.
If there are possible values, then you need to move on and see if any of them is a solution. Before you do that, you can add a little optimization to the code. If there’s only a single possible value, then you can insert that value and move on to the next position. The solution shown does this in a loop, so you can place multiple numbers into the grid without having to recur.
This may seem like a small improvement, and I’ll admit my first implementation did not include this. But some testing showed that this solution was dramatically faster than simply recurring here at the price of more complex code.
Note: This is an excellent point to bring up during an interview even if you don’t add the code to do this. Showing them that you’re thinking about trading off speed against complexity is a strong positive signal to interviewers.
Sometimes, of course, there will be multiple possible values for the current position, and you’ll need to decide if any of them will lead to a solution. Fortunately, you’ve already determined the next position in the grid, so you can forgo placing the possible values.
If the next position is off the end of the grid, then the current position is the final one to fill. If you know that there’s at least one possible value for this position, then you’ve found a solution! The current position is filled in and the completed grid is returned up to the calling function.
If the next position is still on the grid, then you loop through each possible value for the current spot, filling in the guess at the current position and then calling solve() with the temp grid and the new position to test.
solve() can return only a completed grid or False , so if any of the possible guesses returns a result that isn’t False , then a result has been found, and that grid can be returned up the stack.
If all possible guesses have been made and none of them is a solution, then the grid that was passed in is unsolvable. If this is the top-level call, then that means the puzzle is unsolvable. If the call is lower in the recursion tree, then it just means that this branch of the recursion tree isn’t viable.
Putting It All Together
At this point, you’re almost through the solution. There’s only one final function left, sudoku_solve() :
This function does three things:
- Converts the input string into a grid
- Calls solve() with that grid to get a solution
- Returns the solution as a string or "Unsolvable" if there’s no solution
That’s it! You’ve walked through a solution for the sudoku solver problem.
Interview Discussion Topics
The sudoku solver solution you just walked through is a good deal of code for an interview situation. Part of an interview process would likely be to discuss some of the code and, more importantly, some of the design trade-offs you made. Let’s look at a few of those trade-offs.
The biggest design decision revolves around using recursion. It’s possible to write a non-recursive solution to any problem that has a recursive solution. Why choose recursion over another option?
This is a discussion that depends not only on the problem but also on the developers involved in writing and maintaining the solution. Some problems lend themselves to rather clean recursive solutions, and some don’t.
In general, recursive solutions will take more time to run and use more memory than non-recursive solutions. But that’s not always true and, more importantly, it’s not always important .
Similarly, some teams of developers are comfortable with recursive solutions, while others find them exotic or unnecessarily complex. Maintainability should play into your design decisions as well.
One good discussion to have about a decision like this is around performance. How fast does this solution need to execute? Will it be used to solve billions of puzzles or just a handful? Will it run on a small embedded system with memory constraints, or will it be on a large server?
These external factors can help you decide which is a better design decision. These are great topics to bring up in an interview as you’re working through a problem or discussing code. A single product might have places where performance is critical (doing ray tracing on a graphics algorithm, for example) and places where it doesn’t matter at all (such as parsing the version number during installation).
Bringing up topics like this during an interview shows that you’re not only thinking about solving an abstract problem, but you’re also willing and able to take it to the next level and solve a specific problem facing the team.
Readability and Maintainability
Sometimes it’s worth picking a solution that’s slower in order to make a solution that’s easier to work with, debug, and extend. The decision in the sudoku solver challenge to convert the data structure to a grid is one of those decisions.
That design decision likely slows down the program, but unless you’ve measured, you don’t know. Even if it does, putting the data structure into a form that’s natural for the problem can make the code easier to comprehend.
It’s entirely possible to write a solver that operates on the linear strings you’re given as input. It’s likely faster and probably takes less memory, but small_square() , among others, will be a lot harder to write, read, and maintain in this version.
Another thing to discuss with an interviewer, whether you’re live coding or discussing code you wrote offline, is the mistakes and false turns you took along the way.
This is a little less obvious and can be slightly detrimental, but particularly if you’re live coding, taking a step to refactor code that isn’t right or could be better can show how you work. Few developers can write perfect code the first time. Heck, few developers can write good code the first time.
Good developers write the code, then go back and refactor it and fix it. For example, my first implementation of detect_possible() looked like this:
Ignoring that it doesn’t consider the small_square() information, this code can be improved. If you compare this to the final version of detect_possible() above, you’ll see that the final version uses a single loop to test both the horizontal and the vertical dimensions.
Wrapping Up
That’s your tour through a sudoku solver solution. There’s more information available on formats for storing puzzles and a huge list of sudoku puzzles you can test your algorithm on.
That’s the end of your Python practice problems adventure! But if you’d like more, head on over to the video course Write and Test a Python Function: Interview Practice to see an experienced developer tackle an interview problem in real time.
Congratulations on working through this set of Python practice problems! You’ve gotten some practice applying your Python skills and also spent some time thinking about how you can respond in different interviewing situations.
In this tutorial, you learned how to:
Remember, you can download the skeleton code for these problems by clicking on the link below:
Feel free to reach out in the comments section with any questions you have or suggestions for other Python practice problems you’d like to see! Also check out our “Ace Your Python Coding Interview” Learning Path to get more resources and for boosting your Python interview skills.
Good luck with the interview!
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
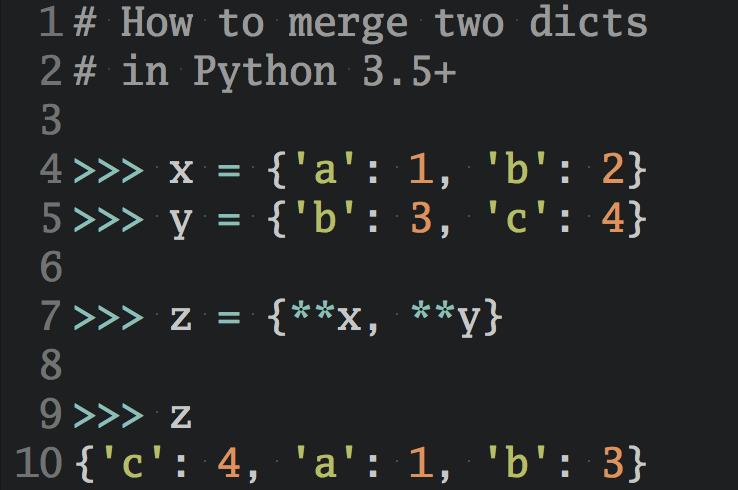
About Jim Anderson
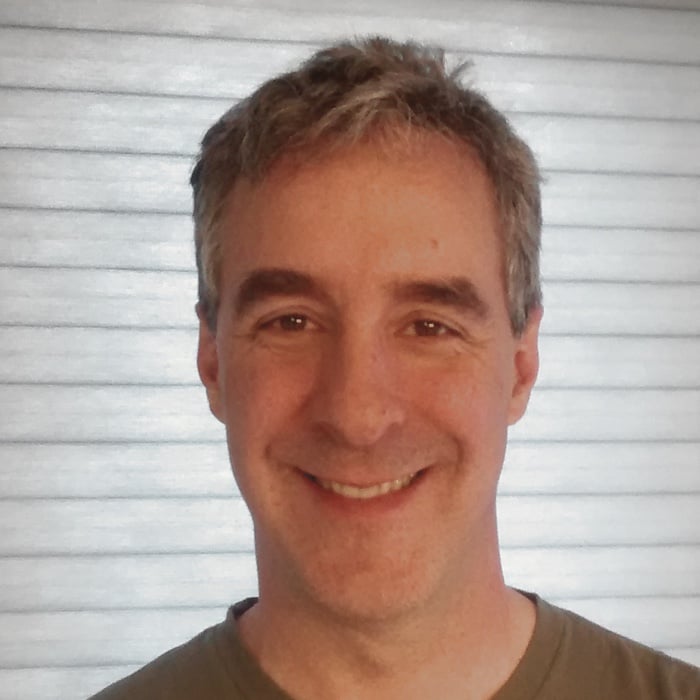
Jim has been programming for a long time in a variety of languages. He has worked on embedded systems, built distributed build systems, done off-shore vendor management, and sat in many, many meetings.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
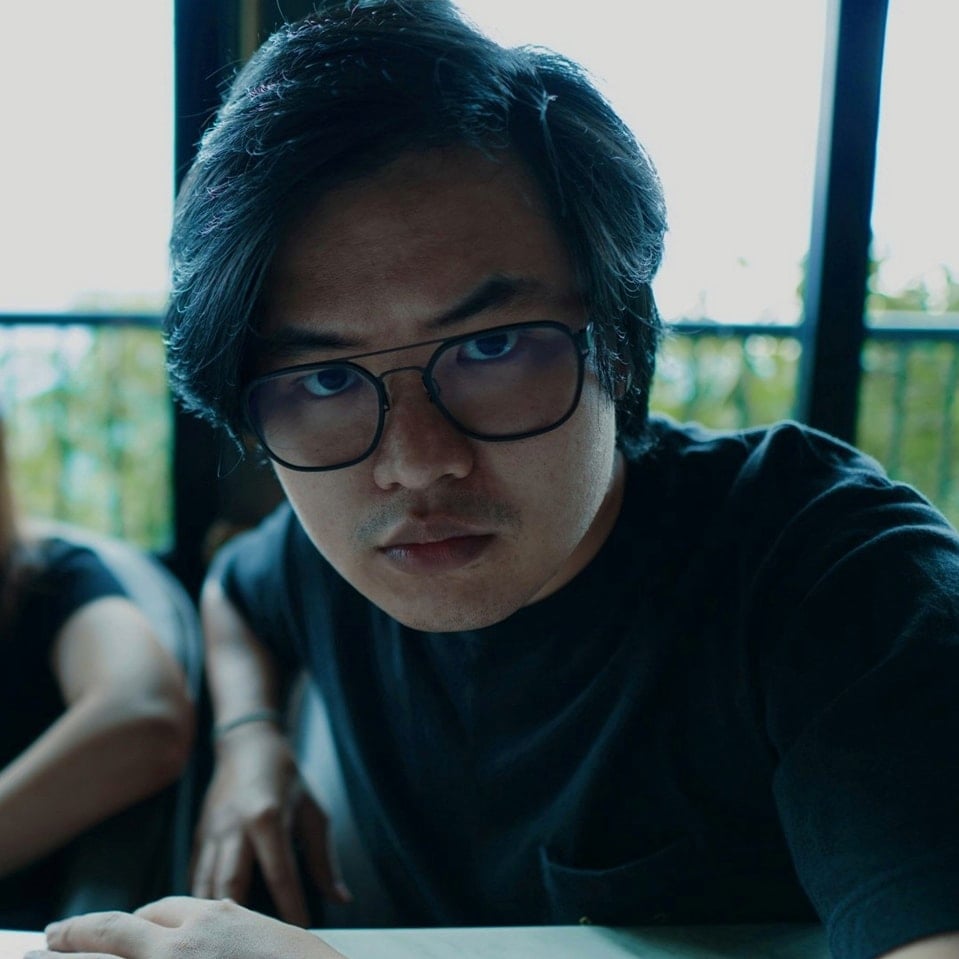
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: intermediate best-practices career
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Almost there! Complete this form and click the button below to gain instant access:
Python Practice Problems Sample Code
🔒 No spam. We take your privacy seriously.
10 Python Practice Exercises for Beginners with Solutions

- python basics
- get started with python
- online practice
A great way to improve quickly at programming with Python is to practice with a wide range of exercises and programming challenges. In this article, we give you 10 Python practice exercises to boost your skills.
Practice exercises are a great way to learn Python. Well-designed exercises expose you to new concepts, such as writing different types of loops, working with different data structures like lists, arrays, and tuples, and reading in different file types. Good exercises should be at a level that is approachable for beginners but also hard enough to challenge you, pushing your knowledge and skills to the next level.
If you’re new to Python and looking for a structured way to improve your programming, consider taking the Python Basics Practice course. It includes 17 interactive exercises designed to improve all aspects of your programming and get you into good programming habits early. Read about the course in the March 2023 episode of our series Python Course of the Month .
Take the course Python Practice: Word Games , and you gain experience working with string functions and text files through its 27 interactive exercises. Its release announcement gives you more information and a feel for how it works.
Each course has enough material to keep you busy for about 10 hours. To give you a little taste of what these courses teach you, we have selected 10 Python practice exercises straight from these courses. We’ll give you the exercises and solutions with detailed explanations about how they work.
To get the most out of this article, have a go at solving the problems before reading the solutions. Some of these practice exercises have a few possible solutions, so also try to come up with an alternative solution after you’ve gone through each exercise.
Let’s get started!
Exercise 1: User Input and Conditional Statements
Write a program that asks the user for a number then prints the following sentence that number of times: ‘I am back to check on my skills!’ If the number is greater than 10, print this sentence instead: ‘Python conditions and loops are a piece of cake.’ Assume you can only pass positive integers.
Here, we start by using the built-in function input() , which accepts user input from the keyboard. The first argument is the prompt displayed on the screen; the input is converted into an integer with int() and saved as the variable number. If the variable number is greater than 10, the first message is printed once on the screen. If not, the second message is printed in a loop number times.
Exercise 2: Lowercase and Uppercase Characters
Below is a string, text . It contains a long string of characters. Your task is to iterate over the characters of the string, count uppercase letters and lowercase letters, and print the result:
We start this one by initializing the two counters for uppercase and lowercase characters. Then, we loop through every letter in text and check if it is lowercase. If so, we increment the lowercase counter by one. If not, we check if it is uppercase and if so, we increment the uppercase counter by one. Finally, we print the results in the required format.
Exercise 3: Building Triangles
Create a function named is_triangle_possible() that accepts three positive numbers. It should return True if it is possible to create a triangle from line segments of given lengths and False otherwise. With 3 numbers, it is sometimes, but not always, possible to create a triangle: You cannot create a triangle from a = 13, b = 2, and c = 3, but you can from a = 13, b = 9, and c = 10.
The key to solving this problem is to determine when three lines make a triangle regardless of the type of triangle. It may be helpful to start drawing triangles before you start coding anything.

Notice that the sum of any two sides must be larger than the third side to form a triangle. That means we need a + b > c, c + b > a, and a + c > b. All three conditions must be met to form a triangle; hence we need the and condition in the solution. Once you have this insight, the solution is easy!
Exercise 4: Call a Function From Another Function
Create two functions: print_five_times() and speak() . The function print_five_times() should accept one parameter (called sentence) and print it five times. The function speak(sentence, repeat) should have two parameters: sentence (a string of letters), and repeat (a Boolean with a default value set to False ). If the repeat parameter is set to False , the function should just print a sentence once. If the repeat parameter is set to True, the function should call the print_five_times() function.
This is a good example of calling a function in another function. It is something you’ll do often in your programming career. It is also a nice demonstration of how to use a Boolean flag to control the flow of your program.
If the repeat parameter is True, the print_five_times() function is called, which prints the sentence parameter 5 times in a loop. Otherwise, the sentence parameter is just printed once. Note that in Python, writing if repeat is equivalent to if repeat == True .
Exercise 5: Looping and Conditional Statements
Write a function called find_greater_than() that takes two parameters: a list of numbers and an integer threshold. The function should create a new list containing all numbers in the input list greater than the given threshold. The order of numbers in the result list should be the same as in the input list. For example:
Here, we start by defining an empty list to store our results. Then, we loop through all elements in the input list and test if the element is greater than the threshold. If so, we append the element to the new list.
Notice that we do not explicitly need an else and pass to do nothing when integer is not greater than threshold . You may include this if you like.
Exercise 6: Nested Loops and Conditional Statements
Write a function called find_censored_words() that accepts a list of strings and a list of special characters as its arguments, and prints all censored words from it one by one in separate lines. A word is considered censored if it has at least one character from the special_chars list. Use the word_list variable to test your function. We've prepared the two lists for you:
This is another nice example of looping through a list and testing a condition. We start by looping through every word in word_list . Then, we loop through every character in the current word and check if the current character is in the special_chars list.
This time, however, we have a break statement. This exits the inner loop as soon as we detect one special character since it does not matter if we have one or several special characters in the word.
Exercise 7: Lists and Tuples
Create a function find_short_long_word(words_list) . The function should return a tuple of the shortest word in the list and the longest word in the list (in that order). If there are multiple words that qualify as the shortest word, return the first shortest word in the list. And if there are multiple words that qualify as the longest word, return the last longest word in the list. For example, for the following list:
the function should return
Assume the input list is non-empty.
The key to this problem is to start with a “guess” for the shortest and longest words. We do this by creating variables shortest_word and longest_word and setting both to be the first word in the input list.
We loop through the words in the input list and check if the current word is shorter than our initial “guess.” If so, we update the shortest_word variable. If not, we check to see if it is longer than or equal to our initial “guess” for the longest word, and if so, we update the longest_word variable. Having the >= condition ensures the longest word is the last longest word. Finally, we return the shortest and longest words in a tuple.
Exercise 8: Dictionaries
As you see, we've prepared the test_results variable for you. Your task is to iterate over the values of the dictionary and print all names of people who received less than 45 points.
Here, we have an example of how to iterate through a dictionary. Dictionaries are useful data structures that allow you to create a key (the names of the students) and attach a value to it (their test results). Dictionaries have the dictionary.items() method, which returns an object with each key:value pair in a tuple.
The solution shows how to loop through this object and assign a key and a value to two variables. Then, we test whether the value variable is greater than 45. If so, we print the key variable.
Exercise 9: More Dictionaries
Write a function called consonant_vowels_count(frequencies_dictionary, vowels) that takes a dictionary and a list of vowels as arguments. The keys of the dictionary are letters and the values are their frequencies. The function should print the total number of consonants and the total number of vowels in the following format:
For example, for input:
the output should be:
Working with dictionaries is an important skill. So, here’s another exercise that requires you to iterate through dictionary items.
We start by defining a list of vowels. Next, we need to define two counters, one for vowels and one for consonants, both set to zero. Then, we iterate through the input dictionary items and test whether the key is in the vowels list. If so, we increase the vowels counter by one, if not, we increase the consonants counter by one. Finally, we print out the results in the required format.

Exercise 10: String Encryption
Implement the Caesar cipher . This is a simple encryption technique that substitutes every letter in a word with another letter from some fixed number of positions down the alphabet.
For example, consider the string 'word' . If we shift every letter down one position in the alphabet, we have 'xpse' . Shifting by 2 positions gives the string 'yqtf' . Start by defining a string with every letter in the alphabet:
Name your function cipher(word, shift) , which accepts a string to encrypt, and an integer number of positions in the alphabet by which to shift every letter.
This exercise is taken from the Word Games course. We have our string containing all lowercase letters, from which we create a shifted alphabet using a clever little string-slicing technique. Next, we create an empty string to store our encrypted word. Then, we loop through every letter in the word and find its index, or position, in the alphabet. Using this index, we get the corresponding shifted letter from the shifted alphabet string. This letter is added to the end of the new_word string.
This is just one approach to solving this problem, and it only works for lowercase words. Try inputting a word with an uppercase letter; you’ll get a ValueError . When you take the Word Games course, you slowly work up to a better solution step-by-step. This better solution takes advantage of two built-in functions chr() and ord() to make it simpler and more robust. The course contains three similar games, with each game comprising several practice exercises to build up your knowledge.
Do You Want More Python Practice Exercises?
We have given you a taste of the Python practice exercises available in two of our courses, Python Basics Practice and Python Practice: Word Games . These courses are designed to develop skills important to a successful Python programmer, and the exercises above were taken directly from the courses. Sign up for our platform (it’s free!) to find more exercises like these.
We’ve discussed Different Ways to Practice Python in the past, and doing interactive exercises is just one way. Our other tips include reading books, watching videos, and taking on projects. For tips on good books for Python, check out “ The 5 Best Python Books for Beginners .” It’s important to get the basics down first and make sure your practice exercises are fun, as we discuss in “ What’s the Best Way to Practice Python? ” If you keep up with your practice exercises, you’ll become a Python master in no time!
You may also like
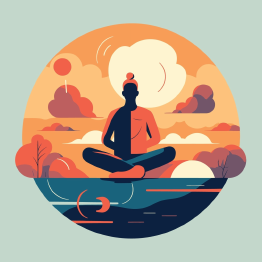
How Do You Write a SELECT Statement in SQL?
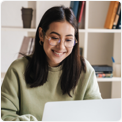
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Assignment Operators in Python
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Here, we will cover Different Assignment operators in Python .
Operators |
| ||
---|---|---|---|
| = | Assign the value of the right side of the expression to the left side operand | c = a + b |
| += | Add right side operand with left side operand and then assign the result to left operand | a += b |
| -= | Subtract right side operand from left side operand and then assign the result to left operand | a -= b |
| *= | Multiply right operand with left operand and then assign the result to the left operand | a *= b |
| /= | Divide left operand with right operand and then assign the result to the left operand | a /= b |
| %= | Divides the left operand with the right operand and then assign the remainder to the left operand | a %= b |
| //= | Divide left operand with right operand and then assign the value(floor) to left operand | a //= b |
| **= | Calculate exponent(raise power) value using operands and then assign the result to left operand | a **= b |
| &= | Performs Bitwise AND on operands and assign the result to left operand | a &= b |
| |= | Performs Bitwise OR on operands and assign the value to left operand | a |= b |
| ^= | Performs Bitwise XOR on operands and assign the value to left operand | a ^= b |
| >>= | Performs Bitwise right shift on operands and assign the result to left operand | a >>= b |
| <<= | Performs Bitwise left shift on operands and assign the result to left operand | a <<= b |
| := | Assign a value to a variable within an expression | a := exp |
Here are the Assignment Operators in Python with examples.
Assignment Operator
Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand.
Addition Assignment Operator
The Addition Assignment Operator is used to add the right-hand side operand with the left-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the addition assignment operator which will first perform the addition operation and then assign the result to the variable on the left-hand side.
S ubtraction Assignment Operator
The Subtraction Assignment Operator is used to subtract the right-hand side operand from the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the subtraction assignment operator which will first perform the subtraction operation and then assign the result to the variable on the left-hand side.
M ultiplication Assignment Operator
The Multiplication Assignment Operator is used to multiply the right-hand side operand with the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the multiplication assignment operator which will first perform the multiplication operation and then assign the result to the variable on the left-hand side.
D ivision Assignment Operator
The Division Assignment Operator is used to divide the left-hand side operand with the right-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the division assignment operator which will first perform the division operation and then assign the result to the variable on the left-hand side.
M odulus Assignment Operator
The Modulus Assignment Operator is used to take the modulus, that is, it first divides the operands and then takes the remainder and assigns it to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the modulus assignment operator which will first perform the modulus operation and then assign the result to the variable on the left-hand side.
F loor Division Assignment Operator
The Floor Division Assignment Operator is used to divide the left operand with the right operand and then assigs the result(floor value) to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the floor division assignment operator which will first perform the floor division operation and then assign the result to the variable on the left-hand side.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the exponentiation assignment operator which will first perform exponent operation and then assign the result to the variable on the left-hand side.
Bitwise AND Assignment Operator
The Bitwise AND Assignment Operator is used to perform Bitwise AND operation on both operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise AND assignment operator which will first perform Bitwise AND operation and then assign the result to the variable on the left-hand side.
Bitwise OR Assignment Operator
The Bitwise OR Assignment Operator is used to perform Bitwise OR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise OR assignment operator which will first perform bitwise OR operation and then assign the result to the variable on the left-hand side.
Bitwise XOR Assignment Operator
The Bitwise XOR Assignment Operator is used to perform Bitwise XOR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise XOR assignment operator which will first perform bitwise XOR operation and then assign the result to the variable on the left-hand side.
Bitwise Right Shift Assignment Operator
The Bitwise Right Shift Assignment Operator is used to perform Bitwise Right Shift Operation on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise right shift assignment operator which will first perform bitwise right shift operation and then assign the result to the variable on the left-hand side.
Bitwise Left Shift Assignment Operator
The Bitwise Left Shift Assignment Operator is used to perform Bitwise Left Shift Opertator on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise left shift assignment operator which will first perform bitwise left shift operation and then assign the result to the variable on the left-hand side.
Walrus Operator
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression.
Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop . The operator will solve the expression on the right-hand side and assign the value to the left-hand side operand ‘x’ and then execute the remaining code.
Please Login to comment...
Similar reads.
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Say "Hello, World!" With Python Easy Max Score: 5 Success Rate: 96.20%
Python if-else easy python (basic) max score: 10 success rate: 89.64%, arithmetic operators easy python (basic) max score: 10 success rate: 97.36%, python: division easy python (basic) max score: 10 success rate: 98.68%, loops easy python (basic) max score: 10 success rate: 98.08%, write a function medium python (basic) max score: 10 success rate: 90.30%, print function easy python (basic) max score: 20 success rate: 97.29%, list comprehensions easy python (basic) max score: 10 success rate: 97.65%, find the runner-up score easy python (basic) max score: 10 success rate: 94.20%, nested lists easy python (basic) max score: 10 success rate: 91.78%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
- Python Home
- ▼Python Exercises
- Exercises Home
- ▼Python Basic
- Basic - Part-I
- Basic - Part-II
- Python Programming Puzzles
- Mastering Python
- ▼Python Advanced
- Python Advanced Exercises
- ▼Python Control Flow
- Condition Statements and Loops
- ▼Python Data Types
- List Advanced
- Collections
- ▼Python Class
- ▼Python Concepts
- Python Unit test
- Python Exception Handling
- Python Object-Oriented Programming
- ▼Functional Programming
- Filter Function
- ▼Date and Time
- Pendulum Module
- ▼File Handling
- CSV Read, Write
- ▼Regular Expressions
- Regular Expression
- ▼Data Structures and Algorithms
- Search and Sorting
- Linked List
- Binary Search Tree
- Heap queue algorithm
- ▼Advanced Python Data Types
- Boolean Data Type
- None Data Type
- Bytes and Byte Arrays
- Memory Views exercises
- Frozenset Views exercises
- NamedTuple exercises
- OrderedDict exercises
- Counter exercises
- Ellipsis exercises
- ▼Concurrency and Threading
- Asynchronous
- ▼Python Modules
- Operating System Services
- SQLite Database
- ▼Miscellaneous
- Cyber Security
- Generators Yield
- ▼Python GUI Tkinter, PyQt
- Tkinter Home
- Tkinter Basic
- Tkinter Layout Management
- Tkinter Widgets
- Tkinter Dialogs and File Handling
- Tkinter Canvas and Graphics
- Tkinter Events and Event Handling
- Tkinter Custom Widgets and Themes
- Tkinter File Operations and Integration
- PyQt Widgets
- PyQt Connecting Signals to Slots
- PyQt Event Handling
- ▼Python NumPy
- Python NumPy Home
- ▼Python urllib3
- Python urllib3 Home
- ▼Python Metaprogramming
- Python Metaprogramming Home
- ▼Python GeoPy
- Python GeoPy Home
- ▼BeautifulSoup
- BeautifulSoup Home
- ▼Arrow Module
- ▼Python Pandas
- Python Pandas Home
- ▼Pandas and NumPy Exercises
- Pandas and NumPy Home
- ▼Python Machine Learning
- Machine Learning Home
- TensorFlow Basic
- ▼Python Web Scraping
- Web Scraping
- ▼Python Challenges
- Challenges-1
- ▼Python Mini Project
- Python Projects
- ▼Python Natural Language Toolkit
- Python NLTK
- ▼Python Project
- Novel Coronavirus (COVID-19)
- ..More to come..
- Python Exercises, Practice, Solution
What is Python language?
Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java.
Python supports multiple programming paradigms, including object-oriented, imperative and functional programming or procedural styles. It features a dynamic type system and automatic memory management and has a large and comprehensive standard library
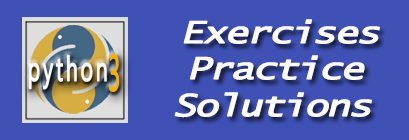
The best way we learn anything is by practice and exercise questions. We have started this section for those (beginner to intermediate) who are familiar with Python.
Hope, these exercises help you to improve your Python coding skills. Currently, following sections are available, we are working hard to add more exercises .... Happy Coding!
You may read our Python tutorial before solving the following exercises.
Latest Articles : Python Interview Questions and Answers Python PyQt
List of Python Exercises :
- Python Basic (Part -I) [ 150 Exercises with Solution ]
- Python Basic (Part -II) [ 150 Exercises with Solution ]
- Python Programming Puzzles [ 100 Exercises with Solution ]
- Mastering Python [ 150 Exercises with Solution ]
- Python Advanced [ 15 Exercises with Solution ]
- Python Conditional statements and loops [ 44 Exercises with Solution]
- Recursion [ 11 Exercises with Solution ]
- Python Data Types - String [ 113 Exercises with Solution ]
- Python JSON [ 9 Exercises with Solution ]
- Python Data Types - List [ 281 Exercises with Solution ]
- Python Data Types - List Advanced [ 15 Exercises with Solution ]
- Python Data Types - Dictionary [ 80 Exercises with Solution ]
- Python Data Types - Tuple [ 33 Exercises with Solution ]
- Python Data Types - Sets [ 30 Exercises with Solution ]
- Python Data Types - Collections [ 36 Exercises with Solution ]
- Python Array [ 24 Exercises with Solution ]
- Python Enum [ 5 Exercises with Solution ]
- Python Class [ 28 Exercises with Solution ]
- Python Unit test [ 10 Exercises with Solution ]
- Python Exception Handling [ 10 exercises with solution ]
- Python Object-Oriented Programming [ 11 Exercises with Solution ]
- Python Decorator [ 12 Exercises with Solution ]
- Python functions [ 21 Exercises with Solution ]
- Python Lambda [ 52 Exercises with Solution ]
- Python Map [ 17 Exercises with Solution ]
- Python Itertools [ 44 exercises with solution ]
- Python - Filter Function [ 11 Exercises with Solution ]
- Python Date Time [ 63 Exercises with Solution ]
- Python Pendulum (DATETIMES made easy) Module [34 Exercises with Solution]
- Python File Input Output [ 21 Exercises with Solution ]
- Python CSV File Reading and Writing [ 11 exercises with solution ]
- Python Regular Expression [ 58 Exercises with Solution ]
- Search and Sorting [ 39 Exercises with Solution ]
- Linked List [ 14 Exercises with Solution ]
- Binary Search Tree [ 6 Exercises with Solution ]
- Python heap queue algorithm [ 29 exercises with solution ]
- Python Bisect [ 9 Exercises with Solution ]
- Python Boolean Data Type [ 10 Exercises with Solution ]
- Python None Data Type [ 10 Exercises with Solution ]
- Python Bytes and Byte Arrays Data Type [ 10 Exercises with Solution ]
- Python Memory Views Data Type [ 10 Exercises with Solution ]
- Python frozenset Views [ 10 Exercises with Solution ]
- Python NamedTuple [ 9 Exercises with Solution ]
- Python OrderedDict [ 10 Exercises with Solution ]
- Python Counter [ 10 Exercises with Solution ]
- Python Ellipsis (...) [ 9 Exercises with Solution ]
- Python Multi-threading and Concurrency [ 7 exercises with solution ]
- Python Asynchronous [ 8 Exercises with Solution ]
- Python built-in Modules [ 31 Exercises with Solution ]
- Python Operating System Services [ 18 Exercises with Solution ]
- Python Math [ 94 Exercises with Solution ]
- Python Requests [ 9 exercises with solution ]
- Python SQLite Database [ 13 Exercises with Solution ]
- Python SQLAlchemy [ 14 exercises with solution ]
- Python - PPrint [ 6 Exercises with Solution ]
- Python - Cyber Security [ 10 Exercises with Solution ]
- Python Generators Yield [ 17 exercises with solution ]
- More to come
Python GUI Tkinter, PyQt
- Python Tkinter Home
- Python Tkinter Basic [ 16 Exercises with Solution ]
- Python Tkinter layout management [ 11 Exercises with Solution ]
- Python Tkinter widgets [ 22 Exercises with Solution ]
- Python Tkinter Dialogs and File Handling [ 13 Exercises with Solution ]
- Python Tkinter Canvas and Graphics [ 14 Exercises with Solution ]
- Python Tkinter Events and Event Handling [ 13 Exercises with Solution ]
- Python Tkinter Customs Widgets and Themes [ 12 Exercises with Solution ]
- Python Tkinter - File Operations and Integration [12 exercises with solution]
- Python PyQt Basic [10 exercises with solution]
- Python PyQt Widgets[12 exercises with solution]
- Python PyQt Connecting Signals to Slots [15 exercises with solution]
- Python PyQt Event Handling [10 exercises with solution]
Python Challenges :
- Python Challenges: Part -1 [ 1- 64 ]
Python Projects :
- Python Numbers : [ 11 Mini Projects with solution ]
- Python Web Programming: [ 12 Mini Projects with solution ]
- 100 Python Projects for Beginners with solution.
- Python Projects: Novel Coronavirus (COVID-19) [ 14 Exercises with Solution ]
Learn Python packages using Exercises, Practice, Solution and explanation
Python urllib3 :
- Python urllib3 [ 26 exercises with solution ]
Python Metaprogramming :
- Python Metaprogramming [ 12 exercises with solution ]
Python GeoPy Package :
- Python GeoPy Package [ 7 exercises with solution ]
Python BeautifulSoup :
- Python BeautifulSoup [ 36 exercises with solution ]
Python Arrow Module :
- Python Arrow Module [ 27 exercises with solution ]
Python Web Scraping :
- Python Web Scraping [ 27 Exercises with solution ]
Python Natural Language Toolkit :
- Python NLTK [ 22 Exercises with solution ]
Python NumPy :
- Mastering NumPy [ 100 Exercises with Solutions ]
- Python NumPy Basic [ 59 Exercises with Solution ]
- Python NumPy arrays [ 205 Exercises with Solution ]
- Python NumPy Linear Algebra [ 19 Exercises with Solution ]
- Python NumPy Random [ 17 Exercises with Solution ]
- Python NumPy Sorting and Searching [ 9 Exercises with Solution ]
- Python NumPy Mathematics [ 41 Exercises with Solution ]
- Python NumPy Statistics [ 14 Exercises with Solution ]
- Python NumPy DateTime [ 7 Exercises with Solution ]
- Python NumPy String [ 22 Exercises with Solution ]
- NumPy Broadcasting [ 20 exercises with solution ]
- NumPy Memory Layout [ 19 exercises with solution ]
- NumPy Performance Optimization [ 20 exercises with solution ]
- NumPy Interoperability [ 20 exercises with solution ]
- NumPy I/O Operations [ 20 exercises with solution ]
- NumPy Advanced Indexing [ 20 exercises with solution ]
- NumPy Universal Functions [ 20 exercises with solution ]
- NumPy Masked Arrays [ 20 exercises with solution ]
- NumPy Structured Arrays [ 20 exercises with solution ]
- NumPy Integration with SciPy [ 19 exercises with solution ]
- Advanced NumPy [ 33 exercises with solution ]
Python Pandas :
- Pandas Data Series [ 40 exercises with solution ]
- Pandas DataFrame [ 81 exercises with solution ]
- Pandas Index [ 26 exercises with solution ]
- Pandas String and Regular Expression [ 41 exercises with solution ]
- Pandas Joining and merging DataFrame [ 15 exercises with solution ]
- Pandas Grouping and Aggregating [ 32 exercises with solution ]
- Pandas Time Series [ 32 exercises with solution ]
- Pandas Filter [ 27 exercises with solution ]
- Pandas GroupBy [ 32 exercises with solution ]
- Pandas Handling Missing Values [ 20 exercises with solution ]
- Pandas Style [ 15 exercises with solution ]
- Pandas Excel Data Analysis [ 25 exercises with solution ]
- Pandas Pivot Table [ 32 exercises with solution ]
- Pandas Datetime [ 25 exercises with solution ]
- Pandas Plotting [ 19 exercises with solution ]
- Pandas Performance Optimization [ 20 exercises with solution ]
- Pandas Advanced Indexing and Slicing [ 15 exercises with solution ]
- Pandas SQL database Queries [ 24 exercises with solution ]
- Pandas Resampling and Frequency Conversion [ 15 exercises with solution ]
- Pandas Advanced Grouping and Aggregation [ 15 exercises with solution ]
- Pandas IMDb Movies Queries [ 17 exercises with solution ]
- Mastering NumPy: 100 Exercises with solutions for Python numerical computing
- Pandas Practice Set-1 [ 65 exercises with solution ]
Pandas and NumPy Exercises :
- Pandas and NumPy for Data Analysis [ 37 Exercises ]
Python Machine Learning :
- Python Machine learning Iris flower data set [38 exercises with solution]
Note : Download Python from https://www.python.org/ftp/python/3.2/ and install in your system to execute the Python programs. You can read our Python Installation on Fedora Linux and Windows 7, if you are unfamiliar to Python installation. You may accomplish the same task (solution of the exercises) in various ways, therefore the ways described here are not the only ways to do stuff. Rather, it would be great, if this helps you anyway to choose your own methods.
List of Exercises with Solutions :
- HTML CSS Exercises, Practice, Solution
- JavaScript Exercises, Practice, Solution
- jQuery Exercises, Practice, Solution
- jQuery-UI Exercises, Practice, Solution
- CoffeeScript Exercises, Practice, Solution
- Twitter Bootstrap Exercises, Practice, Solution
- C Programming Exercises, Practice, Solution
- C# Sharp Programming Exercises, Practice, Solution
- PHP Exercises, Practice, Solution
- R Programming Exercises, Practice, Solution
- Java Exercises, Practice, Solution
- SQL Exercises, Practice, Solution
- MySQL Exercises, Practice, Solution
- PostgreSQL Exercises, Practice, Solution
- SQLite Exercises, Practice, Solution
- MongoDB Exercises, Practice, Solution
More to Come !
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
[ Want to contribute to Python exercises? Send your code (attached with a .zip file) to us at w3resource[at]yahoo[dot]com. Please avoid copyrighted materials.]
Test your Python skills with w3resource's quiz
Follow us on Facebook and Twitter for latest update.
- Weekly Trends and Language Statistics
Python for Everybody
- Coursera: Python for Everybody Specialization
- edX: Python for Everybody
- FutureLearn: Programming for Everybody (Getting Started with Python)
- FreeCodeCamp
- Free certificates for University of Michigan students and staff
If you log in to this site you have joined a free, global open and online course. You have a grade book, autograded assignments, discussion forums, and can earn badges for your efforts.
We take your privacy seriously on this site, you can review our Privacy Policy for more details.
If you want to use these materials in your own classes you can download or link to the artifacts on this site, export the course material as an IMS Common Cartridge®, or apply for an IMS Learning Tools Interoperability® (LTI®) key and secret to launch the autograders from your LMS.
The code for this site including the autograders, slides, and course content is all available on GitHub . That means you could make your own copy of the course site, publish it and remix it any way you like. Even more exciting, you could translate the entire site (course) into your own language and publish it. I have provided some instructions on how to translate this course in my GitHub repository.
And yes, Dr. Chuck actually has a race car - it is called the SakaiCar . He races in a series called 24 Hours of Lemons .
Programming DSA using Python Nptel Week 2 Assignment Answers
Are you looking for the Programming, Data Structures and Algorithms using Python NPTEL Week 2 Assignment Answers 2024 ? You’re in the right place! This guide provides detailed solutions to the Week 2 assignment questions, covering key concepts in Python programming, data structures, and algorithms.
Course Link: Click Here
Table of Contents
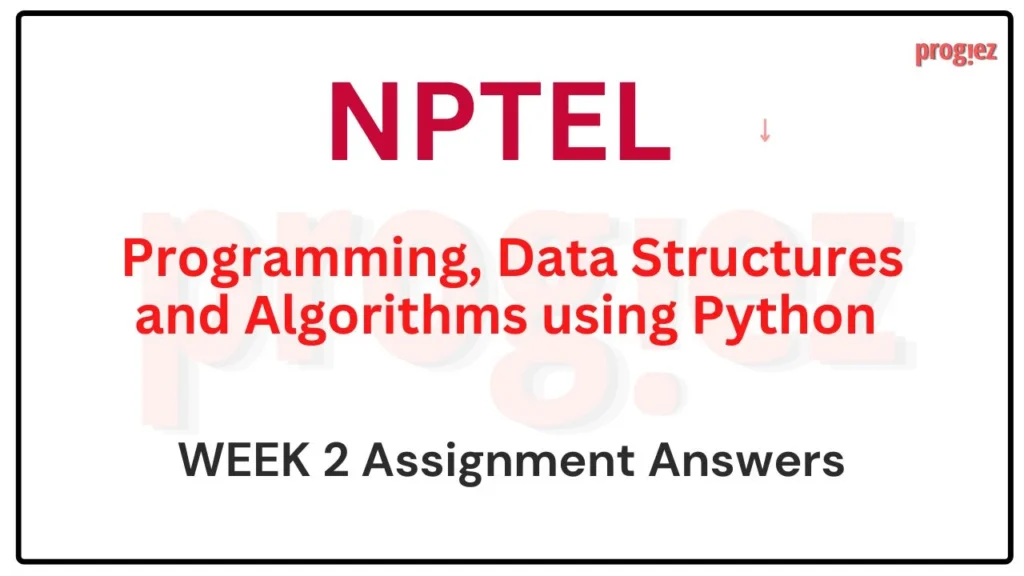
Programming DSA using Python Week 2 Assignment Answers (July-Dec 2024)
Q1.One of the following 10 statements generates an error. Which one? (Your answer should be a number between 1 and 10.)
x = [[3,5],”mimsy”,2,”borogove”,1] # Statement 1 y = x[0:50] # Statement 2 z = y # Statement 3 w = x # Statement 4 x[1] = x[1][:5] + ‘ery’ # Statement 5 y[1] = 4 # Statement 6 w[1][:3] = ‘fea’ # Statement 7 z[4] = 42 # Statement 8 x[0][0] = 5555 # Statement 9 a = (x[3][1] == 1) # Statement 10
Q2.Consider the following lines of Python code.
b = [43,99,65,105,4] a = b[2:] d = b[1:] c = b d[1] = 95 b[2] = 47 c[3] = 73
Which of the following holds at the end of this code?
a[0] == 47, b[3] == 73, c[3] == 73, d[1] == 47 a[0] == 65, b[3] == 105, c[3] == 73, d[1] == 95 a[0] == 65, b[3] == 73, c[3] == 73, d[1] == 95 a[0] == 95, b[3] == 73, c[3] == 73, d[1] == 95
Answer: a[0] == 65, b[3] == 73, c[3] == 73, d[1] == 95
For answers or latest updates join our telegram channel: Click here to join
These are Programming DSA using Python Week 2 Assignment Answers
Q3. What is the value of endmsg after executing the following lines?
startmsg = “anaconda” endmsg = “” for i in range(1,1+len(startmsg)): endmsg = endmsg + startmsg[-i]
Answer: “adnocana”
Q4. What is the value of mylist after the following lines are executed?
def mystery(l): l = l[2:] return(l)
mylist = [7,11,13,17,19,21] mystery(mylist)
Answer: [7,11,13,17,19,21]
These are Nptel Programming DSA using Python Week 2 Assignment Answers
Programming, Data Structures and Algorithms using Python NPTEL All weeks: Click Here
More Nptel Courses: https://progiez.com/nptel-assignment-answers
Programming DSA using Python Week 2 Assignment Answers (JULY-DEC 2023 )
These are Programming Data Structure And Algorithms Assignment 2 Answers
Q1. One of the following 10 statements generates an error. Which one? (Your answer should be a number between 1 and 10.) x = [“slithy”,[7,10,12],2,”tove”,1] # Statement 1 y = x[0:50] # Statement 2 z = y # Statement 3 w = x # Statement 4 x[0] = x[0][:5] + ‘ery’ # Statement 5 y[2] = 4 # Statement 6 z[4] = 42 # Statement 7 w[0][:3] = ‘fea’ # Statement 8 x[1][0] = 5555 # Statement 9 a = (x[4][1] == 1) # Statement 10
Q2. Consider the following lines of Python code. b = [23,44,87,100] a = b[1:] d = b[2:] c = b d[0] = 97 c[2] = 77 Which of the following holds at the end of this code? a[1] == 77, b[2] == 77, c[2] == 77, d[0] == 97 a[1] == 87, b[2] == 87, c[2] == 77, d[0] == 97 a[1] == 87, b[2] == 77, c[2] == 77, d[0] == 97 a[1] == 97, b[2] == 77, c[2] == 77, d[0] == 97
Answer: a[1] == 87, b[2] == 77, c[2] == 77, d[0] == 97
Q3. What is the value of endmsg after executing the following lines? startmsg = “python” endmsg = “” for i in range(1,1+len(startmsg)): endmsg = startmsg[-i] + endmsg
Answer: python
Q4. What is the value of mylist after the following lines are executed? def mystery(l): l = l[1:] return() mylist = [7,11,13] mystery(mylist)
Answer: [7,11,13]
Assignment Question s
Question 1 A positive integer m is a prime product if it can be written as p×q, where p and q are both primes. Write a Python function primeproduct(m) that takes an integer m as input and returns True if m is a prime product and False otherwise. (If m is not positive, your function should return False.) Here are some examples of how your function should work.
Write a function delchar(s,c) that takes as input strings s and c, where c has length 1 (i.e., a single character), and returns the string obtained by deleting all occurrences of c in s. If c has length other than 1, the function should return s Here are some examples to show how your function should work.
Write a function shuffle(l1,l2) that takes as input two lists, 11 and l2, and returns a list consisting of the first element in l1, then the first element in l2, then the second element in l1, then the second element in l2, and so on. If the two lists are not of equal length, the remaining elements of the longer list are appended at the end of the shuffled output. Here are some examples to show how your function should work.
More Weeks of Programming Data Structure And Algorithms Using Python: Click here
More Nptel Courses: Click here
Programming, DSA using Python Week 2 Assignment Answers (JAN-APR 2023)
Q1. One of the following 10 statements generates an error. Which one? (Your answer should be a number between 1 and 10.)
Q2. Consider the following lines of Python code.
After these execute, which of the following is correct? a. x[2] == 487, y[0] == 397, u[2] == 487, w[0] == 357 b. x[2] == 487, y[0] == 357, u[2] == 487, w[0] == 357 c. x[2] == 487, y[0] == 397, u[2] == 397, w[0] == 357 d. x[2] == 487, y[0] == 357, u[2] == 397, w[0] == 357
Answer: a. x[2] == 487, y[0] == 397, u[2] == 487, w[0] == 357
Q3. What is the value of second after executing the following lines?
Answer: ‘aedsoe’
Q4. What is the value of list1 after the following lines are executed?
Answer: [88, 53, 12, 88, 53, 97, 62]
Assignment Question
1. A positive integer m can be partitioned as primes if it can be written as p + q where p > 0, q > 0 and both p and q are prime numbers. Write a Python function primepartition(m) that takes an integer m as input and returns True if m can be partitioned as primes and False otherwise. (If m is not positive, your function should return False.) Here are some examples of how your function should work.
2. Write a function matched(s) that takes as input a string s and checks if the brackets “(” and “)” in s are matched: that is, every “(” has a matching “)” after it and every “)” has a matching “(” before it. Your function should ignore all other symbols that appear in s. Your function should return True if s has matched brackets and False if it does not. Here are some examples to show how your function should work.
3. A list rotation consists of taking the first element and moving it to the end. For instance, if we rotate the list [1,2,3,4,5], we get [2,3,4,5,1]. If we rotate it again, we get [3,4,5,1,2]. Write a Python function rotatelist(l,k) that takes a list l and a positive integer k and returns the list l after k rotations. If k is not positive, your function should return l unchanged. Note that your function should not change l itself, and should return the rotated list. Here are some examples to show how your function should work.
More Weeks of Programming Data Structure And Algorithms: Click Here
More Nptel courses: https://progiez.com/nptel
Programming DSA using Python Week 2 Assignment Answers(JULY-DEC 2022 )
Answer: c – a[0] == 65, b[3] == 73, c[3] == 73, d[1] == 95
Answer: ‘anaconda’
Assignment Answers
Q1. Write a function intreverse(n) that takes as input a positive integer n and returns the integer obtained by reversing the digits in n. Write a function matched(s) that takes as input a string s and checks if the brackets “(” and “)” in s are matched: that is, every “(” has a matching “)” after it and every “)” has a matching “(” before it. Your function should ignore all other symbols that appear in s. Your function should return True if s has matched brackets and False if it does not. Write a function sumprimes(l) that takes as input a list of integers l and retuns the sum of all the prime numbers in l.
These ar e Nptel Programming DSA using Python Week 2 Assignment Answers
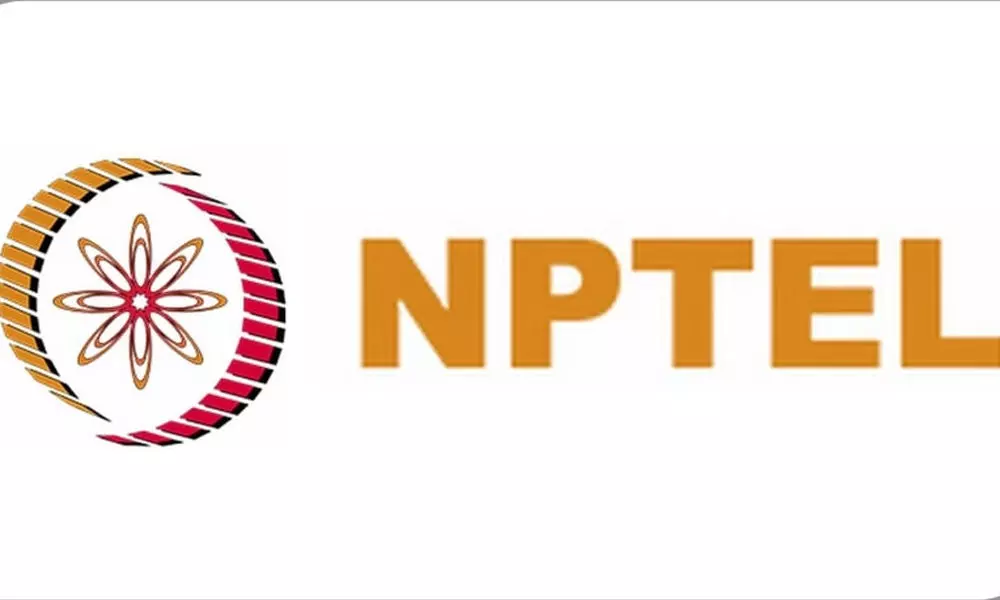
The content uploaded on this website is for reference purposes only. Please do it yourself first. |
Python Programming
Python Object-Oriented Programming (OOP) Exercise: Classes and Objects Exercises
Updated on: December 8, 2021 | 51 Comments
This Object-Oriented Programming (OOP) exercise aims to help you to learn and practice OOP concepts. All questions are tested on Python 3.
Python Object-oriented programming (OOP) is based on the concept of “objects,” which can contain data and code: data in the form of instance variables (often known as attributes or properties), and code, in the form method. I.e., Using OOP, we encapsulate related properties and behaviors into individual objects.
What is included in this Python OOP exercise?
This OOP classes and objects exercise includes 8 different programs, questions, and challenges. All solutions are tested on Python 3.
This OOP exercise covers questions on the following topics :
- Class and Object creation
- Instance variables and Methods, and Class level attributes
- Model systems with class inheritance i.e., inherit From Other Classes
- Parent Classes and Child Classes
- Extend the functionality of Parent Classes using Child class
- Object checking
When you complete each question, you get more familiar with the Python OOP. Let us know if you have any alternative solutions . It will help other developers.
Use Online Code Editor to solve exercise questions.
- Guide on Python OOP
- Inheritance in Python
Table of contents
Oop exercise 1: create a class with instance attributes, oop exercise 2: create a vehicle class without any variables and methods, oop exercise 3: create a child class bus that will inherit all of the variables and methods of the vehicle class, oop exercise 4: class inheritance, oop exercise 5: define a property that must have the same value for every class instance (object), oop exercise 6: class inheritance, oop exercise 7: check type of an object, oop exercise 8: determine if school_bus is also an instance of the vehicle class.
Write a Python program to create a Vehicle class with max_speed and mileage instance attributes.
- Classes and Objects in Python
- Instance variables in Python
Create a Bus object that will inherit all of the variables and methods of the parent Vehicle class and display it.
Expected Output:
Refer : Inheritance in Python
Create a Bus class that inherits from the Vehicle class. Give the capacity argument of Bus.seating_capacity() a default value of 50.
Use the following code for your parent Vehicle class.
Expected Output :
- Polymorphism in Python
- First, use method overriding.
- Next, use default method argument in the seating_capacity() method definition of a bus class.
Define a class attribute” color ” with a default value white . I.e., Every Vehicle should be white.
Use the following code for this exercise.
Refer : Class Variable in Python
Define a color as a class variable in a Vehicle class
Variables created in .__init__() are called instance variables . An instance variable’s value is specific to a particular instance of the class. For example, in the solution, All Vehicle objects have a name and a max_speed, but the name and max_speed variables’ values will vary depending on the Vehicle instance.
On the other hand, the class variable is shared between all class instance s. You can define a class attribute by assigning a value to a variable name outside of .__init__() .
Create a Bus child class that inherits from the Vehicle class. The default fare charge of any vehicle is seating capacity * 100 . If Vehicle is Bus instance, we need to add an extra 10% on full fare as a maintenance charge. So total fare for bus instance will become the final amount = total fare + 10% of the total fare.
Note: The bus seating capacity is 50 . so the final fare amount should be 5500. You need to override the fare() method of a Vehicle class in Bus class.
Use the following code for your parent Vehicle class. We need to access the parent class from inside a method of a child class.
Write a program to determine which class a given Bus object belongs to.
Use Python’s built-in function type() .
Use isinstance() function
Did you find this page helpful? Let others know about it. Sharing helps me continue to create free Python resources.
About Vishal

I’m Vishal Hule , the Founder of PYnative.com. As a Python developer, I enjoy assisting students, developers, and learners. Follow me on Twitter .
Related Tutorial Topics:
Python exercises and quizzes.
Free coding exercises and quizzes cover Python basics, data structure, data analytics, and more.
- 15+ Topic-specific Exercises and Quizzes
- Each Exercise contains 10 questions
- Each Quiz contains 12-15 MCQ
Loading comments... Please wait.
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Online Python Code Editor
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
infytq-assignment-solutions
Here are 7 public repositories matching this topic..., sudarshantadage / infytq-answers.
Solution of all InfyTQ Assignments, Exercise, Quiz
- Updated Nov 22, 2020
iamwatchdogs / Infytq-Programming-Fundamentals-using-Python-Part-1
Solution for all the quizzes, exercises and assignments for the Infytq's course Programming Fundamental using python part-1 in this repository.
- Updated Mar 4, 2023
ashwanisng / infyTQ-java
- Updated Jan 20, 2021
rajdip20 / InfyTQ_Problem_Solved
In this repo, I answered all the InfyTQ problems and also provided the problem statement at the beginning of the program for all.
- Updated Aug 1, 2021
2002Dhanush / INFYTQ-PYTHON-SOLUTIONS
Solutions of InfyTQ Assignments
- Updated Aug 14, 2023
- Jupyter Notebook
avibhawnani / InfytQ--Solutions
InfyTQ Answers
- Updated Apr 2, 2022
saurabhc24 / InfyTQAnswers
This repository contains solutions to quizes, exercises and assignments of InfyTQ certification.
- Updated Oct 23, 2020
Improve this page
Add a description, image, and links to the infytq-assignment-solutions topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the infytq-assignment-solutions topic, visit your repo's landing page and select "manage topics."

COMMENTS
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
Also, try to solve Python list Exercise. Exercise 7: Return the count of a given substring from a string. Write a program to find how many times substring "Emma" appears in the given string. Given: str_x = "Emma is good developer. Emma is a writer" Code language: Python (python) Expected Output: Emma appeared 2 times
In this article, I'll list down some problems that I've done and the answer code for each exercise. Analyze each problem and try to solve it by yourself. If you have any doubts, you can check the code that I've provided below. I've also attached the corresponding outputs. 1. Python program to check whether the given number is even or not.
Python exercises, practice questions, and solutions. A detailed guide with 50 plus Python practice exercises for Python developer. ... Top 50+ Python Interview Questions and Answers (Latest 2024) Python is the most used language in top companies such as Intel, IBM, NASA, Pixar, Netflix, Facebook, JP Morgan Chase, Spotify, and many more because ...
ALL Answered. Question #350996. Python. Create a method named check_angles. The sum of a triangle's three angles should return True if the sum is equal to 180, and False otherwise. The method should print whether the angles belong to a triangle or not. 11.1 Write methods to verify if the triangle is an acute triangle or obtuse triangle.
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
To run a cell of code, click the "play button" icon to the left of the cell or click on the cell and press "Shift+Enter" on your keyboard. This will execute the Python code contained in the cell. Executing a cell that defines a variable is important before executing or authoring a cell that depends on that previously created variable assignment.
We have gathered a variety of Python exercises (with answers) for each Python Chapter. Try to solve an exercise by filling in the missing parts of a code. If you're stuck, hit the "Show Answer" button to see what you've done wrong. Count Your Score. You will get 1 point for each correct answer. Your score and total score will always be displayed.
Exercise 1: Create a function in Python. Exercise 2: Create a function with variable length of arguments. Exercise 3: Return multiple values from a function. Exercise 4: Create a function with a default argument. Exercise 5: Create an inner function to calculate the addition in the following way. Exercise 6: Create a recursive function.
Beginner Python exercises. Home; Why Practice Python? Why Chilis? Resources for learners; All Exercises. 1: Character Input 2: Odd Or Even 3: List Less Than Ten 4: Divisors 5: List Overlap 6: String Lists 7: List Comprehensions 8: Rock Paper Scissors 9: Guessing Game One 10: List Overlap Comprehensions 11: Check Primality Functions 12: List Ends 13: Fibonacci 14: List Remove Duplicates
Python Crash Course on GitHub. Solutions. Solutions for selected exercises from each chapter can be found below. Be careful about looking at the solutions too quickly; make sure you've given yourself time to wrestle with the concepts you just learned before looking at a solution. Also, there are several ways to solve many of the exercises ...
Current repository contains all assignments, notes, quizzes and course materials from the "Python for Everybody Specialization" provided by Coursera and University of Michigan. - sersavn/coursera-python-for-everybody-specialization ... Each course described above contains assignments answers, course material and might contain some notes.
For this problem, you'll need to parse a log file with a specified format and generate a report: Log Parser ( logparse.py) Accepts a filename on the command line. The file is a Linux-like log file from a system you are debugging. Mixed in among the various statements are messages indicating the state of the device.
7 Python programming Assignment on external tool. The answers to each programming assignment and Quiz is uploaded in each week. ... Each Document has Screenshot of the right answer along with the questions. There may be a few exception where the answer is wrong, In those cases the answer is explicitly mentioned below the screenshot. ...
Multiple- target assignment: x = y = 75. print(x, y) In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left. OUTPUT. 75 75. 7. Augmented assignment : The augmented assignment is a shorthand assignment that combines an expression and an assignment.
Exercise 1: User Input and Conditional Statements. Write a program that asks the user for a number then prints the following sentence that number of times: 'I am back to check on my skills!'. If the number is greater than 10, print this sentence instead: 'Python conditions and loops are a piece of cake.'.
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression. Syntax: a := expression. Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop.
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews.
Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java. Python supports multiple programming paradigms, including object-oriented ...
Coursera: Python for Everybody Specialization; edX: Python for Everybody; FreeCodeCamp; Free certificates for University of Michigan students and staff; CodeKidz; If you log in to this site you have joined a free, global open and online course. You have a grade book, autograded assignments, discussion forums, and can earn badges for your efforts.
This Python loop exercise include the following: -. It contains 18 programs to solve using if-else statements and looping techniques.; Solutions are provided for all questions and tested on Python 3. This exercise is nothing but an assignment to solve, where you can solve and practice different loop programs and challenges.
Are you looking for NPTEL Week 1 assignment answers for 2024 for July Dec Session ! If you're enrolled in any of the NPTEL courses, this post will help you find the relevant assignment answers for Week 1. Ensure to submit your assignments by August 8, 2024.
Assignment Questions. Question 1 A positive integer m is a prime product if it can be written as p×q, where p and q are both primes. Write a Python function primeproduct(m) that takes an integer m as input and returns True if m is a prime product and False otherwise.
This Object-Oriented Programming (OOP) exercise aims to help you to learn and practice OOP concepts. All questions are tested on Python 3. Python Object-oriented programming (OOP) is based on the concept of "objects," which can contain data and code: data in the form of instance variables (often known as attributes or properties), and code, in the form method.
To associate your repository with the infytq-assignment-solutions topic, visit your repo's landing page and select "manage topics." GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.