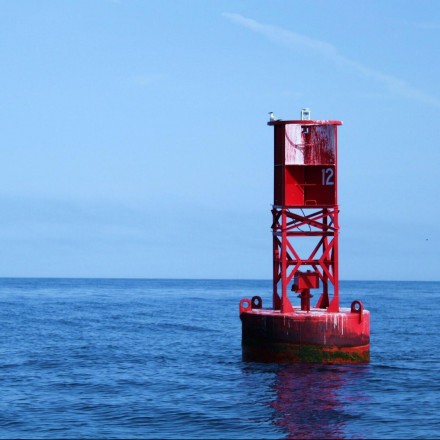

Posts · Projects
Assigning students to rank-ordered group projects with linear optimization.
It is fairly common in a classroom setting to have students who must be assiged to projects, either to work alone or in groups. If the students have each ranked the projects from most to least desirable, one typically wants to assign all of the students to their most desired projects.
This is a perfect scenario for optimization, in that we can come up with a "satisfaction" metric based on the rankings and let the solver try to find the best configuration. In this post, we will set up the problem using the proprietary Gurobi solver via their Python bindings , but it can be just as easily set up using PuLP or OR-Tools .
Here, we will consider the problem of assigning students to groups of at most 4 for projects that they have ranked from 1 (most desirable) up to the number projects, and we will try to make everyone as happy as possible in the resulting solution. The data is anonymized but is real data from a recent class (though slightly modified to make the problem harder).
Input the data ¶
Here's some data that was collected using a web form asking each student to rate each of the nine projects from 1 (most desired) to 9 (least desired). In our case, the form required a complete ordering from each student, but if that was not available we could easily create a constraint that prevents students from being assigned to a project they wanted so little that they refused to rate it.
Project 00 | Project 01 | Project 02 | Project 03 | Project 04 | Project 05 | Project 06 | Project 07 | Project 08 | |
---|---|---|---|---|---|---|---|---|---|
Student 00 | 6 | 4 | 1 | 9 | 8 | 2 | 7 | 5 | 3 |
Student 01 | 1 | 2 | 5 | 3 | 7 | 6 | 9 | 4 | 8 |
Student 02 | 4 | 5 | 6 | 8 | 9 | 3 | 2 | 1 | 7 |
Student 03 | 2 | 4 | 3 | 7 | 9 | 1 | 5 | 8 | 6 |
Student 04 | 3 | 2 | 5 | 1 | 9 | 8 | 4 | 6 | 7 |
Student 05 | 2 | 1 | 7 | 4 | 9 | 8 | 6 | 3 | 5 |
Student 06 | 5 | 2 | 3 | 1 | 9 | 4 | 8 | 7 | 6 |
Student 07 | 4 | 1 | 7 | 2 | 9 | 3 | 6 | 5 | 8 |
Student 08 | 8 | 6 | 3 | 5 | 2 | 4 | 7 | 1 | 9 |
Student 09 | 2 | 6 | 7 | 3 | 5 | 1 | 4 | 9 | 8 |
Student 10 | 1 | 5 | 3 | 4 | 9 | 7 | 2 | 8 | 6 |
Student 11 | 4 | 5 | 8 | 7 | 9 | 3 | 2 | 1 | 6 |
Student 12 | 7 | 5 | 8 | 1 | 6 | 9 | 4 | 3 | 2 |
Student 13 | 4 | 5 | 2 | 9 | 8 | 1 | 3 | 7 | 6 |
Student 14 | 3 | 5 | 2 | 4 | 8 | 1 | 9 | 6 | 7 |
Student 15 | 1 | 3 | 4 | 2 | 8 | 5 | 7 | 6 | 9 |
And here are the students who have indicated that they would specifically like to be together with or apart from another students.
Setting up the optimization ¶
This setup naturally leads to a classic assignment ILP (integer linear program), where each decision variable is a one (assign a certain student to a certain project) or a zero (not assigned). Here's how the ILP breaks down:
Data ¶
- List of students indexed by $i \in I$
- List of projects indexed by $j \in J$
- Student preferences $\mathrm{rank}(i,j) \in {1, \ldots, J}$ available $\forall i \in I,\, j \in J$ or else assumed to be infeasible (set equal to 100)
Decision variables ¶
- Assignment matrix (binary):
Constraints ¶
- Each student is assigned to one and only one project
- Each project has less than or equal to four students
If two students mutually request on another, they are put in the same group.
If any student requests not to work with another, they are not put together.
The number of projects is less than or equal to some value $P$ (see below for discussion).
Objective function ¶
Place students in their most desired project, if possible. Higher (worse) preference rank results in a squared loss (making lower places much worse).
- student $i$ gets 1st choice $\implies Z_i = 0^2 = 0$
- student $i$ gets 2nd choice $\implies Z_i = 1^2 = 1$
- student $i$ gets 3rd choice $\implies Z_i = 2^2 = 4$
Implementation ¶
Given the problem definition above, we can start to set this up with code. Gurobi has a multidict object which allows for convenient summing and other relevant operations by indices:
The first object returned is a list of all of indices of decision variables, basically the $i$ and $j$ for each $X_{ij}$ but with the Python-native string keys instead of the actual indexes:
The ratings part is the same dictionary comprehension defined above that has all of these permutations as dict keys and then the currently assigned values as the dict values, except as a multidict it now has some extra functions that come in handy for defining the ILP:
Now we'll use the Gurobi built-ins to set this up. Here's the twist — above we set a maximum number of projects $P$, except we're going to put the problem solving inside a function and let that be the one variable we specifcy dynamically.
The motivation for this is that you can imagine having a soft constraint on the number of projects while still having a preference for fewer, so it could be helpful to see a few scenarios of number of projects and how that affects the objective function.
This could be set as a variable in the optimization, but in many assignment problems there is value to putting a "human in the loop" so we can make informed decisions about whether it's worth having some groups of three and one extra project with only two if that means everyone is much happier about their assignments.
A first pass ¶
Let's solve this for a maximum of 4 projects:
This solved almost immediately, and in fairness this problem is small enough that a person could get pretty close to optimal just going by hand. But you can probably imagine how hard this would be for a person to do once the class size got to be in the dozens of students. Meanwhile, the solver will still be very fast for almost any reasonably sized classroom.
The solve function gives us back a model object and an assignment dictionary, but we'll set up a function to turn this into some helpful dataframes:
Project 00 | Project 01 | Project 02 | Project 03 | Project 04 | Project 05 | Project 06 | Project 07 | Project 08 | |
---|---|---|---|---|---|---|---|---|---|
Student 00 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 |
Student 01 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Student 02 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
Student 03 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 |
Student 04 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Student 05 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Student 06 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Student 07 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Student 08 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
Student 09 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 |
Student 10 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Student 11 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
Student 12 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
Student 13 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Student 14 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 |
Student 15 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
So most students got their first choice, a few got their second, and then a couple students were lower than that.
Meanwhile, as specified this time around we only allowed four of the projects to be used, so with 16 students each project ended up with the maximum number of students.
Could we get better assignments if we allow another project instead of dense packing students into the maximum group size of 4 for each of 4 projects?
Solving again with a bit more slack ¶
This way we have demonstrated that going from 4 to 5 projects spreads the students out without causing much improvement in satisfaction. At this point, a human instructor could decide whether it is worthwhile or not to take on supervising another project.
Conclusion ¶
This is the type of problem that fits neatly into an optimization approach. It is extremely difficult to do by hand once the problem size grows past a handful of decision variables — try looking at the rank dataframe above and thinking about how you would do this manually if necessary. Meanwhile it is trivial to represent and solve as an ILP.
The approach can easily be extended to a situation where only one student can work on a given "thing" they have ranked and there are at least as many of the assignable things as students. For example, instead of groups they might have ranked physical resources or project topics they want to work on.
In the real world, assignment problems like this often need a "fudge factor." After all, you can optimize a problem like nurse scheduling or project assignment to 4 decimal places in the objective function, but people do messy things like calling in sick or dropping classes, and in practice our objective function isn't really one-to-one with actual happiness. Tiny changes rarely matter.
A better strategy than coming up with one perfect solution is to jump past all the hard work of figuring this out on paper but then put a human in the loop by showing a nunber of feasible and nearly-optimal solutions and let a person decide based on the totality of the circumstances.
Next: Targeting lead service line projects to minimize childhood exposure
Previous: Dirichlet-multinomial model for Skittle proportions
Any comments or suggestions? Let me know .
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Use MIQP to improve the group assignment of college computer science courses
xiaohk/CS524-Group-Assignment-Optimization
Folders and files.
Name | Name | |||
---|---|---|---|---|
12 Commits | ||||
Repository files navigation
Cs 524 (intro to optimization) final project.
In the notebook , I designed a flexible and robust Mixed Integer Quadratic Programming model in Julia to solve college course group assignment problems.
CS 506 Group Assignment Optimization
I specialized my goal to improve CS 506 project assignment experience. The objective is to maximize the student happiness and group fairness. Through visualization, I gained many insights into the optimal solution and the domain problem.
Fortunately, the project got the highest grades in terms of "originality, creativity, insightful commentary, educational value, and general awesomeness." I am also honored to have a free lunch with our professor.
- To run all cells in the notebook , you want to have Gurobi correctly installed.
- Recommend to use nbviewer for better online rendering of this project.
- Jupyter Notebook 98.4%
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
group membership assignment by preferences - optimization problem?
I have 30 users and 7 groups. All users will be members of exactly 2 groups. Each group must have between 4 and 6 members. The users have ordered their preferences for group membership (i.e., users have listed in order the groups they would like to be apart of). I would like a solution that assigns all users to groups that minimizes the total distance of all users from their top group preference.
I am trying to find a solution to this problem that is not a brute force calculation (as the search space is quite large). Is there a way to pose this problem such that it would be solvable by some optimization technique? It appears to be non-linear and non-smooth, which reduces the types of solutions, but I still can't figure out how to write the constraints. Or is there an entirely better way to solve this?
- optimization
- $\begingroup$ This sounds like one of those problems where you may have to take some naive algorithm, accept that its result won't be optimal, but prove that its result is somehow decent. $\endgroup$ – 2'5 9'2 Commented Apr 17, 2012 at 7:01
- $\begingroup$ Yes, I'm willing to accept a 'good enough' solution. $\endgroup$ – maddyblue Commented Apr 17, 2012 at 15:15
- $\begingroup$ The Hungarian method ( en.wikipedia.org/wiki/Hungarian_algorithm ) might to be applicable, which runs in polynomial time. Not 100% sure, it might need some adaptation. Don't have time to get my head around... $\endgroup$ – B0rk4 Commented Apr 19, 2012 at 17:06
Don't know if you're still looking for a solution, but it is a straight forward 0/1/ assignment model. 210 dec variables, 30 constraints for each user, 14 constraints (2 for each group) for groups, objective function is simply the (7-order) and it is a maximization.
Problem with your objective function is that there will likely be alternative optimal solutions given that the measure of preference is ordinal - there are ways in which we might implement different objectives for 'fairness' - minimize the maximum difference from the most preferred for all users, etc.
If you have the preference data in a "matrix"-style on a spreadsheet, I can get you a solution within minutes.
Interesting problem. Fish Doc
You must log in to answer this question.
Not the answer you're looking for browse other questions tagged optimization ..
- Featured on Meta
- Upcoming sign-up experiments related to tags
Hot Network Questions
- Specific calligraphic font for lowercase g
- Have there been any scholarly attempts and/or consensus as regards the missing lines of "The Ruin"?
- Is there any legal justification for content on the web without an explicit licence being freeware?
- Do capacitor packages make a difference in MLCCs?
- Can I route audio from a macOS Safari PWA to specific speakers, different from my system default?
- Summation not returning a timely result
- Correlation for Small Dataset?
- How can I take apart a bookshelf?
- Why can't LaTeX (seem to?) Support Arbitrary Text Sizes?
- What is the term for when a hyperlink maliciously opens different URL from URL displayed when hovered over?
- Do known physical systems all have a unique time evolution?
- What type of black color text for brochure print in CMYK?
- Do S&P 500 funds run by different investment companies have different performance based on the buying / selling speed of the company?
- Should I accept an offer of being a teacher assistant without pay?
- Determine the voltages Va and V1 for the circuit
- Summation of arithmetic series
- Are Dementors found all over the world, or do they only reside in or near Britain?
- Why depreciation is considered a cost to own a car?
- What is the original source of this Sigurimi logo?
- How would I say the exclamation "What a [blank]" in Latin?
- Was BCD a limiting factor on 6502 speed?
- Con permiso to enter your own house?
- What kind of sequence is between an arithmetic and a geometric sequence?
- Does it matter if a fuse is on a positive or negative voltage?
IEEE Account
- Change Username/Password
- Update Address
Purchase Details
- Payment Options
- Order History
- View Purchased Documents
Profile Information
- Communications Preferences
- Profession and Education
- Technical Interests
- US & Canada: +1 800 678 4333
- Worldwide: +1 732 981 0060
- Contact & Support
- About IEEE Xplore
- Accessibility
- Terms of Use
- Nondiscrimination Policy
- Privacy & Opting Out of Cookies
A not-for-profit organization, IEEE is the world's largest technical professional organization dedicated to advancing technology for the benefit of humanity. © Copyright 2024 IEEE - All rights reserved. Use of this web site signifies your agreement to the terms and conditions.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Group assignment problem with optimisation methods
There’s a company organising a seminar, where 60 trainees will attend. The company plans to divide the participants into 10 groups, each of 6 trainees. Each of the trainees was asked beforehand to choose 5 other trainees they would like to work with. And each of the five is weighted equally. The problem is how we can assign the participants into groups so that the total satisfaction could to optimised.
- optimization

- define total satisfaction please – juvian Commented Oct 29, 2018 at 18:49
- if a trainee were assigned to work with another one who he/she liked (prefer) to, then 1 point is added to the satisfaction. if not, then 0. – Jørgen Andreasen Commented Oct 29, 2018 at 19:14
- What have you tried so far? – G_B Commented Oct 29, 2018 at 23:18
let me give you a small example with CPO within CPLEX in OPL:
- Could you please explain a bit more about how come it is modulo operation here? int wishes[t in trainees][w in likes]=(t+w-1) mod nbTrainees+1; Thanks. – Jørgen Andreasen Commented Oct 31, 2018 at 20:00
- This helped me enter values without too much effort: friends of 1 : 2,3,4,5,6 and firiends of 60 : 1,2,3,4,5 – Alex Fleischer Commented Oct 31, 2018 at 20:53
- And how should I interpret x[t][g]*x[wishes[t][w]][g] in the optimisation function? I am quite confused about the latter part. – Jørgen Andreasen Commented Nov 1, 2018 at 19:50
- x[t][g]*x[wishes[t][w]][g] is 1 iff both factors are 1 which mean trainee t is in group g and his friend wishes[t][w] is also in group g – Alex Fleischer Commented Nov 2, 2018 at 8:05
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged optimization cplex ampl lindo or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- Policy: Generative AI (e.g., ChatGPT) is banned
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- Duplicating Matryoshka dolls
- add an apostrophe to equation number having a distant scope
- Do I need to indicate 'solo' for wind/brass instruments in shared staff?
- Is it unfair to retroactively excuse a student for absences?
- Visit USA via land border for French citizen
- Why was the animal "Wolf" used in the title "The Wolf of Wall Street (2013)"?
- How do guitarists remember what note each string represents when fretting?
- Were there engineers in airship nacelles, and why were they there?
- Is the FOCAL syntax for Alphanumeric Numbers ("0XYZ") documented anywhere?
- Was Paul's Washing in Acts 9:18 a Ritual Purification Rather Than a Christian Baptism?
- What is the term for when a hyperlink maliciously opens different URL from URL displayed when hovered over?
- Can I get a refund for ICE due to cancelled regional bus service?
- Rear shifter cable wont stay in anything but the highest gear
- Are both vocal cord and vocal chord correct?
- Both my adult kids and their father (ex husband) dual citizens
- Tombs of Ancients
- Why is it 'capacité d'observation' (without article) but 'sens de l'observation' (with article)?
- Predictable Network Interface Names: ensX vs enpXsY
- Does this double well potential contradict the fact that there is no degeneracy for one-dimensional bound states?
- How can a landlord receive rent in cash using western union
- Weird topology shading in viewport
- Determine the voltages Va and V1 for the circuit
- No simple group or order 756 : Burnside's proof
- Where does someone go with Tzara'as if they are dwelling in a Ir Miklat?
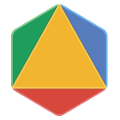
- Google OR-Tools
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Solving an Assignment Problem
This section presents an example that shows how to solve an assignment problem using both the MIP solver and the CP-SAT solver.
In the example there are five workers (numbered 0-4) and four tasks (numbered 0-3). Note that there is one more worker than in the example in the Overview .
The costs of assigning workers to tasks are shown in the following table.
Worker | Task 0 | Task 1 | Task 2 | Task 3 |
---|---|---|---|---|
90 | 80 | 75 | 70 | |
35 | 85 | 55 | 65 | |
125 | 95 | 90 | 95 | |
45 | 110 | 95 | 115 | |
50 | 100 | 90 | 100 |
The problem is to assign each worker to at most one task, with no two workers performing the same task, while minimizing the total cost. Since there are more workers than tasks, one worker will not be assigned a task.
MIP solution
The following sections describe how to solve the problem using the MPSolver wrapper .
Import the libraries
The following code imports the required libraries.
Create the data
The following code creates the data for the problem.
The costs array corresponds to the table of costs for assigning workers to tasks, shown above.
Declare the MIP solver
The following code declares the MIP solver.
Create the variables
The following code creates binary integer variables for the problem.
Create the constraints
Create the objective function.
The following code creates the objective function for the problem.
The value of the objective function is the total cost over all variables that are assigned the value 1 by the solver.
Invoke the solver
The following code invokes the solver.
Print the solution
The following code prints the solution to the problem.
Here is the output of the program.
Complete programs
Here are the complete programs for the MIP solution.
CP SAT solution
The following sections describe how to solve the problem using the CP-SAT solver.
Declare the model
The following code declares the CP-SAT model.
The following code sets up the data for the problem.
The following code creates the constraints for the problem.
Here are the complete programs for the CP-SAT solution.
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2023-01-02 UTC.
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Assignment based on ranked preference
Assume that there are n students, who have to be evenly assigned to m groups. For every student, a preference ranking of of the m groups is given.
I partially order assignment by pointwise preference, i.e. one is better or equal to another if for every student, the assigned group is ranked higher or equal.
What algorithm can I use find “locally optimal” solutions, i.e. assignments where there are no strictly better solutions?
I assume there will be multiple locally optimal solutions. Is there a sensible way to order them without giving the students an incentive to be dishonest in their ranking, i.e. without encouraging strategic voting? If so, can that be solved?
And finally: What are the right terms to search for research that solves this and related problems?
- optimization
- combinatorics
- $\begingroup$ Check out the "stable marriage" problem. $\endgroup$ – Tom van der Zanden Commented Nov 6, 2015 at 12:30
- $\begingroup$ Right, that’s related. I’ll see what I can find starting from there. $\endgroup$ – Joachim Breitner Commented Nov 6, 2015 at 12:49
- 1 $\begingroup$ Did you end up solving this problem in a satisfiable manner? It appears to be a non-trivial step to go from the SMP to this particular problem. $\endgroup$ – Joost Commented Mar 24, 2016 at 15:19
- $\begingroup$ I did not solve it at all, sorry. $\endgroup$ – Joachim Breitner Commented Mar 24, 2016 at 17:30
- $\begingroup$ This post describes a similar problem and might be of use to you. $\endgroup$ – Pim Commented Feb 23, 2021 at 21:08
The terms you can use to search the literature are stable marriage and the assignment problem .
As far as discouraging strategic voting, one relevant term might be strategy-proof . This might also be relevant to the general area of mechanism design . My vague recollection is that discouraging strategic voting in stable-marriage-like problems is a hard problem, but I don't know if I'm remembering that right.
For instance, consider stable marriage. The traditional algorithm gives a matching that is male-optimal and female-pessimal (every male gets the best possible mate he possibly could have received in any stable matching; and every female gets the worst possible mate she could have received in any stable matching). Now suppose the females all get together and collude. In a world of perfect information, they can compute what the female-optimal matching would be, and then change their reported preferences to ensure that the female-optimal matching will be selected.
In fact, collusion is not necessary -- the same result happens if each female individually acts in their own self-interest, without any cooperation or conspiracy. Assume again a world of perfect information, where everyone's true preferences are known (to the females, at least). Then each female can compute the best possible mate she could hope for, i.e., the best mate out of all possible matches in all possible stable matchings; she can then change her reported preferences to list that male as her first preference. If the men use their true preferences and each woman uses this procedure to select her preferences strategically, and then we run the traditional algorithm, we end up with the female-optimal (and male-pessimal) matching. Each woman has an incentive to behave in this way, and no collusion is necessary (assuming perfect information).

Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged optimization combinatorics matching voting or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
Hot Network Questions
- Navigation on Mars without Martian GPS
- D&D 3.5 Shadow Magic / Shadow Conjuration: Can an illusionist create a quasi-real shadow of ANY monster? Or only those on the summon monster lists?
- Do capacitor packages make a difference in MLCCs?
- Why can't LaTeX (seem to?) Support Arbitrary Text Sizes?
- Google Search Console reports "Page with redirect" as "errors", are they?
- Why depreciation is considered a cost to own a car?
- Where can I access records of the 1947 Superman copyright trial?
- Is there any legal justification for content on the web without an explicit licence being freeware?
- Is it unfair to retroactively excuse a student for absences?
- What does ‘a grade-hog’ mean?
- Was BCD a limiting factor on 6502 speed?
- Rear shifter cable wont stay in anything but the highest gear
- Why we use trace-class operators and bounded operators in quantum mechanics?
- Do known physical systems all have a unique time evolution?
- Could space habitats have large transparent roofs?
- Integration of the product of two exponential functions
- Folk stories and notions in mathematics that are likely false, inaccurate, apocryphal, or poorly founded?
- Does Matthew 7:13-14 contradict Luke 13:22-29?
- Can you help me to identify the aircraft in a 1920s photograph?
- Summation of arithmetic series
- Duplicating Matryoshka dolls
- Were there engineers in airship nacelles, and why were they there?
- How would I say the exclamation "What a [blank]" in Latin?
- Can you arrange 25 whole numbers (not necessarily all different) so that the sum of any three successive terms is even but the sum of all 25 is odd?
Help | Advanced Search

Computer Science > Machine Learning
Title: go4align: group optimization for multi-task alignment.
Abstract: This paper proposes \textit{GO4Align}, a multi-task optimization approach that tackles task imbalance by explicitly aligning the optimization across tasks. To achieve this, we design an adaptive group risk minimization strategy, compromising two crucial techniques in implementation: (i) dynamical group assignment, which clusters similar tasks based on task interactions; (ii) risk-guided group indicators, which exploit consistent task correlations with risk information from previous iterations. Comprehensive experimental results on diverse typical benchmarks demonstrate our method's performance superiority with even lower computational costs.
Subjects: | Machine Learning (cs.LG); Computer Vision and Pattern Recognition (cs.CV) |
Cite as: | [cs.LG] |
(or [cs.LG] for this version) | |
Focus to learn more arXiv-issued DOI via DataCite |
Submission history
Access paper:.
- HTML (experimental)
- Other Formats
References & Citations
- Google Scholar
- Semantic Scholar
BibTeX formatted citation

Bibliographic and Citation Tools
Code, data and media associated with this article, recommenders and search tools.
- Institution
arXivLabs: experimental projects with community collaborators
arXivLabs is a framework that allows collaborators to develop and share new arXiv features directly on our website.
Both individuals and organizations that work with arXivLabs have embraced and accepted our values of openness, community, excellence, and user data privacy. arXiv is committed to these values and only works with partners that adhere to them.
Have an idea for a project that will add value for arXiv's community? Learn more about arXivLabs .
- SMP WEDDINGS
- SMP’S VENUE BLOG
- LITTLE BLACK BOOK BLOG
- LBB INDUSTRY BLOG
- DESTINATION
- NEW ENGLAND
- THE NORTHWEST
- THE MIDWEST
- THE SOUTHWEST
- THE SOUTHEAST
- THE MID-ATLANTIC
- THE TRI-STATE AREA
Russia Weddings
- Real Weddings
- Bridal Week
- Engagements & Proposals
- Seasonal Wedding Trends
- Bridal Beauty
- Wedding Fashion Trends
- Vendor Guide
- DIY Projects
- Registry Guide
- Engagements & Proposals
- by Melissa Hammam
- comments ( )
Hands up if you’re ready to be dazzled! From a ceremony structure designed to float on water to a jaw-dropping reception room with flowers blooming from every service, we’re swooning over every bit of this wedding. If you can believe it, that’s just the beginning. Julia Kaptelova artfully shot every detail, like the ballet performance guests were treated to and snow falling from the ceiling for the first dance! Prepare to be amazed and take a visit to the full gallery .
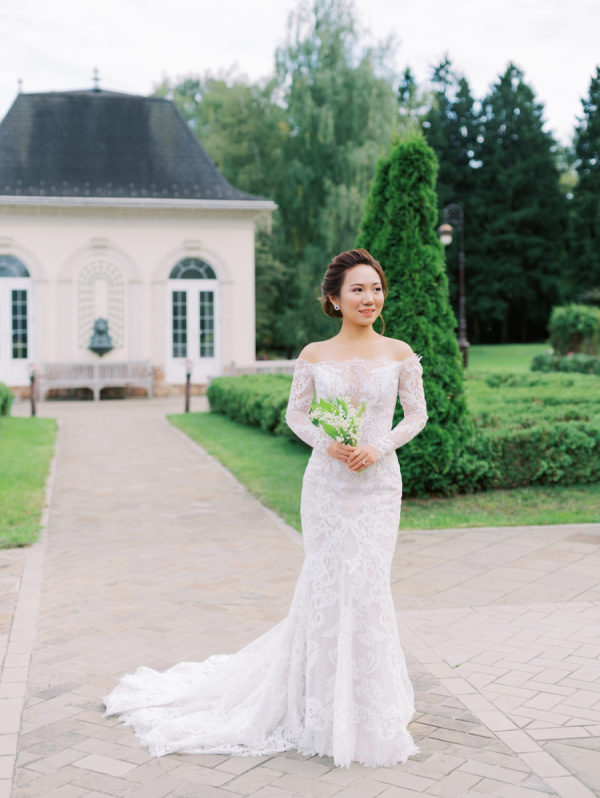
From About You Decor … Our design is a symbol of dawn and a distant endless horizon. Ahead is a long, happy life without any borders. An international couple, Pavel and Cherry, met in London and have been walking together for many years.
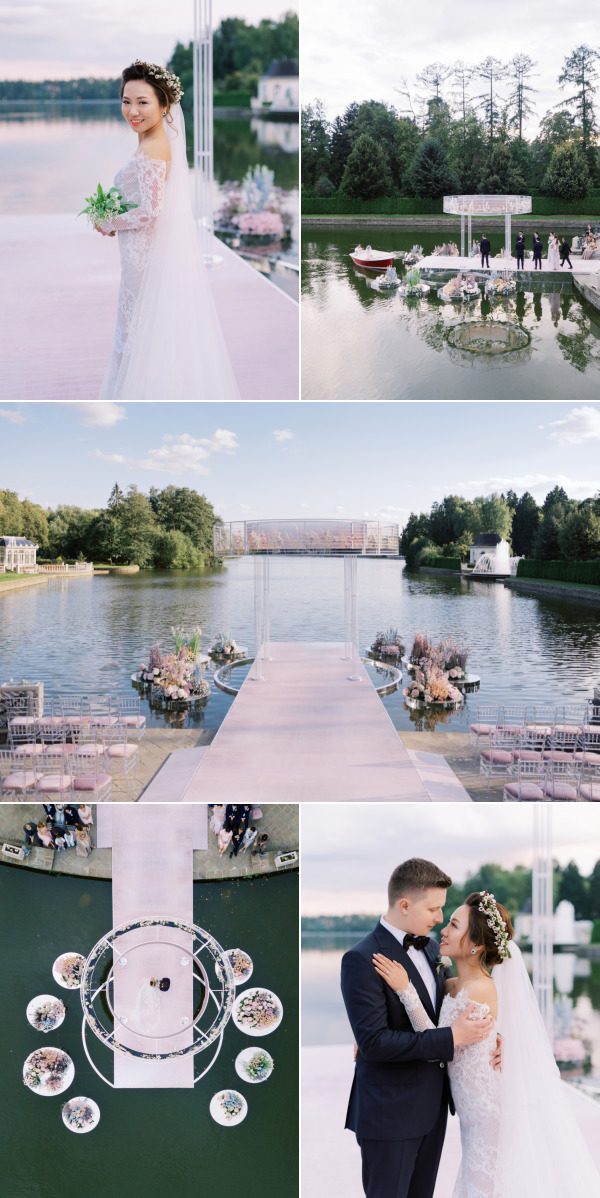
From the Bride, Cherry… My husband and I we decided to have our summer wedding in Moscow because the city is where his roots are. As we knew we were going to have the other wedding ceremony in China, we wanted our Moscow one to be very personal and intimate. We’ve known each other since we were fourteen, together with many of our friends whom we’ve also known for a decade.
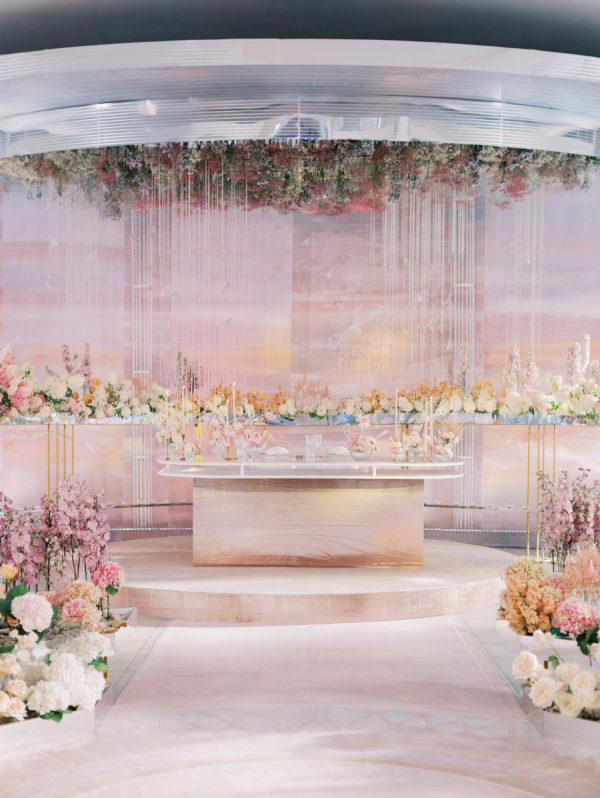
I didn’t want to walk down the aisle twice so the plausibility of my request quickly came into discussion. The open pontoon stage was constructed in order to facilitate the bridal entrance on water, although there were concerns about safety as the last thing we wanted was probably a drowned bride before she could get on stage, picture that! I have to say on that day it wasn’t easy to get on the pontoon stage from the boat, in my long gown and high heels. Luckily my bridesmaids still noticed even though they stood the furthest from me on the stage, and helped me out without prior rehearsal. My girls could just tell whenever I needed a hand or maybe they were just so used to my clumsiness. Who knows 😂
We all love our photographer Julia! She’s so talented and her style is so unique. Our beloved host Alex is exceptional who made everyone laugh and cry. It was truly a blessing to have so many kind and beautiful souls on our big day. Thank you all!
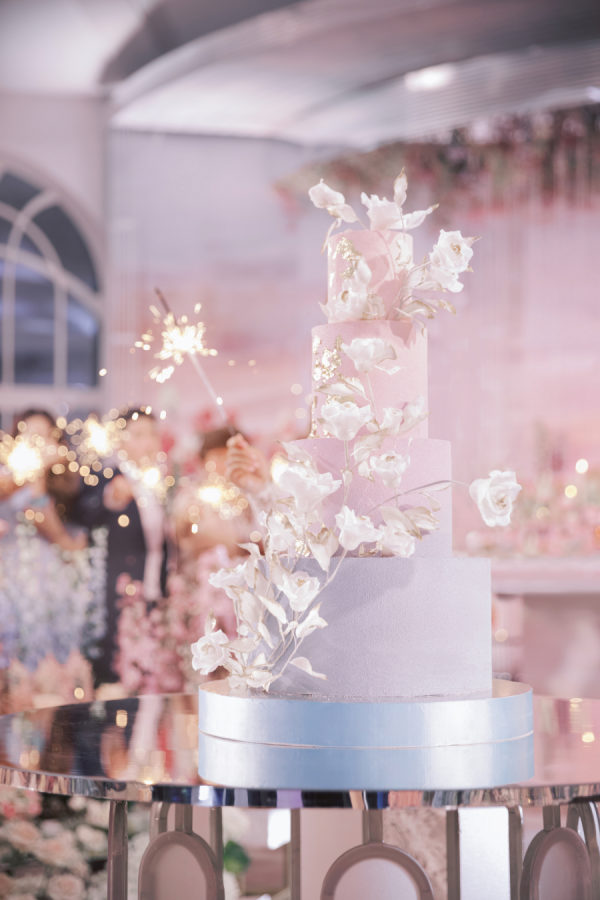
[iframe https://player.vimeo.com/video/384992271 600 338]
Share this gorgeous gallery on |
Photography: Julia Kaptelova Photography | Wedding Planner: Caramel | Cake: Any Cake | Invitations: Inviteria | Rings: Harry Winston | Band: Menhouzen | Grooms attire: Ermenegildo Zegna | Wedding Venue: Elizaveta Panichkina | Bridesmaids’ dresses: Marchesa | Bridesmaids’ dresses: Alice McCall | Bride’s gown : Jaton Couture | Bride’s shoes: Manolo Blahnik | Decor : About you decor | Earrings: Damiani | Muah: Khvanaco Studio | Video: Artem Korchagin
More Princess-Worthy Ballgowns

I’m still not convinced this Moscow wedding, captured to perfection by Sonya Khegay , isn’t actually an inspiration session—it’s just that breathtaking. From the beautiful Bride’s gorgeous lace wedding dress and flawless hair and makeup to the pretty pastel color palette and stunning ceremony and reception spaces, this wedding is almost too good to be true. Do yourself a favor and see it all in The Vault now!
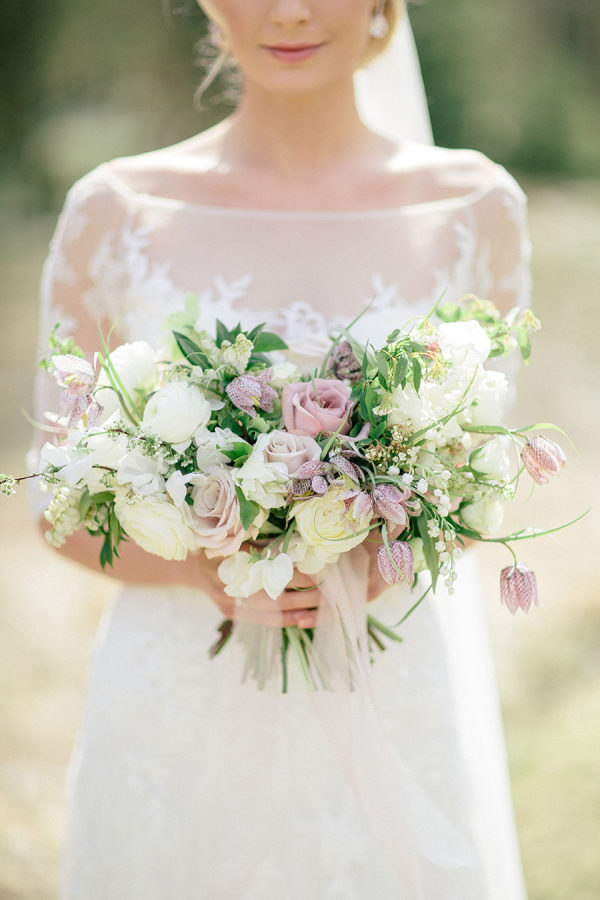
From Sonya Khegay … It was the last day of April and still very cold in the morning. The weather forecast wasn’t pleasing and no one expected that the sun would come out, but miracles happen and light rain gave way to the warm rays.
I love how all the details went together, you could feel the harmony in everything throughout the entire wedding day from the morning until the fireworks.
A gentle look of the bride, elegant but so airy and unique decor, the fresh and light atmosphere of early spring and, of course, true happiness in the eyes. My heart becomes so warm from these memories, it is always a pleasure to see the birth of a new family of two loving hearts.
Photography: Sonya Khegay | Event Design: Latte Decor | Event Planning: Ajur Wedding | Floral Design: Blush Petals | Wedding Dress: La Sposa | Stationery: Special Invite | Bride's Shoes: Gianvito Rossi | Hair + Makeup: Natalie Yastrebova | Venue: Rodniki Hotel
- by Elizabeth Greene
You really can’t go wrong with simple: a beautiful Bride , perfectly pretty petals , loved ones all around. But add in an amazing firework show to cap off the night and simple just became downright extraordinary. Captured by Lena Elisseva , with assistance by Katya Butenko , this rustic Russian celebration is simply fantastic. See it all in the Vault right here !
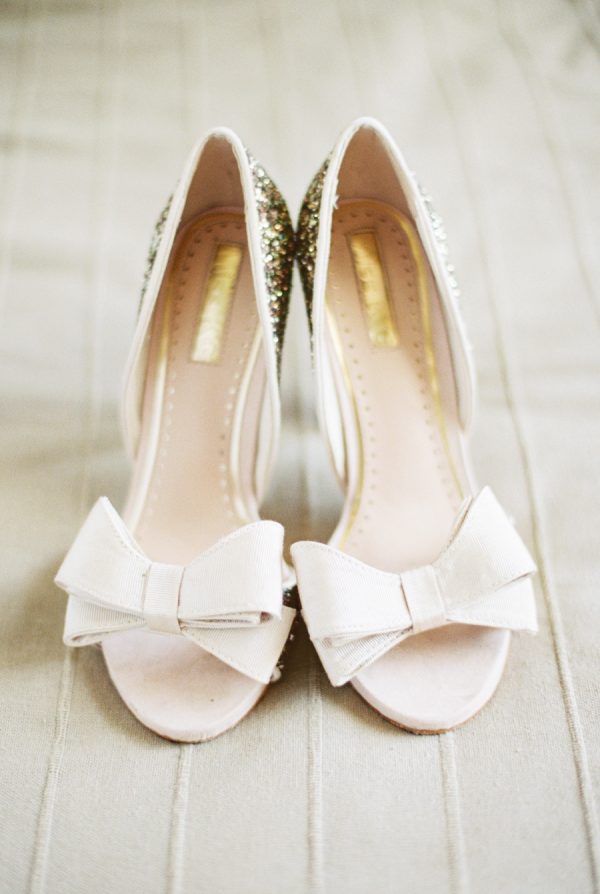
From Lena Eliseeva Photo … This cozy and warm summer wedding of gorgeous Natalia and Anton was in the middle of June. The young couple decided to organize their wedding themselves, and the day was very personal and touching. I am absolutely in love with rustic outdoor weddings, and this one is my favourite because of the free and easy atmosphere.
All the decor excluding the bride’s bouquet was made by a team of ten friends of the bride and groom. And it was charming – a light and beautiful arch, eco-style polygraphy and succulents, candy-bar with caramel apples and berries – sweet joys of summer.
At the end of ceremony the guests tossed up white handkerchiefs embroidered by Natalia’s own hands.
The most touching moment was the happy eyes of the groom’s grandmother, the most estimable person on the wedding. And the fireworks were a bright end to that beautiful day.

Photography: LENA ELISEEVA PHOTO | Floral Design: Katerina Kazakova | Hair And Makeup: Svetlana Fischeva | Photography - Assistance: Katya Butenko
These photos from Lena Kozhina are so stunningly beautiful – as in you can’t help but stop and stare – it’s hard to believe it’s real life. But these pics are proof of this gorgeous Bride and her handsome Groom’s celebration at Moscow’s Fox Lodge , surrounded by vibrant colors and breathtaking blooms . Oh, and the idea of prepping for your Big Day outside in the sun ? Brilliant. See more bright ideas right here !
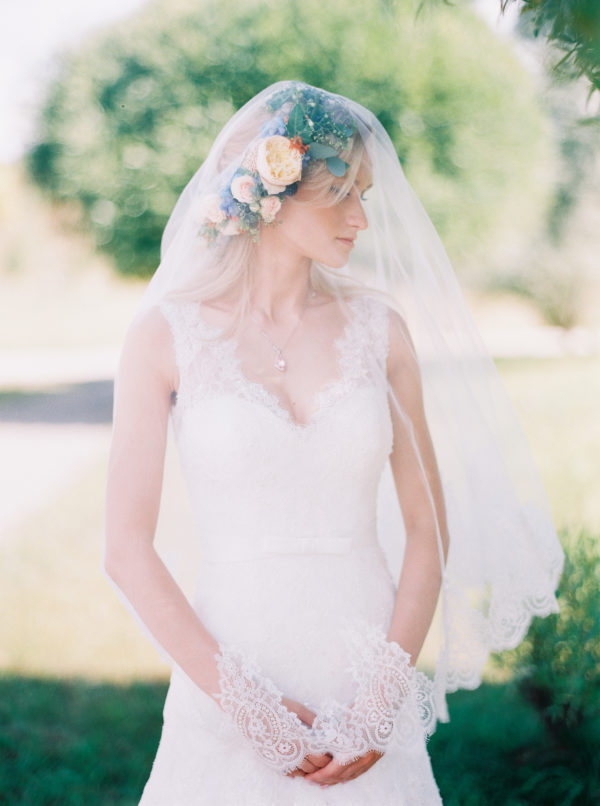
From Lena Kozhina … When we met with the couple for the first time, we immediately paid attention to Dima’s behavior towards Julia. There was a feeling of tenderness and awe, and we immediately wanted to recreate this atmosphere of love, care and warmth on their Big Day.
Later, when we had chosen a green meadow and an uncovered pavilion overlooking a lake as the project site, it only highlighted a light summer mood with colorful florals and a great number of natural woods. The name of the site is Fox Lodge and peach-orange color, as one of the Bride’s favorites, set the tone for the whole design – from the invitations, in which we used images of fox cubs to elements of serving guest tables and other decorative elements with the corresponding bright accents.
Photography: Lena Kozhina | Event Planning: Ajur Wedding | Wedding Dress: Rosa Clara | Shoes: Marc Jacobs | Catering: Fox Lodge | Makeup Artist: Elena Otrembskaya | Wedding Venue: Fox Lodge | Cake and Desserts: Yumbaker | Decor: Latte Decor
From Our Partners

You are using an unsupported browser ×
You are using an unsupported browser. This web site is designed for the current versions of Microsoft Edge, Google Chrome, Mozilla Firefox, or Safari.
Site Feedback
The Office of the Federal Register publishes documents on behalf of Federal agencies but does not have any authority over their programs. We recommend you directly contact the agency associated with the content in question.
If you have comments or suggestions on how to improve the www.ecfr.gov website or have questions about using www.ecfr.gov, please choose the 'Website Feedback' button below.
If you would like to comment on the current content, please use the 'Content Feedback' button below for instructions on contacting the issuing agency
Website Feedback
- Incorporation by Reference
- Recent Updates
- Recent Changes
- Corrections
- Reader Aids Home
- Using the eCFR Point-in-Time System
- Understanding the eCFR
- Government Policy and OFR Procedures
- Developer Resources
- My Subscriptions
- Sign In / Sign Up
Hi, Sign Out
The Electronic Code of Federal Regulations
Enhanced content :: cross reference.
Enhanced content is provided to the user to provide additional context.
Navigate by entering citations or phrases (eg: suggestions#fillExample" class="example badge badge-info">1 CFR 1.1 suggestions#fillExample" class="example badge badge-info">49 CFR 172.101 suggestions#fillExample" class="example badge badge-info">Organization and Purpose suggestions#fillExample" class="example badge badge-info">1/1.1 suggestions#fillExample" class="example badge badge-info">Regulation Y suggestions#fillExample" class="example badge badge-info">FAR ).
Choosing an item from citations and headings will bring you directly to the content. Choosing an item from full text search results will bring you to those results. Pressing enter in the search box will also bring you to search results.
Background and more details are available in the Search & Navigation guide.
- Title 43 —Public Lands: Interior
- Subtitle B —Regulations Relating to Public Lands
- Chapter II —Bureau of Land Management, Department of the Interior
- Subchapter C —Minerals Management (3000)
- Part 3100 —Oil and Gas Leasing
- Subpart 3107 —Continuation and Extension
Extension of Leases Segregated by Assignment
Enhanced content - table of contents.
IMAGES
VIDEO
COMMENTS
Performance of these assignments can then be evaluated for each element or group individually ti. a performance score can be computed for each element or group of elements. The question: What optimization algorithm would you recommend for efficiently solving (maximizing the sum of scores of individual elements) this problem with very large ...
7 Mar 2021. It is fairly common in a classroom setting to have students who must be assiged to projects, either to work alone or in groups. If the students have each ranked the projects from most to least desirable, one typically wants to assign all of the students to their most desired projects. This is a perfect scenario for optimization, in ...
The assignment problem is a fundamental combinatorial optimization problem. In its most general form, the problem is as follows: The problem instance has a number of agents and a number of tasks. Any agent can be assigned to perform any task, incurring some cost that may vary depending on the agent-task assignment.
One of the most well-known combinatorial optimization problems is the assignment problem. Here's an example: suppose a group of workers needs to perform a set of tasks, and for each worker and task, there is a cost for assigning the worker to the task. The problem is to assign each worker to at most one task, with no two workers performing the ...
CS 506 Group Assignment Optimization. I specialized my goal to improve CS 506 project assignment experience. The objective is to maximize the student happiness and group fairness. Through visualization, I gained many insights into the optimal solution and the domain problem.
All users will be members of exactly 2 groups. Each group must have between 4 and 6 members. The users have ordered their preferences for group membership (i.e., users have listed in order the groups they would like to be apart of). I would like a solution that assigns all users to groups that minimizes the total distance of all users from ...
In §§3 and 4, we describe our constraint pro-gramming model for assigning students to groups. Section 5 presents computational results from apply-ing our model (with various objective functions) to seven test problems. Section 6 concludes the paper and gives directions for future work. 2.
Group role assignment with a flexible formation (GRAFF) is essential for group performance optimization in collaborative systems. In this paper, problems of GRAFF are formalized based on the Environment-Class, Agent, Role, Group, and Object (E-CARGO) model. Then, based on group role assignment (GRA) and linear programming (LP), two algorithms are proposed. Experiments are developed to analyze ...
• We develop a heuristic optimization pipeline in GO4Align to tractably achieve the principle, involving dynamical group assignment and risk-guided group indicators. The pipeline incor-porates beneficial task interactions into the group assignments and exploits task correlations for multi-task alignment, improving the overall multi-task ...
The company plans to divide the participants into 10 groups, each of 6 trainees. Each of the trainees was asked beforehand to choose 5 other trainees they would like to work with. And each of the five is weighted equally. The problem is how we can assign the participants into groups so that the total satisfaction could to optimised. optimization.
Table 3 summarizes the optimization results for 16 courses, including nine for which the group assignment was done manually and the preference sheets were still available, and two where primarily the computer tool was employed, but a manual assignment was created additionally by some lecturers (not knowing the results of the computer tool). All ...
This section presents an example that shows how to solve an assignment problem using both the MIP solver and the CP-SAT solver. Example. In the example there are five workers (numbered 0-4) and four tasks (numbered 0-3). Note that there is one more worker than in the example in the Overview.
2. Assume that there are n students, who have to be evenly assigned to m groups. For every student, a preference ranking of of the m groups is given. I partially order assignment by pointwise preference, i.e. one is better or equal to another if for every student, the assigned group is ranked higher or equal.
Optimization Group Project Instructions Instructions. This is a group assignment. In order to complete the assignment, first read the "Hawley Lighting Company" case study. Conduct necessary calculations and answer the questions listed below. Submit your Excel spreadsheet(s) with calculations to the assignment dropbox before the posted deadline.
This paper proposes \\textit{GO4Align}, a multi-task optimization approach that tackles task imbalance by explicitly aligning the optimization across tasks. To achieve this, we design an adaptive group risk minimization strategy, compromising two crucial techniques in implementation: (i) dynamical group assignment, which clusters similar tasks based on task interactions; (ii) risk-guided group ...
1: Conflict Resolution Strategies. Conflicts among team members can disrupt the harmony essential for successful group assignments. Excel can be a valuable tool for tracking and addressing these conflicts. By creating a log within Excel, you can document conflicts, their resolutions, and any ongoing issues.
Group formation for solving collaborative tasks The optimization goals for task assignment is putatively similar between collaborative task and traditional microtask—maximize the quality of the completed tasks while minimizing cost by assigning appropriate tasks to appropriate workers. Task assignment has been extensively studied for ...
Those in the Fleet Optimization logical group can be used by multiple processes. For the Optimize Driver Assignments action, use the parameters under the 'General' and 'Savings Merge Algorithm' groups to define values used by the savings merge algorithm when Savings Merge is selected for the Fleet Assignment Algorithm parameter. If 'Column ...
Group formation for solving collaborative tasks The optimization goals for task assignment is putatively simi-lar between collaborative task and traditional microtask— maximize the quality of the completed tasks while mini-mizing cost by assigning appropriate tasks to appropriate workers.
Service provider assignment can use commitments to assign service providers to shipments on legs that share equipment with other legs. Note the following: When order movements on the other shared equipment legs are not already planned, then OTM will be able to choose equipment group as well as service provider.
From the wedding planner, Ajur Wedding… This real wedding is pure inspiration. With each stroke of the brush, the details add to the already ideal picture: one of the most picturesque Moscow areas, the warm day in June, the tenderest and the most beautiful bridal portraits, the ceremony in the greenhouse, wallowing in flowers and sun rays…
§ 3107.60 Extension of reinstated leases. Where a reinstatement of a terminated lease is granted under 43 CFR 3108.20 and the authorized officer finds that the reinstatement will not afford the lessee a reasonable opportunity to continue operations under the lease, the authorized officer may extend the term of such lease for a period sufficient to give the lessee such an opportunity.
Geographic coordinates of Elektrostal, Moscow Oblast, Russia in WGS 84 coordinate system which is a standard in cartography, geodesy, and navigation, including Global Positioning System (GPS). Latitude of Elektrostal, longitude of Elektrostal, elevation above sea level of Elektrostal.
In 1938, it was granted town status. [citation needed]Administrative and municipal status. Within the framework of administrative divisions, it is incorporated as Elektrostal City Under Oblast Jurisdiction—an administrative unit with the status equal to that of the districts. As a municipal division, Elektrostal City Under Oblast Jurisdiction is incorporated as Elektrostal Urban Okrug.
Elektrostal, city, Moscow oblast (province), western Russia.It lies 36 miles (58 km) east of Moscow city. The name, meaning "electric steel," derives from the high-quality-steel industry established there soon after the October Revolution in 1917. During World War II, parts of the heavy-machine-building industry were relocated there from Ukraine, and Elektrostal is now a centre for the ...
This paper proposes a meta-heuristic called Eel and Grouper Optimizer (EGO). The EGO algorithm is inspired by the symbiotic interaction and foraging strategy of eels and groupers in marine ecosystems. The algorithm's efficacy is demonstrated through rigorous evaluation using nineteen benchmark functions, showcasing its superior performance compared to established meta-heuristic algorithms ...
Group C 1-1 vs Slovenia (Stuttgart, 16 June) 1-1 vs England (Frankfurt, 20 June) 0-0 vs Serbia (Munich, 25 June) Round of 16 Germany 2-0 Denmark (Dortmund, 29 June) England EURO 2024 results, fixtures